In Solidity, Bytes32 is a data type that holds exactly 32 bytes of data, which equals 256 bits. It’s commonly used because it’s efficient for managing information on the Ethereum Virtual Machine (EVM). This data type is especially useful for handling things like cryptographic hashes (such as SHA256 or Keccak256), fixed-size identifiers, Ethereum addresses, or any other data that has a fixed length. The reason Bytes32 is so popular is that the EVM works in 256-bit chunks, meaning Bytes32 fits perfectly into how the system processes data. This helps save gas (transaction costs) and makes the storage of data more efficient.
Understanding Data Types in Ethereum Smart Contracts
Bytes32 is one of the most important and commonly used data types in Solidity, especially in Ethereum smart contracts. Solidity, the primary programming language for writing Ethereum-based applications, uses Bytes32 for efficient data storage and manipulation. In this article, we’ll explore what Bytes32 is, how it compares to other data types, and why it is crucial for building secure and efficient smart contracts.
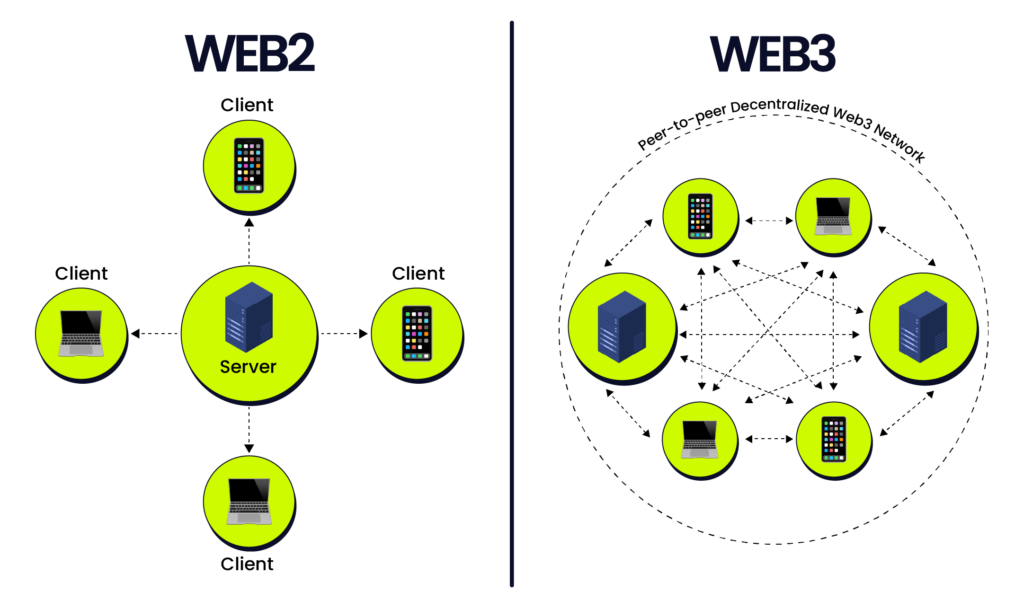
Understanding Data Types in Solidity
Solidity offers a variety of data types to developers, ranging from integers (uint
), addresses (address
), to dynamic arrays like bytes
and string
. However, Bytes32 stands out as a fixed-size byte array that is commonly used when you need to store data in a precise and space-efficient manner.
Bytes32 holds 32 bytes of data, which is equivalent to 256 bits, making it an ideal choice for storing hashes, addresses, and fixed-length strings. Understanding the role of data types in Solidity is crucial for optimising the performance of smart contracts and minimizing gas costs.
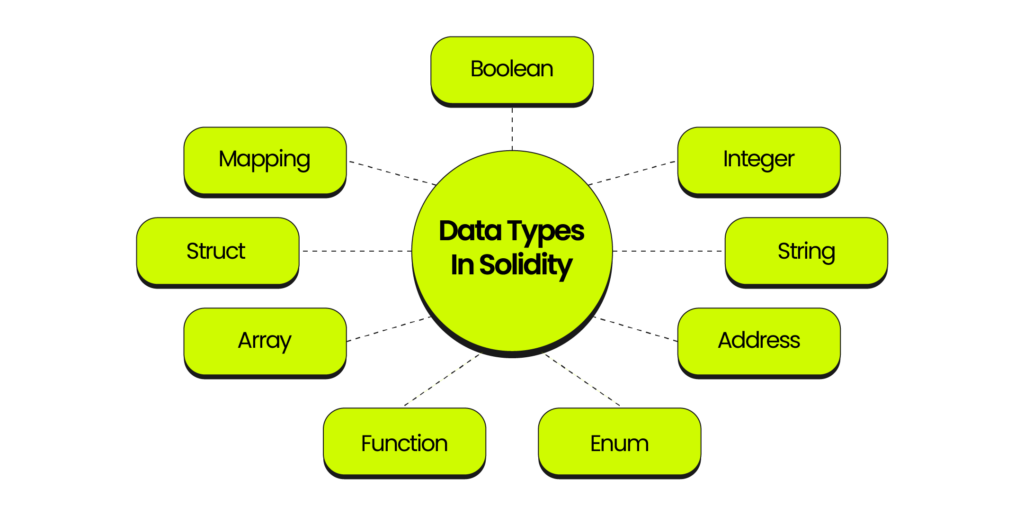
What is Bytes32 in Solidity?
