Web3 development thrives on smart contracts – self-executing programs on the blockchain. These contracts are written in high-level languages like Solidity and then compiled into machine code that the blockchain understands. However, a hidden threat lurks within the compilation process itself: Compiler Bugs. When these bugs occur, they can introduce unintended behavior into smart contracts, potentially leading to devastating consequences. Let’s dive into the nature of compiler bugs and how they can impact Web3 projects.
A Glitch in the Matrix
Imagine a developer meticulously writing a secure smart contract for a DeFi application. They compile the code using a Solidity compiler, confident in its ability to translate their instructions accurately. However, unbeknownst to them, a compiler bug exists. This bug might:
- Misinterpret Code: The compiler might misunderstand a specific line of code, generating machine code that deviates from the developer’s intended functionality. This can lead to unexpected behavior in the deployed smart contract.
- Optimization Gone Wrong: In an attempt to optimize the code for efficiency, the compiler might introduce unintended side effects. These could create vulnerabilities that attackers can exploit to manipulate the contract or steal user funds.
Real-World Wake-Up Calls
Compiler bugs have played a role in high-profile security breaches in Web3:
- The Vyper Compiler Issues (2020): The Ethereum Foundation discovered several serious bugs in the Vyper compiler, an alternative to Solidity. These bugs could have potentially led to vulnerabilities in smart contracts written in Vyper.
- The Parity Multisig Hack (2017): While not a direct compiler bug, a flaw in the compilation process for the Parity multi-signature wallet contract contributed to a critical security vulnerability that resulted in the loss of millions of dollars worth of Ether.
Building Secure Smart Contracts
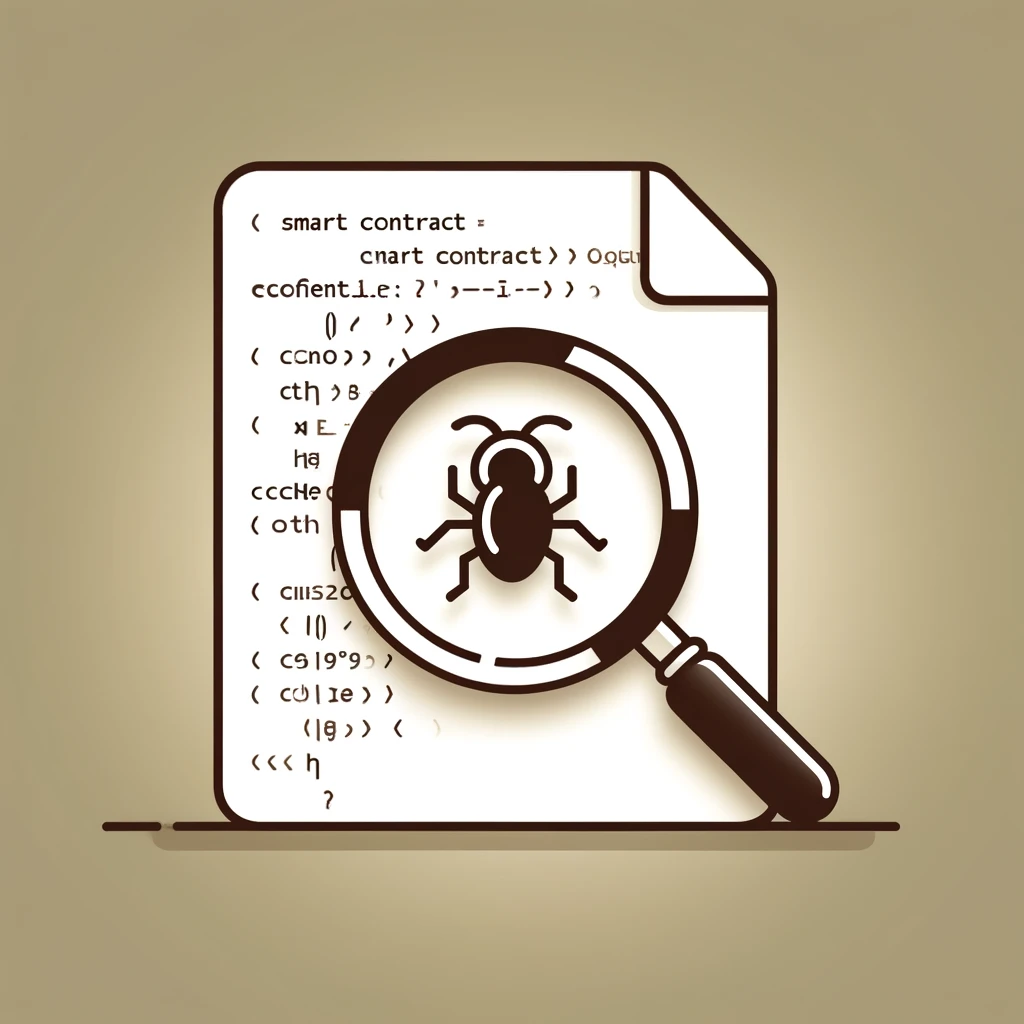
Web3 developers can mitigate the risks of compiler bugs by following several best practices. One essential practice is to always use the latest versions of Solidity and other Web3 compilers. These updated versions often include bug fixes and security patches identified in previous releases, reducing the risk of encountering known compiler issues.
