Operators are the mini-commands that make code come alive! They’re like special symbols that tell a computer what actions to perform on values and variables. Think of them as the tools that let you craft calculations, comparisons, and logical decisions within your code. Let’s dive into the world of Solidity Operators!
Here’s a quick breakdown of the key operator types:
- Arithmetic Operators
- Comparison Operators
- Logical Operators
- Assignment Operators
- Bitwise Operators
- Special Operators
Arithmetic Operators
Arithmetic operators are like math helpers in programming that let you do things like add, take away, multiply, and divide numbers.
Operator | Symbol | Description | Example |
---|---|---|---|
Addition | + | Adds two values together | uint256 totalSupply = initialSupply + tokensMinted; |
Subtraction | - | Subtracts one value from another | uint256 remainingTokens = totalSupply - tokensSold; |
Multiplication | * | Multiplies two values | uint256 totalReward = tokenAmount * rewardRate; |
Division | / | Divides one value by another (integer division) | uint256 averagePrice = totalAmount / numberOfSales; |
Modulo | % | Gives the remainder after division | bool isEven = (number % 2) == 0; |
Key Points:
- Integer Division: Solidity’s division only returns the quotient, discarding any remainder. For floating-point division, you’ll need to use external libraries or tricks.
- Overflow/Underflow: Be cautious of potential overflow or underflow when dealing with large numbers or calculations. Solidity doesn’t throw exceptions for these cases, so handle them appropriately to ensure contract integrity.
- Precedence: Remember the order of operations (PEMDAS/BODMAS) applies in Solidity. Use parentheses to clarify the order of calculations if needed.
Comparison Operators
Comparison operators in Solidity act as truth-seeking detectives, evaluating relationships between values and returning boolean results (true or false). They’re essential for crafting conditional logic and ensuring contract integrity.
Operator | Symbol | Description | Example |
---|---|---|---|
Equal to | == | Checks if two values are exactly the same | bool isApproved = (sender == owner); |
Not equal to | != | Verifies if two values are not the same | require(tokenId != 0, "Invalid token ID"); |
Greater than | > | Determines if the first value is larger than the second | if (bidAmount > highestBid) { highestBid = bidAmount; } |
Less than | < | Checks if the first value is smaller than the second | require(balance >= amount, "Insufficient funds"); |
Greater than or equal to | >= | Assesses if the first value is greater than or equal to the second | if (playerLevel >= requiredLevel) { accessGranted = true; } |
Less than or equal to | <= | Determines if the first value is less than or equal to the second | while (index <= arrayLength) { processElement(array[index]); } |
Key Points:
- Data Type Compatibility: Ensure the values being compared are of compatible data types. Comparing incompatible types can lead to unexpected results.
- Strict Equality: Solidity uses strict equality checks for values. For example,
0
is not considered equal tofalse
. - Address Comparison: Use the
==
operator to compare addresses directly.
Logical Operators
Logical operators in Solidity are the master weavers of complex decisions, allowing you to craft intricate conditional logic within your smart contracts. They act like Boolean gatekeepers, evaluating conditions and determining which code paths to execute.
Operator | Symbol | Description | Example |
---|---|---|---|
AND | && | Returns true only if both operands are true | if (isAdmin && isVerified) { approveTransaction(); } |
OR | || | Returns true if at least one operand is true | if (hasPermission(Permission.READ) || isOwner()) { allowAccess(); } |
NOT | ! | Inverts the truth value of its operand | if (!isLocked) { allowTransfer(); } |
Key Points:
- Operand Types: Logical operators primarily work with Boolean values (
true
orfalse
). However, Solidity can implicitly convert non-zero numbers totrue
and 0 tofalse
in certain contexts. - Short-Circuit Evaluation: Solidity uses short-circuit evaluation for AND and OR operators. This means it only evaluates the second operand if necessary to determine the final result.
- Precedence: Logical operators have lower precedence than comparison operators. Use parentheses to clarify the order of evaluation when combining different operators.
Assignment Operators
Assignment operators in Solidity are the diligent scribes of your smart contracts, gracefully assigning values to variables and ensuring the flow of information within your code. They act like bridges between calculations and storage, keeping track of important data and enabling dynamic updates.
Operator | Symbol | Description | Example |
---|---|---|---|
Simple Assignment | = | Assigns the value on the right to the variable on the left | uint256 balance = 100; |
Compound Assignment (Addition) | += | Adds the value on the right to the variable and assigns the result | balance += 50; |
Compound Assignment (Subtraction) | -= | Subtracts the value on the right from the variable and assigns the result | balance -= 2; |
Compound Assignment (Multiplication) | *= | Multiplies the variable by the value on the right and assigns the result | price *= 1.15; |
Compound Assignment (Bitwise AND) | &= | Performs a bitwise AND operation between the variable and the value on the right, and assigns the result | flag &= 0x0F; |
Key Points:
- Evaluation Order: The expression on the right side is evaluated first, then the result is assigned to the variable on the left.
