Imagine a world where you can leverage the same language, JavaScript, that brings websites to life, to power the engines behind those websites. That’s the magic of Node.js! In this comprehensive guide, we’ll embark on a journey to understand Node.js, its functionalities, and why it’s become a game-changer in web development.
JavaScript
Traditionally, JavaScript has reigned supreme in the realm of web browsers. It’s the language that animates web pages, validates user input, and creates dynamic interactions. But Node.js shatters this confinement, introducing a runtime environment that executes JavaScript code outside the browser, directly on the server.
What is Node.js ?
In simpler terms, Node.js acts as a bridge between JavaScript and the server. It’s an open-source, cross-platform runtime environment built on Google Chrome’s powerful V8 JavaScript engine. This engine is responsible for taking your JavaScript code and turning it into machine-readable instructions, allowing it to function outside the browser’s limitations.
Why Use Node.js ?
Node.js offers a plethora of benefits that have propelled it to the forefront of web development. Here are some key reasons why you should consider using it:
- JavaScript All the Way: For web developers already familiar with JavaScript, Node.js presents a familiar and efficient environment. You can leverage your existing skills to write both front-end (browser-side) and back-end (server-side) code using the same language, streamlining development and reducing the learning curve.
- Speedy Performance: Node.js is renowned for its exceptional performance, particularly when it comes to handling real-time applications and asynchronous tasks. Its event-driven, non-blocking I/O (Input/Output) model allows it to handle numerous concurrent connections efficiently. Imagine a server juggling multiple requests simultaneously – Node.js excels at this, keeping things smooth and responsive.
- Scalability for Growth: As your web application scales and attracts more users, Node.js can gracefully adapt. Its architecture allows for easy horizontal scaling, meaning you can add more servers to handle the increased traffic. This makes it ideal for applications that experience bursts of activity or have a steadily growing user base.
- Package Powerhouse: The npm Advantage: Node.js boasts a vibrant ecosystem with a vast repository of pre-written code snippets called modules. This treasure trove is known as the Node Package Manager (npm). With npm, you can discover and install ready-made solutions for various functionalities, saving you time and effort when building your application.
- Real-time Applications Made Easy: Node.js shines in the world of real-time applications like chat rooms, collaborative editing tools, and online multiplayer games. Its event-driven architecture facilitates seamless communication between users in real-time, keeping everyone on the same page.
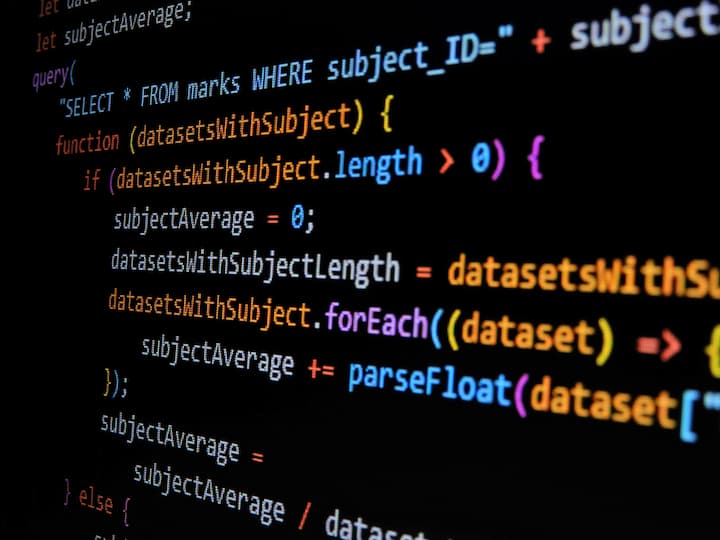
Exploring the Core Concepts of Node.js
Now that we’ve been dazzled by the benefits of Node.js, let’s delve into some fundamental concepts that make it tick:
- Event Loop: The Maestro of Execution: The event loop is the heart of Node.js. It continuously monitors for events (like incoming requests or data completion) and triggers appropriate callbacks (functions) to handle them. This non-blocking approach ensures that the server doesn’t get bogged down waiting for one task to finish before moving on to the next.
- Modules: Building Blocks of Functionality: As mentioned earlier, modules are reusable code snippets that provide specific functionalities. They can be created by developers and shared through npm, allowing you to incorporate pre-written solutions into your projects without reinventing the wheel.
- Streams: Efficient Data Flow Management: Streams are a powerful concept in Node.js that enable handling large amounts of data efficiently. They provide a way to process data in chunks, rather than loading everything into memory at once. This is particularly useful for scenarios like file uploads, video streaming, and real-time data processing.
- Callbacks and Promises: Handling Asynchronous Operations: Node.js thrives on asynchronous operations, meaning tasks don’t necessarily complete instantly. Callbacks and promises are mechanisms used to manage the flow of execution when dealing with asynchronous tasks. They provide a way to specify what code should run once an operation finishes, ensuring a smooth and predictable program flow.
