A deeper understanding on the difference between storage, memory, and calldata is important for optimizing smart contracts, ensuring better performance, reducing gas costs, and securing data integrity. Solidity, the primary language for Ethereum smart contract development, provides these three data locations for variables, each serving distinct purposes and operating within different scopes of the Ethereum Virtual Machine.
This article dives into the functionality of each component, their specific use cases, and how they can be effectively utilized for efficient Solidity development.
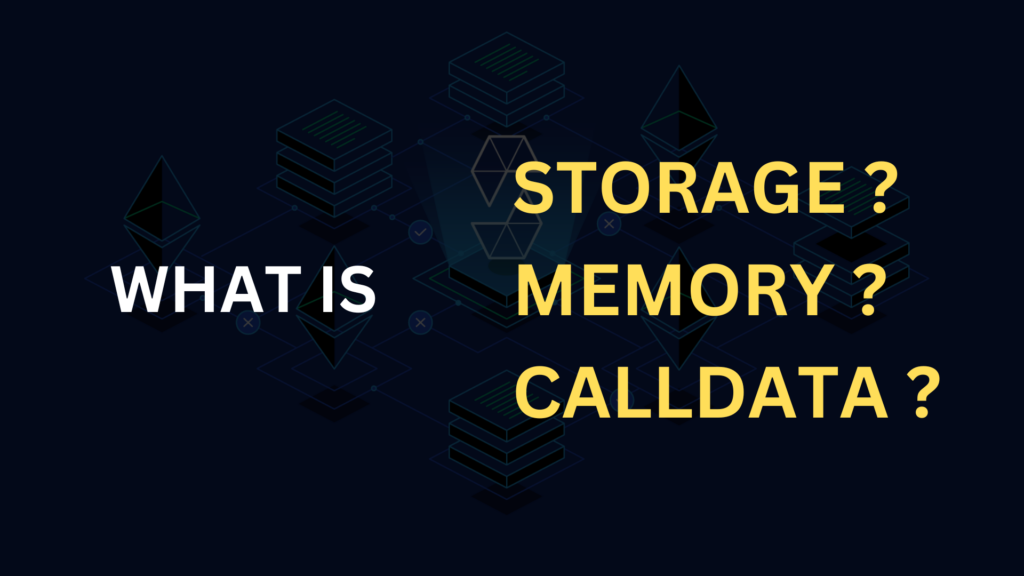
Variables in Solidity
Solidity supports several types of variables that interact with Ethereum’s blockchain in different ways. These variables are categorized based on their scope, lifetime, and location in memory, which allows developers to manage data efficiently during smart contract execution.
State variables are variables whose values are stored permanently in contract storage, meaning they maintain their values between function calls and across transactions. They are declared at the contract level, outside of functions, and form the “state” of the smart contract. Since Ethereum is a decentralized platform, any data that is stored in state variables is written onto the blockchain, making it accessible, immutable, and auditable by anyone interacting with the contract.
When you define a variable in Solidity, the data needs to be stored somewhere during the execution. The location where this data is stored is crucial for both gas efficiency and the behavior of the contract. Solidity offers three main types of data locations for handling variables in smart contracts.
- Storage
- For persistent, on-chain data that remains between transactions.
- Memory
- For temporary data used within function calls that do not persist after execution.
- Calldata
- For read-only, external inputs passed to functions from users or other contracts.
Understanding how each data location works and when to use them effectively is important to help you write robust and optimized smart contracts in Solidity.
Storage in Solidity
Storage refers to data that is persistently maintained on the Ethereum blockchain. These are known as solidity state variables, which remain intact across function calls and transactions. Due to their permanent storage on the blockchain, state variables are costly in terms of gas consumption. Variables declared at the contract level (state variables) are automatically stored in storage.
pragma solidity ^0.8.0;
contract StorageExample {
uint public storedData; // state variable
function setStoredData(uint _data) public {
storedData = _data; // storedData is stored in storage
}
}
In this example, storedData
is stored in storage and persists across function calls, even after the transaction is complete.
Memory in Solidity
Memory in Solidity serves as a temporary storage location, holding data only for the duration of a function call. Once the function execution is complete, the data in memory is discarded. Variables stored in memory are less costly in terms of gas compared to storage but more expensive than calldata. Unlike state variables, memory variables are transient and used only within the scope of a function.
pragma solidity ^0.8.0;
contract MemoryExample {
function manipulateData(uint _data) public pure returns (uint) {
uint tempData = _data + 10; // tempData is stored in memory
return tempData;
}
}
In this example, tempData
is stored in memory and is discarded once the function completes.
Calldata in Solidity
Calldata is a temporary, non-modifiable storage location where function arguments are stored. This data is passed to a function by external callers and can only be read but not modified. Calldata is generally cheaper than memory because it doesn’t allow modification, making it the most efficient for function inputs.
pragma solidity ^0.8.0;
contract CalldataExample {
function processArray(uint[] calldata _data) public pure returns (uint) {
return _data[0] + _data[1]; // _data is stored in calldata and is read-only
}
}
In this example, _data
is passed as calldata, meaning it cannot be modified within the function.
Storage vs. Memory vs. Calldata – What are the Differences ?
Now that we know what these concepts are and how they are used in Solidity development, let’s explore on what are the key differences between them.