Bytes32 in Solidity is a type that represents a static byte array of exactly 32 bytes. It is frequently used for:
- Hash values (e.g., SHA-256 or Keccak-256)
- Ethereum addresses
- Fixed-size strings
Example:
// Declaring a Bytes32 variable in Solidity
bytes32 myBytes32Value = 0x5465737456616c75654f6642797465733332;
In the example above, we declare a Bytes32 variable and assign it a hexadecimal value. This fixed-size array is efficient in terms of both storage and computation when working with blockchain applications.
How to Use Bytes32 in Solidity: Code Examples
To give you a better idea of how Bytes32 is used in Solidity, here are a few practical examples:
Storing Hashes
One of the most common use cases for Bytes32 is to store hash values. Ethereum uses Keccak-256 (a variant of SHA-3) for hashing, and the resulting output is a Bytes32 type.
bytes32 public hashValue = keccak256(abi.encodePacked("example data"));
This code snippet generates a hash of the string "example data"
and stores it as a Bytes32 variable. This is useful in various blockchain scenarios like proof-of-existence and verifiable signatures.
Storing Ethereum Addresses
While Solidity has a dedicated address
type, Bytes32 can also be used to store Ethereum addresses in a more compact form.
address public myAddress = 0x1234567890abcdef1234567890abcdef12345678;
bytes32 public compactAddress = bytes32(uint256(uint160(myAddress)));
Here, the Ethereum address is cast into a Bytes32 type. This can be beneficial when working with large datasets or minimizing gas consumption.
Bytes32 vs. Other Data Types: Pros and Cons
When should you choose Bytes32 over other data types like string
, bytes
, or uint
? Here’s a quick comparison of Bytes32 with other common Solidity types:
Data Types | Description | Pros | Cons |
---|---|---|---|
Bytes32 | Fixed-length byte array | Efficient storage, fast access | Limited to 32 bytes |
String | Dynamic-length string | More flexible for text | Higher gas cost |
Bytes | Dynamic-length byte array | Variable size | Less efficient for fixed-size data |
Uint256 | Unsigned integer | Ideal for numeric operations | Cannot store non-numeric data |
Common Pitfalls and Best Practices with Bytes32
1. Converting Between String and Bytes32
One common mistake developers make is attempting to convert strings to Bytes32 directly, especially when dealing with fixed-size strings.
string memory myString = "hello";
bytes32 public myBytes32 = bytes32(bytes(myString));
While this method works, be cautious when handling strings longer than 32 characters, as the extra data will be truncated, potentially leading to data loss.
2. Optimizing for Gas
Since Bytes32 is a fixed-size array, it offers performance benefits by consuming less gas during operations like hashing or storing data. To maximize gas efficiency, always use Bytes32 when dealing with fixed-length data, such as hashes or addresses.
Best Practices:
- Use Bytes32 for storing hashes or addresses when precision is required.
- Avoid using Bytes32 for data that could exceed 32 bytes, as it will lead to truncation.
- Always use Solidity’s abi.encodePacked for hashing multiple values.
Conclusion: Why Bytes32 Matters in Solidity
Bytes32 is a powerful data type that significantly improves the efficiency of smart contracts in Ethereum. Whether you’re working with hashes, addresses, or fixed-size data, using Bytes32 can minimize storage costs and enhance performance. Understanding the strengths and limitations of Bytes32 will help you write better, more efficient smart contracts that are both gas-efficient and secure.
By tapping into the potential of Bytes32, Solidity developers can build more efficient applications that reduce both storage and execution costs. This optimization is key to helping Ethereum-based systems scale smoothly while staying fast and lightweight..
FAQs
Can you convert Bytes32 to a string in Solidity?
- Yes, you can convert Bytes32 to a string, but care must be taken to ensure the conversion doesn’t truncate valuable data.
Here’s an example:
function bytes32ToString(bytes32 _bytes32) public pure returns (string memory) { return
string(abi.encodePacked(_bytes32));<br>
}
How does gas usage differ between Bytes32 and string?
- Bytes32 is more gas-efficient than
string
due to its fixed size. String variables consume more gas since they are dynamic and require additional memory management.
Why is Bytes32 commonly used in Ethereum?
- Bytes32 is ideal for storing hashes and other fixed-length data due to its balance between memory efficiency and processing speed.