- Return Value: Assignment operators don’t return a value, so they can’t be used directly within expressions.
- Data Type Compatibility: Ensure the value being assigned is compatible with the variable’s data type to avoid errors.
Bitwise Operators
Bitwise operators in Solidity offer a granular way to manipulate individual bits within binary data, empowering you to perform precise operations and optimizations within your smart contracts. They act like skilled micro-technicians, working directly with the fundamental building blocks of binary information.
Operator | Symbol | Description | Example |
---|---|---|---|
Bitwise AND | & | Compares corresponding bits of two values and sets the resulting bit to 1 only if both bits are 1. | uint8 flags = 0b1010; uint8 mask = 0b0101; uint8 result = flags & mask; // result will be 0b0000 |
Bitwise OR | | | Sets a resulting bit to 1 if either or both corresponding bits in the operands are 1. | uint8 a = 0b1100; uint8 b = 0b0011; uint8 result = a | b; // result will be 0b1111 |
Bitwise XOR | ^ | Sets a resulting bit to 1 if the corresponding bits in the operands are different. | uint8 a = 0b1010; uint8 b = 0b1100; uint8 result = a ^ b; // result will be 0b0110 |
Bitwise NOT | ~ | Inverts the bits of a single operand, flipping 1s to 0s and 0s to 1s. | uint8 x = 0b1111; uint8 result = ~x; // result will be 0b0000 |
Bitwise Left Shift | << | Shifts the bits of a value to the left by a specified number of positions, adding zeros to the right. | uint8 x = 0b0010; uint8 result = x << 2; // result will be 0b1000 |
Bitwise Right Shift | >> | Shifts the bits of a value to the right by a specified number of positions, discarding bits on the right. | uint8 x = 0b1000; uint8 result = x >> 1; // result will be 0b0100 |
Special Operators
While Solidity shares many common operators with other programming languages, it also features a few unique operators that cater to its specific needs in the blockchain environment. Here’s a rundown of some notable special operators.
Operator | Description | Example |
---|---|---|
. | Member Access | user.balance += amount; |
[] | Index Access | uint256 firstNumber = numbers[0]; |
... | Unpacking and array copying | (bool success, bytes memory result) = target.call(data); |
is | Inheritance check | require(tokenContract is ERC20, "Not an ERC20 token"); |
as | Type casting | address payable recipient = payable(msg.sender); |
! | Boolean negation | if (!isOwner) { revert("Unauthorized access"); } |
++ , -- | Increment/decrement (with specific Solidity behavior) | x++ |
? : | Conditional (ternary) operator | age = isAdult ? 25 : 17; |
** (experimental) | Exponentiation (Solidity 0.8.0+) | a ** b |
% (experimental) | Remainder for signed integers (Solidity 0.8.0+) | a % b |
Conclusion: Solidity Operators
Solidity operators, which seemed like just confusing symbols at first, are actually like magic in your smart contracts. They turn simple data into smart decisions and control. Think of operators not just as tools, but as your way to paint your ideas into the blockchain world.
The learning doesn’t stop here, though. The Solidity documentation is full of more things to learn. You can also join other people who are building cool things, try out your own ideas, and become really good at using operators. When you write code, you’re making a new world where smart contracts make things easier and more interesting.
So, get ready, programmers! Use these solidity operators smartly and have fun with them. Make things that nobody has thought of before. The blockchain is waiting for your ideas, and people can’t wait to see what you create. Keep learning and building!
Want to learn more on Solidity, check out our recent articles on solidity variables, global variables, methods and control structures.
