In modern JavaScript development, modules have become an essential tool for organizing code. This article explores what JavaScript modules are, how they enhance code organization, and offers a detailed guide on using the import
and export
statements effectively. Whether you’re a beginner or an experienced developer, this article provides in-depth insights and practical examples to help you write clean, maintainable, and scalable code.
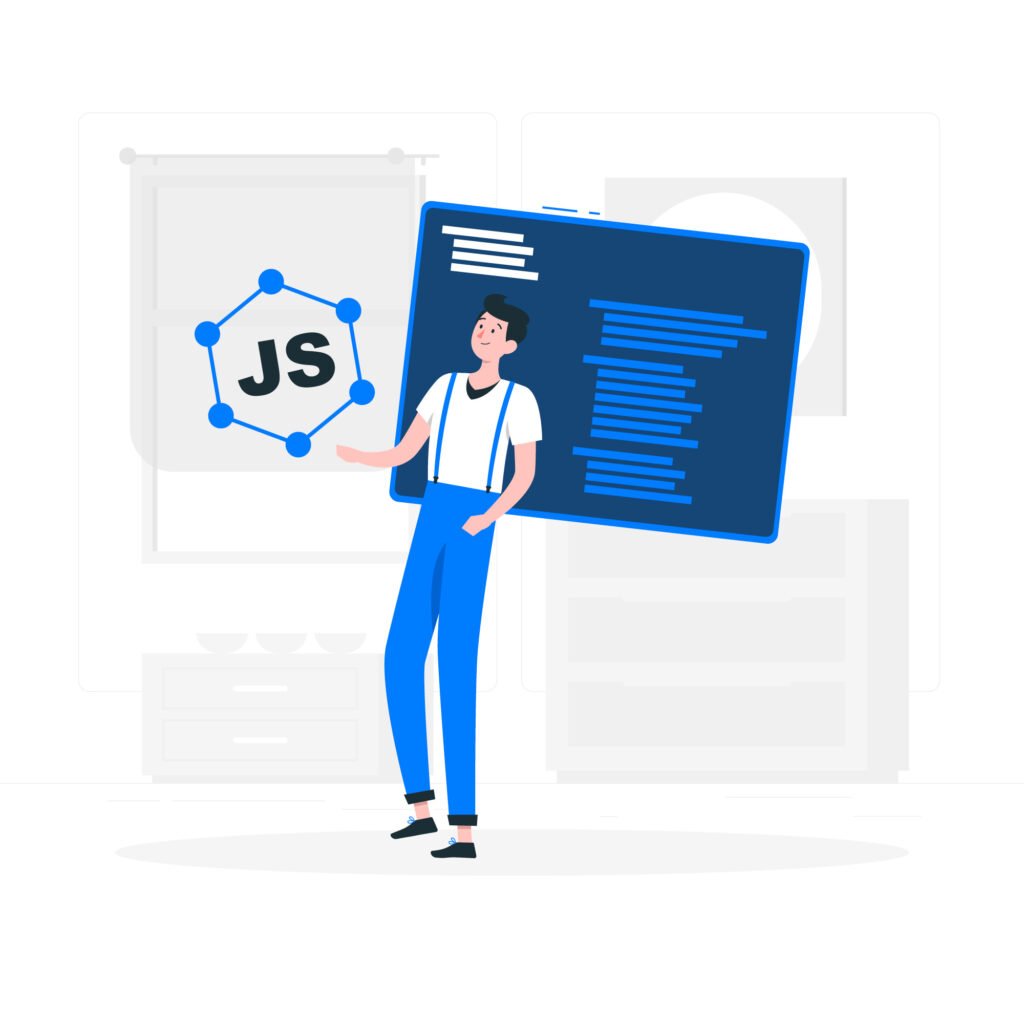
TL;DR
This guide explains that JavaScript modules are self-contained code units that improve maintainability by encapsulating functionality. It covers both named and default exports, demonstrates best practices for using the import
and export
statements, and discusses advanced topics like dynamic imports and handling circular dependencies. With practical examples, directory organization tips, and SEO strategies, this article is designed to help developers write modular, scalable, and optimized code.
Understanding JavaScript Modules
What Are JavaScript Modules?
JavaScript modules are self-contained pieces of code that encapsulate functionality, making it easier to reuse and maintain. Modules allow developers to split code into separate files and functions, each with its own scope. This design ensures that variables and functions do not leak into the global scope, reducing the likelihood of conflicts and bugs.
Prior to the advent of modules, developers used patterns like Immediately Invoked Function Expressions (IIFE) or namespaces to emulate private scope. With the introduction of ES6, JavaScript adopted a native module system that allows you to export variables, functions, classes, or objects from one file and import them into another.
The Evolution of JavaScript Code Organization
Historically, JavaScript was written as monolithic scripts embedded within HTML. As applications grew more complex, the need for a better way to organize code became evident. Early methods—such as using libraries like RequireJS or module loaders—were stepping stones toward the robust module system we now have with ES6. This evolution has led to a more modular, maintainable, and testable approach to software development.
Improving Code Organization with Modules
Benefits of Modular Code
Using modules offers several compelling benefits:
- Encapsulation: Modules encapsulate functionality, preventing unwanted interference from other parts of the code. This isolation minimizes side effects and enhances security.
- Reusability: Once a module is written, it can be reused across multiple projects without modification, significantly reducing development time.
- Maintainability: Breaking down code into smaller, logically grouped files makes it easier to debug, update, and scale.
- Improved Readability: With clearly defined responsibilities for each module, the overall code structure becomes much easier to understand.
- Enhanced Collaboration: Modular code allows multiple developers to work on different parts of a project concurrently without causing conflicts.
