In blockchain development, particularly on Ethereum, testing smart contracts is crucial to ensuring that they function as expected and are secure from exploits. Once a smart contract is deployed, it is immutable, which means that any bugs or vulnerabilities can’t be fixed without deploying a new contract , a costly and complex process. Writing thorough unit tests can prevent these problems, but developers face an important decision: Should you write unit tests in Solidity, the language used to develop smart contracts, or JavaScript, a more widely known language used for testing across various platforms?
In this article, we’ll explore the pros and cons of writing unit tests in Solidity versus JavaScript, helping you understand which approach works best for your Ethereum smart contract project.
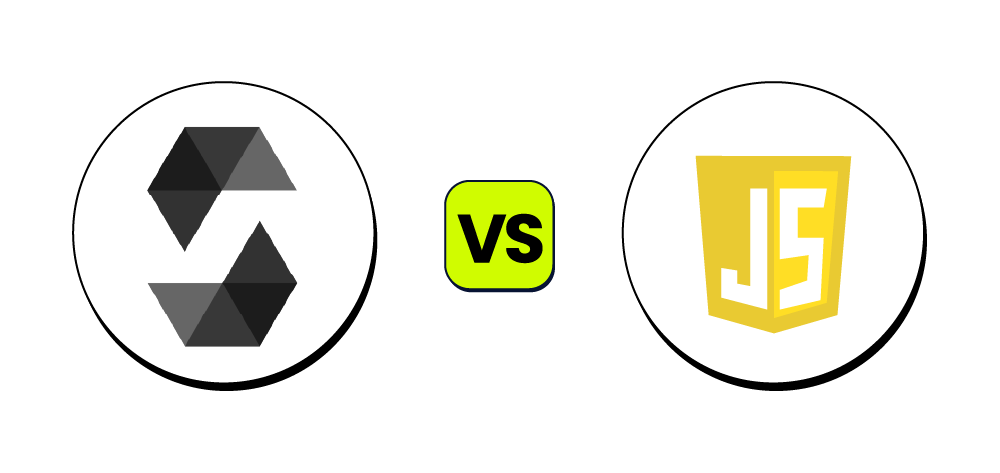
Unit Testing in Blockchain Development
Unit testing involves checking individual units or components of your code to ensure they function as intended. For smart contracts, this means testing specific functions, interactions, and behaviors in a controlled environment before deploying the contract on a live blockchain. Smart contracts operate under very strict rules, and once deployed, they cannot be altered. Therefore, catching issues early through unit testing is essential to avoid costly mistakes, security vulnerabilities, and functional errors.
Ethereum developers typically write smart contracts in Solidity. However, when it comes to testing these contracts, they can use either Solidity itself or JavaScript through testing libraries such as Mocha and Chai.
The Pros of Writing Unit Tests in Solidity
One of the primary benefits of writing unit tests in Solidity is that you are using the native language of Ethereum smart contracts. By writing tests in Solidity, you ensure that the test environment closely mimics the actual Ethereum environment, as the tests are executed on the Ethereum Virtual Machine (EVM) itself. This ensures that the behavior, performance, and execution of your smart contract are as close as possible to how they would perform once deployed.
Solidity offers a higher degree of accuracy and precision in testing. It allows developers to directly test low-level behaviors such as gas usage, memory allocation, and storage costs. This is particularly important for developers working on projects where gas optimization and cost efficiency are critical, as it allows them to simulate real-world Ethereum conditions.
For complex contracts that involve intricate logic, inheritance, or state transitions, Solidity-based unit tests ensure that these aspects are tested thoroughly and correctly. By writing tests in the same language as the contract itself, you reduce the risk of mismatches between the contract’s behavior and the test logic.
Moreover, Solidity is particularly effective for security testing. Common vulnerabilities such as reentrancy attacks, overflow errors, or unauthorized access can be tested directly within the same context in which they would occur, providing better security assurance.
Lastly, writing tests in Solidity means that you’re testing at the EVM level. You can test specific EVM behaviors and edge cases that would be difficult or impossible to replicate accurately with higher-level abstractions such as JavaScript-based frameworks.
The Cons of Writing Unit Tests in Solidity
Despite the advantages, there are several drawbacks to writing unit tests in Solidity. One of the biggest challenges is the learning curve. Solidity is a less familiar and more specialized language compared to JavaScript, making it harder for developers—especially those new to blockchain development—to write efficient and effective tests.
Solidity’s testing frameworks, though functional, are not as mature or user-friendly as the tools available for JavaScript. Libraries like Mocha, Chai, and Jest, commonly used in JavaScript testing, offer a much richer set of features for organizing tests, asserting conditions, and debugging errors. Solidity’s frameworks lack some of these conveniences, which can slow down the testing process.
Another downside is the slower execution of Solidity tests. Since they run on a simulated blockchain environment (such as Ganache) or a local Ethereum network, they are inherently slower than JavaScript-based tests. Developers seeking rapid feedback loops may find this limiting, as each test cycle takes longer.
Additionally, Solidity-based tests can be harder to read and maintain. Solidity’s strict typing and verbosity often result in less readable code compared to JavaScript’s dynamic, flexible syntax. This can make tests harder to manage, particularly for larger projects or teams.
The Pros of Writing Unit Tests in JavaScript
JavaScript is one of the most widely-used programming languages in the world, and many developers are already familiar with its syntax and libraries. Writing unit tests for smart contracts in JavaScript is often easier for most development teams because they already have experience with the language.
