As blockchain continues to grow, the development of smart contracts has become an important aspect of creating decentralized applications. With smart contracts becoming more popular among people and businesses, it’s important to make sure a contract works as expected before it’s deployed on the blockchain. Testing of smart contracts across all functionalities is essential for developers to verify that everything operates correctly and that the contract is free from vulnerabilities.
Testing is a critical step in the smart contract development lifecycle. It involves verifying that the contract’s functions work correctly, handling edge cases, and identifying potential security issues before deployment. Proper testing not only prevents costly errors and exploits but also ensures that your contract behaves as expected under various conditions.
This article delves deep into the concept of testing and will guide you through the process of testing your first smart contract. Whether you’re new to smart contracts or looking to brush up your testing skills, this step-by-step guide will provide you with the foundational knowledge needed to ensure the reliability and security of your smart contracts.
Understanding Smart Contract Testing
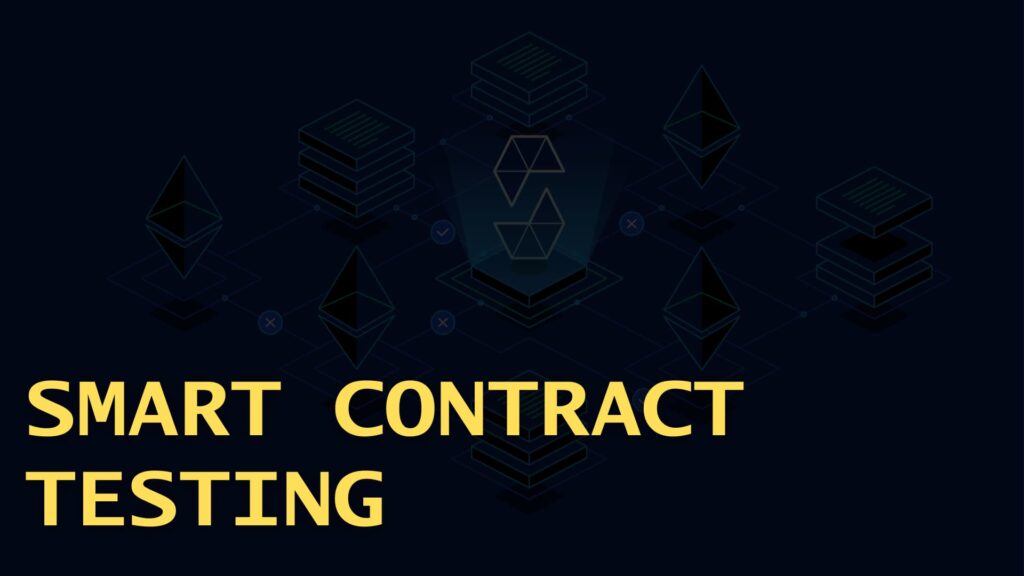
Before we get started with all the testing, it is important to have a clear understanding on why testing is important for smart contracts. Unlike other softwares, smart contracts operate on a public blockchain making them immutable after being deployed. Failing to test a smart contract before deployment could lead to a lot of serious issues such as financial loss or security breaches. Testing would help us identify such issues early during the development stage reducing costly errors that could occur after deployment.
Conducting a test on a smart contract is a lot complexed than how it sounds. A simple test will not ensure that all functionalities of your smart contract is working as expected. As a blockchain developer, it is important to completely understand the testing process to ensure a full test coverage of your smart contract including all possible edge cases. A properly written test case will ensure that all functionalities of the smart contract is working correctly.
Setting up your Development Environment
Before you begin testing, it is important to ensure that your development environment is properly configured. First, verify that Node.js is installed on your system and that it is running the latest version. You can do this by executing the following command on your terminal.
node -v
If Node.js is not installed or is outdated, it is important to ensure that you download and install the latest version correctly on your operating system. You can follow the instructions given here to install Node.js successfully.
Install tools and Frameworks
Now lets create a new directory for your project and run the following command to install necessary tools and frameworks.
npm install --save-dev hardhat jest ethereum-waffle chai
The above command installs the following packages to help conduct a successful test on your smart contract
hardhat
– Hardhat is a versatile Ethereum development environment that integrates well with Jest.jest
– A library that will be used for writing and running your tests.ethereum-waffle
&chain
– These libraries work with Jest to provide testing utilities and assertions for smart contracts.
Configure Jest
It is important to ensure that Jest is properly configured to work with your environment. Create a jest.config.js
file in the root of your project and add the following code.
module.exports = {
testEnvironment: 'node',
setupFilesAfterEnv: ['./test/setup.js'],
testMatch: ['**/*.test.js'],
};
Create a setup.js
file in the test
directory and add the following code to configure the testing environment.
const { ethers } = require('hardhat');
const chai = require('chai');
const { solidity } = require('ethereum-waffle');
chai.use(solidity);
Conduct your First Test
Assume you have the following Solidity file within your project directory inside the contracts
folder, and you are preparing to conduct a test on the specified contract.