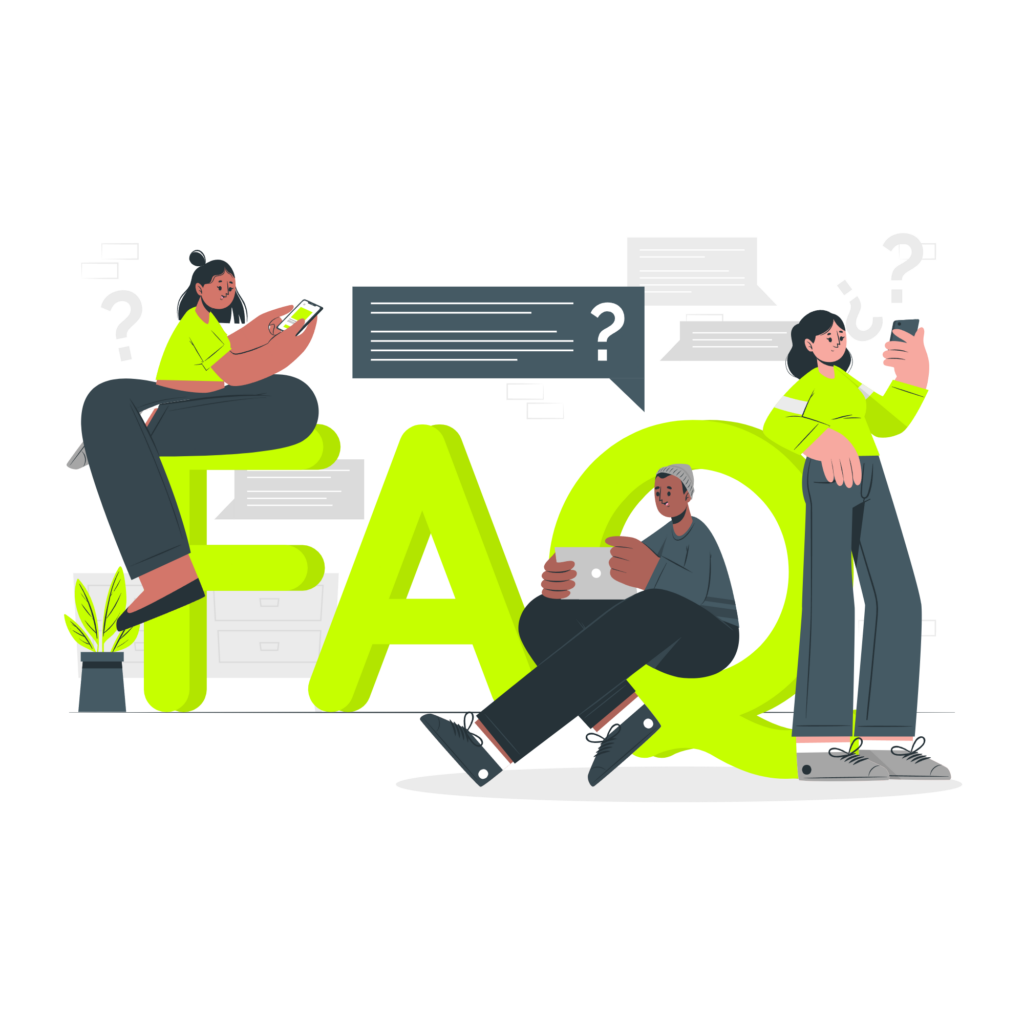
What are Solidity operators in Ethereum programming?
- Solidity operators are special symbols used in programming that perform operations on variables and values. They include arithmetic operators (like
+
,-
), comparison operators (like==
,!=
), logical operators (like&&
,||
), and others, crucial for controlling the logic and flow in Ethereum smart contracts.
How do comparison operators work in Solidity?
- Comparison operators in Solidity, such as
==
,!=
,>
,<
,>=
, and<=
, are used to compare values. They are essential in making decisions within smart contracts, like validating conditions before transactions.
Why are arithmetic operators important in Solidity?
- Arithmetic operators in Solidity are used for basic mathematical operations which are essential in handling and manipulating numerical values in smart contracts, such as calculating token distributions or user balances.
Can you use logical operators in Solidity for multiple conditions?
- Yes, logical operators like AND (
&&
), OR (||
), and NOT (!
) in Solidity are used to combine multiple conditions, which is vital for complex decision-making in smart contracts.
What is the significance of the assignment operators in Solidity?
- Assignment operators in Solidity, like
=
,+=
,-=
, are used for assigning and updating the values of variables. They are fundamental in changing the state of smart contracts.
What is gas optimization in Solidity?
- Gas optimization in Solidity involves writing code in a way that minimizes the computational resources required to execute transactions, thus reducing the cost (or “gas”) on the Ethereum network.
How does the Ethereum Virtual Machine (EVM) work?
- The EVM is the runtime environment for smart contracts in Ethereum. It’s like a global computer where all Ethereum smart contracts are executed and where state changes are stored.
What are the security considerations when writing smart contracts?
- Security in smart contract development includes guarding against common vulnerabilities, such as reentrancy attacks, overflow/underflow, and ensuring proper access control and error handling.
Can Solidity be used for creating NFTs?
- Yes, Solidity is commonly used for creating NFTs (Non-Fungible Tokens) on the Ethereum blockchain, as it can define unique properties and ownership rules.
What’s the future of blockchain technology in terms of development?
- The future of blockchain development is likely to see increased adoption in various sectors, improvements in scalability and sustainability, and advancements in interoperability among different blockchain networks.