The internet thrives on data exchange. From social media updates to online shopping carts, information constantly zips between servers and your device. But how does this data travel in a way that different systems can understand? Enter JavaScript and JSON, the dynamic duo that makes web data exchange smooth and efficient.
JavaScript
Imagine JavaScript (JS) as a all in one toolbox for web development. It’s a programming language that breathes life into static web pages, making them interactive and responsive. JS can manipulate elements on the page, respond to user actions, and even communicate with servers.
One of JS’s core strengths is its ability to work with data. It can store information in various formats, like numbers, text, and even complex structures like objects and arrays. These data structures become the building blocks of web applications.
What is JSON?
JavaScript Object Notation (JSON) is a lightweight data format designed specifically for data interchange. Think of it as a universal language that computers can understand, regardless of their programming language. JSON uses a simple text-based structure similar to JS object literals, making it easy for humans to read and write.
Here’s what makes JSON special:
- Lightweight: JSON files are compact, making them ideal for transferring data over networks without slowing things down.
- Human-Readable: The structure is easy to understand, even for those without programming experience.
- Language-Independent: Any programming language can parse and utilize JSON data.
Why Use JavaScript and JSON Together?
The synergy between JS and JSON is what makes them a powerful combination for web development. Here’s why they work so well together:
- Seamless Conversion: JS provides built-in functions to effortlessly convert between JSON strings and JavaScript objects. This allows you to easily manipulate data received in JSON format.
- Shared Syntax: The similarity between JSON structure and JS object literals makes working with JSON data intuitive for JS developers.
- Ubiquitous Usage: JSON is the go-to format for data exchange on the web. This makes JS, with its native JSON support, a perfect fit for building web applications that communicate with servers and APIs.
JSON Structure
JSON data is organized using two fundamental building blocks:
- Key-Value Pairs: These are the heart of JSON. Each pair consists of a key (a string in quotes) and a value. Keys act like labels, identifying the data associated with them. Values can be various data types, including strings, numbers, booleans (true/false), arrays, or even nested objects.
"name": "Alice",
"age": 30,
"is_active": true
- Arrays: An ordered collection of values enclosed in square brackets []. Arrays are useful for storing lists of items, such as product details or user preferences.
["apple", "banana", "orange"]
Working with JSON Data in JavaScript
Here’s a glimpse into how JS interacts with JSON data:
- Fetching JSON Data: JS can use techniques like fetch API or libraries like Axios to retrieve JSON data from a server.
- Parsing JSON: The received JSON string needs to be converted into a JavaScript object for manipulation. JS provides the JSON.parse() function for this purpose.
const jsonString = '{"name": "Bob", "occupation": "developer"}';
const jsonData = JSON.parse(jsonString);
console.log(jsonData.name); // Outputs: "Bob"
- Accessing Data: Once parsed, you can access data within the object using its key names. Dot notation or bracket notation can be used for this.
console.log(jsonData["occupation"]); // Outputs: "developer"
- Modifying Data: JavaScript objects are mutable, meaning you can change their properties after parsing.
jsonData.age = 25;
console.log(jsonData); // Now the object includes "age": 25
- Serializing Back to JSON: If you need to send the modified data back to the server, you can convert the JavaScript object back to a JSON string using JSON.stringify().
const updatedJsonString = JSON.stringify(jsonData);
Advanced Techniques for Powerful Data Manipulation
While the above provides a foundation, JS offers powerful tools for more complex data manipulation:
- Looping through Arrays: Iterate over each element in an array using loops like for or forEach to access and process data.
- Iterating through Objects: Use techniques like for…in loops or object methods like Object.keys() to access properties (key-value pairs) within an object.
- Conditional Statements: Employ if, else if, and else statements to make decisions based on data values within the JSON object.
- Functions for Reusability: Encapsulate common data manipulation tasks within functions to improve code organization and reusability.