SimpleStorage.sol
// contracts/SimpleStorage.sol
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract SimpleStorage {
uint public storedData;
function set(uint x) public {
storedData = x;
}
function get() public view returns (uint) {
return storedData;
}
}
The above contract includes a public state variable storedData
and two functions. set
allows updating storedData
with a new value, and get
returns the current value of storedData
. Now, we will write test cases for these functions to ensure they operate correctly, verifying that the contract properly stores and retrieves the integer value as intended
1. Write your First Test Case with Jest
Now that everything is set up correctly, we can go ahead and write our first test with jest. You can start by creating a file named SimpleStorage.test.js
in the test
directory and adding the following code.
const { expect } = require('chai');
const { ethers } = require('hardhat');
describe('SimpleStorageTest', function () {
let SimpleStorage;
let simpleStorage;
beforeEach(async function () {
SimpleStorage = await ethers.getContractFactory('SimpleStorage');
simpleStorage = await SimpleStorage.deploy();
await simpleStorage.deployed();
});
it('should store the value 89', async function () {
await simpleStorage.set(89);
const storedData = await simpleStorage.get();
expect(storedData).to.equal(89);
});
});
The above file contains code to test your SimpleStorage.sol
contract. The describe
block defines a test suite named SimpleStorageTest
for the SimpleStorage
smart contract. Within the beforeEach
hook, the test suite sets up the testing environment by deploying a new instance of the SimpleStorage
contract before each test is run. The it
block contains a test case that checks if the set
function correctly stores the value 89
in the contract. After calling the set
function with the value 89
, the test retrieves the stored value using the get
function and asserts that it equals 89
using Chai’s expect
function. This ensures that the SimpleStorage
contract behaves as expected.
2. Running the Tests and Analyzing the Test Results
With your first test written, you can now go ahead and run your tests using Jest by executing the following command.
npx jest
After running your tests, Jest will output the results, showing which tests passed and which failed. If any test fails, review the test case and the contract code to identify the issue. Modify your code as necessary and rerun the tests. Additionally, If you encounter issues during testing, you can add console.log
statements or use Jest’s debugging features to inspect variables and understand what’s actually going wrong.
Best Practices
Testing is an important phase in smart contract development where you ensure that your code performs as intended and adheres to the highest standards of security and reliability. Adopting best practices in smart contract testing helps reduce risks and ensures that your contracts perform as intended across various scenarios. These practices help you write effective test cases and implement a thorough test coverage. By following these guidelines, developers can catch potential issues early, improve code quality, and build more secure and reliable decentralized applications.
- Write clear and concise tests:
- Using descriptive names and avoiding redundant tests would help you write clear tests eventually resulting in helping you maintain a proper test coverage for your smart contract.
- Test all possible edge cases:
- Performing test on scenarios like zero values, maximum limits, or invalid inputs would ensure a proper test coverage for your smart contract.
- Leverage Code Coverage:
- Measuring the percentage of your codebase that is covered by tests ensure a proper test coverage for you smart contract and enables you to identify all possible vulnerabilities early during development stage.
- Consider security audits:
- Engage professionals to identify potential vulnerabilities in the security and reliability of your smart contract.
Conclusion
As smart contracts gain prominence and blockchain technology continues to evolve, it is important to ensure that contracts are free from vulnerabilities that could affect the trust that has been built. This is why rigorous testing is an essential phase during smart contract development. Conducting tests on smart contracts is a critical step in the development process, ensuring that the contract functions correctly and securely before deployment. There are a lot of tools available to facilitate this process for developers. However, as a blockchain developer, it is important to thoroughly understand these concepts to contribute effectively to the ongoing success and reliability of blockchain technology in today’s world.
FAQs
What are the basic steps to test a smart contract?
- Write test cases, deploy the contract on a testnet, and run tests using tools like Truffle or Hardhat.
Which tools are best for testing smart contracts?
- Truffle, Hardhat, and Remix are popular tools that provide robust frameworks for testing smart contracts.
Why is it important to test a smart contract before deployment?
- Testing ensures your smart contract functions as intended, preventing costly errors and vulnerabilities on the blockchain.
How can I use a testnet for smart contract testing?
- Deploy your contract on a testnet like Ropsten or Rinkeby to simulate real-world blockchain interactions without financial risk.
What are common issues to look for when testing smart contracts?
- Watch for reentrancy attacks, gas efficiency, and boundary conditions to ensure your smart contract is secure and efficient.