Building Your First Node.js Application
Let’s get our hands dirty and create a simple Node.js application that serves a basic webpage. Here’s a step-by-step breakdown:
- Installation:
Download and install the latest stable version of Node.js from the official website. The installation process is straightforward and shouldn’t take long. Once complete, you can verify the installation by opening your terminal or command prompt and typingnode -v
. This should display the installed Node.js version. - Creating Your First Script:
Now, let’s create a simple JavaScript file that will act as our Node.js application. Open a text editor and create a new file namedhello_world.js
. Paste the following code snippet into the file:
const http = require('http');
const hostname = 'localhost';
const port = 3000;
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello, World!\\n');
});
server.listen(port, hostname, () => {
console.log(`Server running at <http://$>{hostname}:${port}/`);
});
- Breaking Down the Code:
const http = require('http');
: This line imports the built-in http module, which provides functionalities for creating a web server.const hostname = 'localhost';
andconst port = 3000;
: These lines define the hostname (localhost) and port number (3000) on which our server will listen for incoming requests.const server = http.createServer((req, res) => {...});
: This line creates a new HTTP server instance using the http.createServer method. The provided function defines how the server will respond to incoming requests.req
(request): This object represents the incoming HTTP request containing information like the requested URL, headers, and any data sent by the client.res
(response): This object represents the HTTP response that our server will send back to the client. We can use it to set the status code, headers, and the content of the response.
res.statusCode = 200;
: This line sets the status code of the response to 200, indicating success.res.setHeader('Content-Type', 'text/plain');
: This line sets the content type header of the response to text/plain, signifying that the response body contains plain text.res.end('Hello, World!\\n');
: This line sends the actual content of the response, which is a simple “Hello, World!” message followed by a newline character.server.listen(port, hostname, () => {...});
: This line starts the server, listening for incoming requests on the specified hostname and port. The provided callback function gets executed once the server is up and running, logging a message to the console.
- Running the Application:
Save thehello_world.js
file and open your terminal or command prompt. Navigate to the directory where you saved the file using the cd command. Now, execute the following command to run your Node.js application:
node hello_world.js
This will start the server, and you should see a message displayed in the terminal indicating that the server is running on http://localhost:3000/
.
- Accessing Your Application:
Open your web browser and navigate to the URLhttp://localhost:3000/
. If everything is set up correctly, you should see the message “Hello, World!” displayed on the webpage. Congratulations, you’ve just created and run your first Node.js application!
Exploring Further
This basic example serves as a stepping stone into the vast world of Node.js development. As you delve deeper, you’ll discover numerous frameworks and libraries that empower you to build complex and dynamic web applications. Here are some exciting areas to explore:
- Express.js: A popular web framework built on top of Node.js that simplifies building web applications by providing a structured approach for handling routes, middleware, and templating.
- Socket.IO: A library that enables real-time communication between web servers and clients. This is perfect for building chat applications, collaborative editing tools, and multiplayer games.
- Database Integration: Node.js seamlessly integrates with various databases like MongoDB, MySQL, and PostgreSQL, allowing you to store and retrieve data for your applications.
Conclusion
Node.js has revolutionized the way we build web applications. Its ability to leverage JavaScript on both the front-end and back-end offers a powerful and efficient development environment. By harnessing the knowledge from this guide, exploring the recommended resources, and continuously learning, you’ll be well on your way to becoming a proficient Node.js developer and creating dynamic web applications that power the future. Remember, the journey is just as important as the destination, so have fun experimenting, building, and contributing to the ever-evolving world of Node.js!
FAQs
What is Node.js?
- Node.js is a JavaScript runtime built on Chrome’s V8 engine, allowing you to build scalable and high-performance applications.
What are the main features of Node.js?
- Node.js features include asynchronous event-driven architecture, non-blocking I/O operations, and a single-threaded model for handling multiple connections efficiently.
What are the common uses of Node.js?
- Node.js is commonly used for server-side development, real-time applications, API services, and microservices.
Why should I use Node.js for my project?
- Node.js offers high performance, scalability, a large ecosystem of libraries, and seamless integration with JavaScript, making it ideal for modern web applications.
How does Node.js handle multiple connections?
- Node.js uses a single-threaded event loop with non-blocking I/O operations to efficiently handle multiple connections without creating new threads for each request.
What is the difference between Node.js and traditional server-side scripting?
- Traditional server-side scripting often relies on multi-threading, while Node.js uses a non-blocking, event-driven architecture for better performance and scalability.
Can I use Node.js for frontend development?
- While Node.js is primarily for server-side development, it can be used in frontend development tools like build systems, package managers, and task runners.
What is npm in the context of Node.js?
- npm (Node Package Manager) is a package manager for Node.js that helps developers install, update, and manage reusable code modules.
Is Node.js suitable for enterprise applications?
- Yes, Node.js is suitable for enterprise applications due to its scalability, performance, and a large ecosystem of modules and tools.
How do I install Node.js on my system?
- You can install Node.js from its official website by downloading the appropriate installer for your operating system, or use a package manager like Homebrew for macOS.