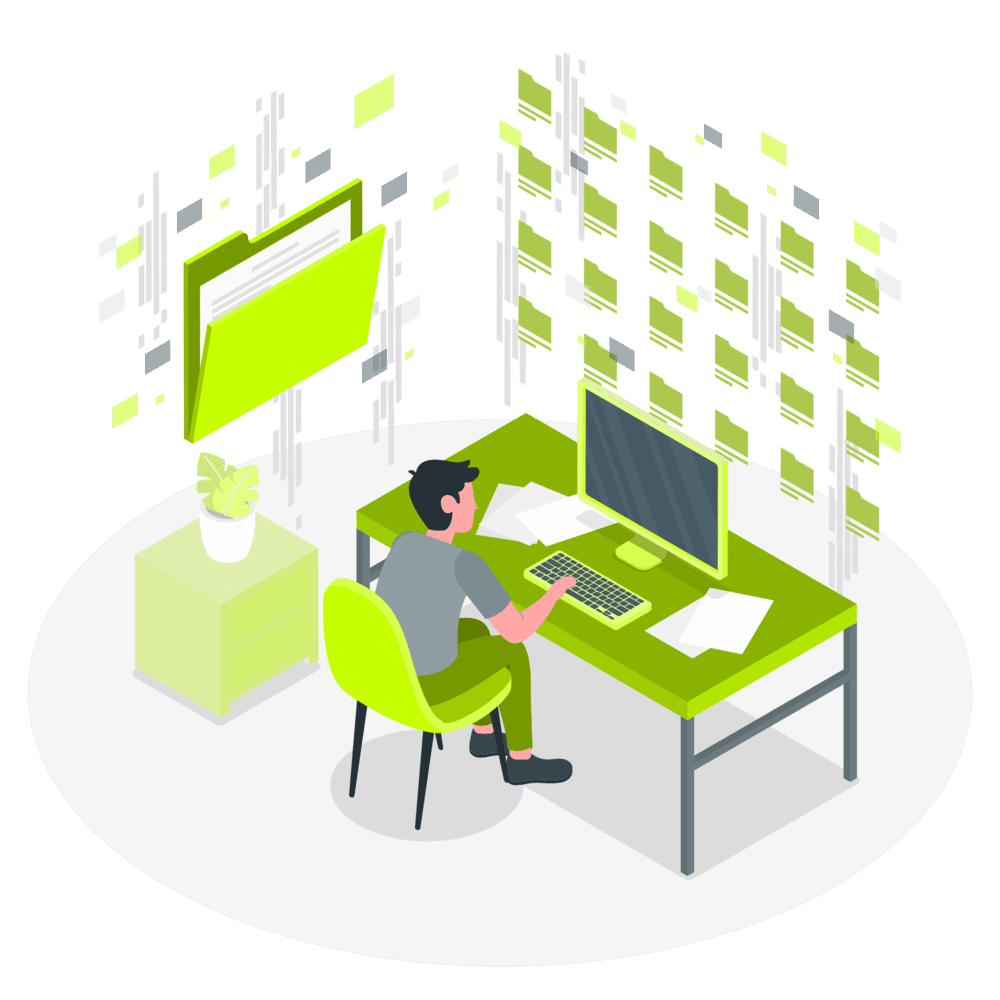
Examples in Action
Let’s explore some real-world scenarios where JS and JSON work together:
- Fetching and Displaying Product Data: An e-commerce website might use JS to fetch product details in JSON format from a server. It can then parse the JSON data, extract product information like name, price, and image URL, and dynamically populate the product page with this information.
- User Login and Authentication: When a user logs in, JS can send login credentials (username and password) to the server in JSON format. The server verifies the credentials and sends back a response, potentially in JSON, indicating success or failure. JS can then handle the login flow based on the received response.
- Interactive To-Do Lists: A to-do list application might store to-do items in a JSON file. JS can read the JSON file, create a user interface displaying the tasks, and allow users to add, edit, or mark tasks as complete. The updated list can then be saved back to the JSON file using JS.
The Power of Node.js
While JS shines in web browsers, Node.js extends its capabilities to the server-side. Node.js allows you to build applications that handle data exchange between servers using JSON. This enables features like creating APIs that provide data to web applications or building real-time applications with data constantly flowing between clients and servers.
Error Handling
When working with data, errors are inevitable. Here’s how to make your JS code robust:
- Try…Catch Blocks: Use these blocks to gracefully handle potential errors during JSON parsing or data manipulation.
- Data Validation: Before using data, implement checks to ensure it’s in the expected format and doesn’t contain unexpected values.
Security Considerations
- Sanitize User Input: Never trust data received from users directly. Sanitize it to remove potentially harmful code before processing it.
- Validate Data: Ensure data received in JSON format adheres to expected structure and data types to prevent unexpected behavior.
Conclusion
By mastering JavaScript and JSON, you unlock the power to build dynamic and data-driven web applications. Their ease of use, efficiency, and universality make them an essential skillset for any web developer. So, dive into the world of JSON and JS, and start creating interactive and powerful web experiences!
FAQs
What is JavaScript?
- JavaScript is a programming language commonly used to create interactive effects within web browsers.
What is JSON?
- JSON (JavaScript Object Notation) is a lightweight data-interchange format that’s easy for humans to read and write and easy for machines to parse and generate.
How do you parse JSON in JavaScript?
- Use
JSON.parse()
to convert a JSON string into a JavaScript object.
How do you convert a JavaScript object to JSON?
- Use
JSON.stringify()
to convert a JavaScript object into a JSON string.
What are common uses of JSON in web development?
- JSON is used for transmitting data between a server and a web application, such as API responses and configuration files.
How do you fetch JSON data from an API in JavaScript?
- Use the
fetch()
function to send an HTTP request to the API and then parse the response withresponse.json()
.
What is the difference between JSON and XML?
- JSON is less verbose and easier to parse than XML, making it a more efficient choice for data interchange.
Can you store arrays in JSON?
- Yes, JSON supports arrays, allowing you to store a list of values in a JSON array.
How do you handle errors when parsing JSON in JavaScript?
- Use a try-catch block to handle errors that may occur during JSON parsing.
What are the security considerations when using JSON?
- Be cautious of JSON injection attacks. Always validate and sanitize JSON data received from untrusted sources.