Feature | Storage | Memory | Calldata |
---|---|---|---|
Persistence | Persistent across function calls and transactions. | Temporary during execution, discarded after the function. | Temporary during execution, discarded after the function. |
Gas Cost | Most expensive since data is written to the blockchain. | Moderately expensive, cheaper than storage but costlier than calldata. | Least expensive in terms of gas usage. |
Modifiability | Data can be modified during contract execution. | Data is modifiable while the function is running. | Immutable, read-only during function execution. |
Usage | Used for state variables that need to persist across transactions and function calls. | Ideal for temporary variables used within a function’s scope. | Typically used for function parameters in external calls. |
Choosing the Right Storage Class
The selection of a storage class for a state variable depends on its intended purpose and the desired behavior within the smart contract. Choosing the appropriate storage class ensures that variables are used efficiently, balancing factors like persistence, gas costs, and access requirements to optimize the contract’s performance.
Following are some key guidelines to consider when deciding which storage class to use:
- Persistent Data
- Use
storage
for data that needs to be retained and accessible throughout the contract’s lifetime.
- Use
- Temporary Data
- Use
memory
for data that is only needed within a function’s scope.
- Use
- Function Arguments
- Use
calldata
for function arguments.
- Use
Best Practices
Optimizing gas costs is an important aspect of developing efficient and cost-effective smart contracts in Solidity. Since gas fees are directly tied to the computational resources consumed by transactions, it’s essential to adopt strategies that reduce unnecessary gas usage.
By understanding how storage, memory, and calldata work, and applying best practices, developers can significantly lower the gas consumption of their contracts. Below are some effective techniques for optimizing gas costs when handling data in Solidity.
- Minimize Use of Storage
- Since storage operations are expensive, avoid unnecessary storage writes. If possible, store data in memory for temporary operations.
- Use Calldata for External Inputs
- For functions that take arguments and don’t modify them, use
calldata
instead ofmemory
to reduce gas costs.
- For functions that take arguments and don’t modify them, use
- Pack Variables
- When storing multiple variables in a struct or state, ensure their data types are packed efficiently to minimize storage space.
- Avoid Redundant Storage Reads
- Instead of reading from storage repeatedly, store the value in a memory variable if it needs to be accessed multiple times within a function.
Optimize Gas by Reducing Storage Writes
Continuously writing to storage results in high gas costs. By eliminating unnecessary storage writes and minimizing the number of operations that modify storage, you can significantly optimize gas usage for better efficiency.
Refer to the example code below to understand how gas optimization can be achieved by minimizing storage writes.
pragma solidity ^0.8.0;
contract GasOptimization {
uint public count; // state variable
function increment(uint _times) public {
for (uint i = 0; i < _times; i++) {
count++; // Writing to storage multiple times is costly
}
}
}
In this example, count
is updated multiple times, leading to high gas costs. Instead, you can optimize by using a memory variable. Refer to the code below for a better solution.
pragma solidity ^0.8.0;
contract GasOptimization {
uint public count; // state variable
function increment(uint _times) public {
uint tempCount = count; // Read storage variable into memory
for (uint i = 0; i < _times; i++) {
tempCount++;
}
count = tempCount; // Write once to storage
}
}
Here, we minimize storage writes by using a temporary memory variable, tempCount
, which reduces gas costs.
Use Calldata for Immutable Inputs
When a function accepts a parameter but doesn’t modify it, you can simply use calldata to store the value which could significantly reduce gas costs instead of using memory or storage which are comparatively more expensive.
Refer to the example code below to understand how gas optimization can be achieved by using calldata for immutable inputs.
pragma solidity ^0.8.0;
contract CalldataOptimization {
function sum(uint[] calldata _numbers) external pure returns (uint) {
uint total = 0;
for (uint i = 0; i < _numbers.length; i++) {
total += _numbers[i];
}
return total;
}
}
In this example, we use calldata for the input _numbers
array since it is only read and not modified, which leads to a better gas optimization.
Things to Keep in Mind
Following are some key tips to consider when working with Storage, Memory, and Calldata in Solidity to develop robust smart contracts that are fully optimized for gas efficiency.
- Unnecessary Storage Reads/Writes
- Repeatedly accessing storage within loops or functions can result in higher gas costs. Always aim to reduce storage operations.
- Using Calldata Instead of Memory
- If you are passing data to a function and do not need to modify it, always use calldata instead of memory to save gas.
- Not Packing Structs or Arrays
- Failing to pack data efficiently in storage can lead to inefficient use of space, which increases gas costs.
Conclusion: Solidity State Variable
Understanding the differences between storage, memory, and calldata is essential for writing optimized, cost-effective Solidity smart contracts. Efficient use of these data locations can significantly reduce gas consumption and improve the overall performance of your smart contracts. By applying best practices such as reducing storage operations, using calldata where appropriate, and being mindful of gas costs, you can write more efficient and secure Solidity code. Whether you’re a beginner or an experienced professional, mastering these concepts is key to advancing your career in Solidity development.
FAQs
Why is storage more expensive than memory or calldata?
- Storage involves writing data to the Ethereum blockchain, which requires more computational resources and results in higher gas fees. Data in storage persists across transactions, making it more expensive compared to memory (temporary) and calldata (read-only).
When should I use memory instead of storage in Solidity?
- Use memory for temporary data that only needs to exist during a single function execution. It’s ideal for local variables and for manipulating data passed to a function when you do not need the changes to persist outside the function call. Always prefer memory when persistence is not required to save on gas costs.
What is the default data location for function parameters in Solidity?
- For complex data types like arrays and structs, the default data location for function parameters is memory. However, for external functions, you can specify calldata as a more efficient, read-only alternative.
Can I return calldata variables from a function?
- No, calldata variables cannot be returned directly from a function because they are only valid within the scope of the function and cannot be passed outside of it. Instead, you can return memory variables or primitive types.
What is the maximum size limit for calldata in Solidity?
- Calldata size is limited by the block size, which means that extremely large calldata inputs could result in failed transactions if they exceed the block gas limit. This is something to consider when passing large arrays or strings as function arguments.