Real-World Examples of Modular Code
Imagine building a web application that includes user authentication, data fetching, and UI rendering. Instead of lumping everything into a single file, you can create separate modules for each functionality:
- auth.js: Handles user login, logout, and registration.
- api.js: Manages API calls and data fetching.
- ui.js: Contains functions for rendering the user interface.
By separating concerns, each module can be developed, tested, and maintained independently, leading to a cleaner, more scalable application.
The Import and Export Mechanism
Exporting Code: Named vs. Default Exports
In ES6 modules, you can export code in two primary ways: named exports and default exports.
Named Exports
Named exports allow you to export multiple variables or functions from a single file. When you import a named export, you must use the exact name it was exported with. Here’s a quick example:
// math.js
export const add = (a, b) => a + b;
export const subtract = (a, b) => a - b;
In another file, you can import these functions:
// app.js
import { add, subtract } from './math.js';
console.log(add(5, 3)); // Outputs: 8
Default Exports
Default exports allow you to export a single value from a file. When importing a default export, you can name it whatever you want:
// greeting.js
export default function greet(name) {
return `Hello, ${name}!`;
}
And import it as:
// app.js
import greet from './greeting.js';
console.log(greet('Alice')); // Outputs: Hello, Alice!
Importing Code: Syntax and Best Practices
The import
statement is flexible and can be used in several ways:
- Importing Named Exports:
Use curly braces to import specific functionalities:
import { add, subtract } from './math.js';
- Importing All Exports as an Object:
Sometimes you want to import everything from a module as an object:
import * as math from './math.js';
console.log(math.add(5, 3)); // Outputs: 8
- Combining Default and Named Imports:
When a module has both a default export and named exports, you can combine them:
import greet, { add, subtract } from './module.js';
Using these techniques effectively keeps your code neat and helps avoid naming conflicts.
Best Practices for Using Modules Effectively
Organizing Your Project Directory
A well-organized project directory is crucial when working with modules. Here are some tips to keep your codebase clean:
- Group Related Modules: Organize modules by feature or functionality. For example, group all authentication-related files in an
auth
folder. - Use Clear Naming Conventions: Name your files and directories in a way that clearly indicates their purpose.
- Index Files: Consider using index files (e.g.,
index.js
) to re-export modules. This allows you to simplify your import statements.
Example directory structure:
/src
/auth
login.js
register.js
index.js
/api
fetchData.js
postData.js
index.js
/components
header.js
footer.js
index.js
app.js
Tips for Large-Scale Applications
When working on larger projects, the following practices can be especially beneficial:
- Modularize by Feature: Instead of splitting code by type (e.g., all functions in one folder), consider grouping by feature. This helps maintain a clear separation of concerns.
- Consistent Coding Standards: Use tools like ESLint and Prettier to enforce coding standards across modules.
- Leverage Code Splitting: Use dynamic imports to load modules only when needed. This can significantly improve application performance.
- Document Module Interfaces: Write clear documentation for each module, outlining its purpose, expected inputs, and outputs. This is invaluable for collaboration and future maintenance.
Advanced Topics in JavaScript Modules
Dynamic Imports and Code Splitting
Dynamic imports allow you to load modules asynchronously at runtime. This feature is particularly useful for large applications where loading all modules at once would hinder performance. Dynamic imports use the import()
function:
// Dynamically import a module when needed
import('./math.js')
.then(math => {
console.log(math.add(5, 3));
})
.catch(err => {
console.error('Module failed to load', err);
});
By using dynamic imports, you can split your code into chunks and load them on demand, thereby reducing the initial load time of your application.
Handling Circular Dependencies
Circular dependencies occur when two or more modules depend on each other, leading to potential issues in module loading. Although ES6 modules handle some circular dependencies gracefully, it’s best to avoid them if possible. Strategies to mitigate circular dependencies include:
- Refactoring shared logic into separate utility modules.
- Reorganizing your module structure to minimize interdependencies.
- Using dependency injection to pass dependencies explicitly.
Comparing ES6 Modules with CommonJS
Before ES6 modules, Node.js primarily used the CommonJS module system. The key differences include:
- Syntax: ES6 uses
import
/export
while CommonJS usesrequire
/module.exports
. - Execution: ES6 modules are statically analyzed, enabling optimizations like tree shaking. CommonJS modules are loaded at runtime, which may lead to performance overhead.
- Usage Environment: While CommonJS is still widely used in Node.js applications, modern front-end development typically favors ES6 modules for their simplicity and improved performance.
FAQs
What exactly are JavaScript modules?
- JavaScript modules are self-contained units of code that encapsulate functionality. They allow you to export variables, functions, classes, or objects from one file and import them into another, thereby promoting code reuse and reducing global namespace pollution.
How do modules improve code organization?
- Modules improve code organization by breaking your code into smaller, manageable pieces. This separation of concerns makes it easier to maintain, debug, and scale your code. Each module handles a specific functionality, reducing the complexity of the overall codebase.
What is the difference between named and default exports?
- Named exports allow you to export multiple items from a module and require you to use the exact names when importing. Default exports, on the other hand, export a single value from a module, which you can import with any name of your choice.
Can I mix named and default exports in the same module?
- Yes, you can. You can have one default export along with one or more named exports in a single module. When importing, you must handle them appropriately—default exports can be named arbitrarily, while named exports must match the exported names.
What are dynamic imports, and why are they useful?
- Dynamic imports allow you to load modules asynchronously using the
import()
function. This technique is particularly useful for code splitting, where parts of your application are loaded on demand, thereby improving performance by reducing the initial load time.