Another effective strategy is multiple compiler testing. If possible, developers should compile their smart contracts with different compatible compilers. This approach can help identify potential compiler-specific bugs that might not be apparent with a single compiler, providing an additional layer of security.
Lastly, developers should consider employing formal verification techniques to mathematically prove the correctness of their smart contract logic. Formal verification can provide an extra layer of assurance beyond relying solely on the compiler, ensuring that the smart contract behaves as intended under all possible conditions.
A Collaborative Effort
Mitigating compiler bugs requires a collaborative approach, involving comprehensive security audits that not only focus on the smart contract code itself but also consider the potential impact of compiler bugs. Additionally, supporting the development of open-source compilers like Solidity is crucial, as it allows the community to identify and fix bugs more efficiently.
Compiler Bug Examples in Smart Contracts
To better understand the potential impact of compiler bugs, let’s look at some examples using Solidity and JavaScript.
Example 1: Solidity Compiler Bug
Consider a simple Solidity smart contract designed to manage a token balance:
solidityCopy code
pragma solidity ^0.8.0;
contract Token {
mapping(address => uint256) public balances;
function transfer(address recipient, uint256 amount) public {
require(balances[msg.sender] >= amount, "Insufficient balance");
balances[msg.sender] -= amount;
balances[recipient] += amount;
}
function deposit() public payable {
balances[msg.sender] += msg.value;
}
}
In this contract, a compiler bug might misinterpret the subtraction operation in the transfer
function, causing an overflow or underflow despite the checks in place. This could lead to incorrect balances being recorded, allowing for potential exploitation.
Example 2: JavaScript Compiler Bug
While JavaScript is not compiled in the same way as Solidity, transpilers like Babel convert modern JavaScript into backward-compatible versions. A bug in Babel could introduce errors. Consider the following ES6 code:
javascriptCopy code
class Token {
constructor() {
this.balances = new Map();
}
transfer(sender, recipient, amount) {
if (this.balances.get(sender) >= amount) {
this.balances.set(sender, this.balances.get(sender) - amount);
this.balances.set(recipient, (this.balances.get(recipient) || 0) + amount);
} else {
throw new Error("Insufficient balance");
}
}
deposit(account, amount) {
this.balances.set(account, (this.balances.get(account) || 0) + amount);
}
}
let token = new Token();
token.deposit("Alice", 100);
token.transfer("Alice", "Bob", 50);
console.log(token.balances);
A bug in the Babel transpiler might incorrectly transform the ES6 class syntax into ES5, leading to runtime errors or unexpected behavior in the transfer
method.
Identifying and Addressing Compiler Bugs
Detecting compiler bugs can be challenging, but there are several strategies developers can employ to minimize their impact:
- Comprehensive Testing: Write extensive unit and integration tests to cover all possible scenarios your smart contract might encounter. This can help catch deviations in behavior due to compiler bugs.
- Static Analysis Tools: Utilize static analysis tools to examine the bytecode generated by the compiler. Tools like Mythril, Slither, and others can identify common vulnerabilities and inconsistencies.
- Code Reviews: Conduct thorough code reviews with a focus on potential compiler issues. Peer reviews can help spot subtle problems that automated tools might miss.
- Community Feedback: Engage with the developer community to share experiences and learn about potential compiler bugs. Platforms like GitHub, Stack Exchange, and various blockchain forums are valuable resources for staying informed.
Conclusion
Compiler bugs pose a significant threat to the security and functionality of smart contracts in the Web3 ecosystem. By understanding the nature of these bugs and implementing best practices to mitigate their risks, developers can build more secure and reliable decentralized applications. Continuous learning, rigorous testing, and community collaboration are essential in safeguarding against the unforeseen consequences of compiler bugs.
FAQs
What are compiler bugs in the context of smart contracts?
- Compiler bugs are errors or vulnerabilities in the software that translates smart contract code into executable blockchain instructions.
How can compiler bugs impact smart contracts?
- They can introduce unexpected behavior or security vulnerabilities, potentially leading to financial losses or exploitation.
What steps can be taken to mitigate the risks of compiler bugs?
- Regularly update the compiler, use established and well-audited versions, and conduct thorough testing and code reviews.
Are compiler bugs common in Solidity smart contracts?
- While not extremely common, they do occur and can have significant impacts, making it crucial to stay vigilant and informed.
How can developers identify potential compiler bugs?
- By using multiple compilers, enabling all compiler warnings, and running extensive tests on different compiler versions.
What is a smart contract?
- A smart contract is a self-executing contract with the terms of the agreement directly written into code, running on a blockchain.
Why is compiler security important in blockchain development?
- Ensuring compiler security helps prevent hidden vulnerabilities that could compromise the integrity and functionality of smart contracts.
What tools can help in detecting compiler bugs?
- Tools like MythX, Slither, and other static analysis tools can help identify potential issues in smart contract code.
What is the role of auditing in smart contract development?
- Auditing involves an in-depth review of smart contract code to find and fix vulnerabilities, ensuring robust and secure blockchain applications.
How often should compilers be updated?
- Compilers should be updated regularly to benefit from the latest security patches and improvements, but updates should be tested thoroughly before deployment.