JavaScript’s rich ecosystem of testing tools is another key advantage. Testing frameworks like Mocha and Chai provide an array of features that make testing easier, from simple assertions to complex mock setups. Tools like Hardhat and Truffle, which are built specifically for Ethereum development, integrate seamlessly with JavaScript, enabling developers to write and run tests with minimal friction.
Tests written in JavaScript are generally much faster than those written in Solidity. JavaScript-based tests interact with smart contracts using abstractions like Web3.js or Ethers.js, which means the tests don’t need to run directly on the blockchain. This results in significantly faster test execution times and quicker feedback during development.
JavaScript also provides better debugging tools compared to Solidity. With tools like console logs, error stacks, and interactive debugging features available out-of-the-box in JavaScript, diagnosing and fixing issues during testing becomes much easier. This can lead to higher developer productivity and fewer headaches when something goes wrong.
Another important advantage of JavaScript is its integration with other tools. Because it is also used for front-end development, JavaScript testing allows developers to not only test smart contracts but also the interaction between the front-end and the blockchain, making it easier to test the entire decentralized application (dApp) stack in a unified way.
The Cons of Writing Unit Tests in JavaScript
Despite its many strengths, JavaScript also has some drawbacks when it comes to testing smart contracts. One of the main issues is the lack of precision. JavaScript tests do not run directly on the EVM, which means they are a step removed from the actual Ethereum environment. This can lead to discrepancies between what happens in testing and what happens when the contract is deployed.
JavaScript interacts with the blockchain through abstractions, and these abstractions can introduce inconsistencies or hide certain behaviors. For example, JavaScript tests do not allow for the same level of control over gas usage and storage allocation as Solidity tests do. This can lead to issues where a contract appears to work in JavaScript tests but behaves differently in production.
Another limitation is that JavaScript is less suited for certain types of security testing. Although it is possible to test for vulnerabilities like reentrancy attacks using JavaScript, it is not as effective as Solidity in testing security issues at the EVM level. JavaScript cannot fully simulate the nuances of Ethereum’s execution environment, which can leave certain vulnerabilities untested.
Additionally, for contracts with complex logic or inheritance structures, writing tests in JavaScript can become cumbersome. The fact that the tests and the contracts are written in different languages can make it harder to ensure that they are in sync, especially as the codebase grows in complexity.
When to Choose Solidity for Unit Testing
Solidity is the better choice when you need to perform highly precise testing, particularly for performance optimization and security verification. For projects focused on decentralized finance (DeFi), where gas efficiency and security are critical, writing tests in Solidity ensures that you are capturing real-world contract behavior with high accuracy.
Solidity is also ideal for testing complex business logic, where inheritance, state machines, or contract interactions are involved. By writing tests in the same language as the contract, you avoid potential discrepancies and can more easily ensure that the contract behaves as intended.
If your project relies heavily on the intricacies of the Ethereum Virtual Machine, such as custom storage layouts or gas optimizations, Solidity-based tests provide the low-level control needed to verify these aspects.
When to Choose JavaScript for Unit Testing
For projects where speed and rapid development cycles are essential, JavaScript is the better option. JavaScript tests run much faster than Solidity tests, making it easier to iterate on your codebase and get immediate feedback. This is particularly useful during the early stages of development when contracts are still evolving.
JavaScript is also a great choice for projects that require close integration between the front-end and the blockchain. Since JavaScript is widely used for building user interfaces, testing both the contract logic and the front-end interactions together provides a more comprehensive view of how the dApp will perform in production.
For teams with less experience in blockchain development, JavaScript offers a more familiar environment. Its extensive ecosystem of testing tools and resources can help onboard developers quickly and enable them to write effective tests without needing deep expertise in Solidity.
Best Practices for Writing Unit Tests in Solidity and JavaScript
To maximize the effectiveness of your unit tests, it’s important to follow best practices regardless of the language you choose. Write comprehensive tests that cover all possible edge cases, including normal, abnormal, and malicious inputs. Isolate each test to ensure that they do not interfere with each other, which can cause unintended side effects.
In Solidity, consider using mock contracts to simulate complex behaviors. For JavaScript, leverage advanced testing features provided by libraries such as Chai, Mocha, and Truffle to structure and organize your tests effectively.
In many cases, a hybrid approach is beneficial. You can write high-level tests in JavaScript for speed and front-end integration, and more granular tests in Solidity for performance optimization and security.
FAQs
Is it better to test in Solidity or JavaScript for Ethereum smart contracts?
- It depends on the project. Solidity offers more accuracy for low-level details and security, while JavaScript provides faster testing cycles and easier integration with front-end development.
Can I use both Solidity and JavaScript for testing?
- Yes, many developers use both. Solidity for precision and security testing, and JavaScript for faster feedback and broader integration with other tools.
Which testing frameworks are available for Solidity?
- Solidity-based tests can be written using Truffle or Hardhat, which simulate the Ethereum environment and provide tools for writing, executing, and organizing tests.
Why is JavaScript better for front-end dApp integration?
- JavaScript is widely used for front-end development, making it easier to test both smart contract logic and user interface interactions in the same environment.
Are JavaScript tests as reliable as Solidity tests?
- While JavaScript tests are faster and easier to write, they may lack precision when dealing with EVM-specific behavior. Solidity tests are more reliable for low-level, performance-critical, and security-focused applications.