Solidity, the preeminent language for building smart contracts on the Ethereum blockchain and other platforms, empowers developers to create decentralized applications (DApps) that automate complex processes without centralized control. However, the immutability and distributed nature of blockchains make vulnerabilities in smart contracts particularly critical. A single security flaw can lead to the loss of millions of dollars in cryptocurrency, as evidenced by numerous high-profile hacks.
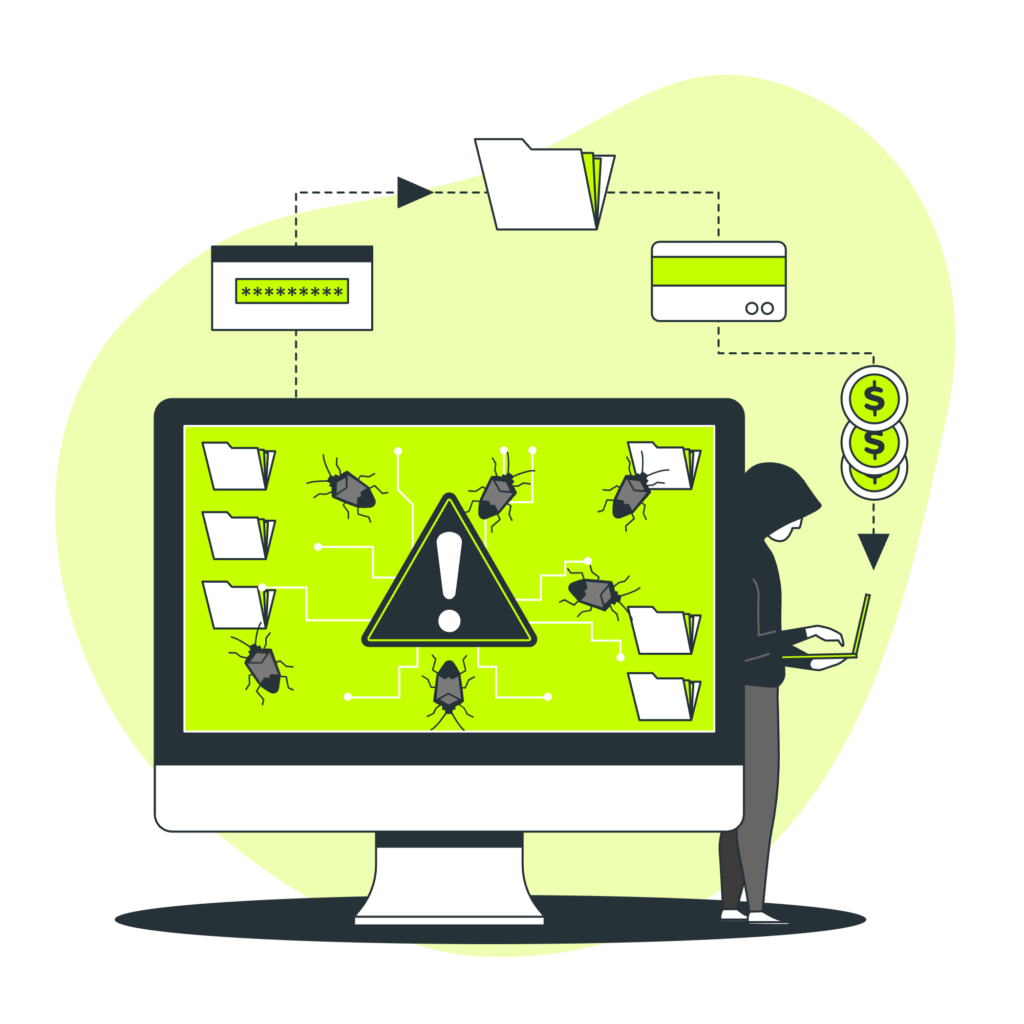
Here we delve into the most common Solidity security vulnerabilities and explores best practices to mitigate them. By understanding these pitfalls and adopting secure coding practices, developers can build robust and trustworthy smart contracts.
Reentrancy Attacks
One of the most prevalent vulnerabilities in Solidity smart contracts is the reentrancy attack. This exploit arises when a function makes an external call (e.g., to another contract) during its execution. The called contract can then re-enter the original function before the first call finishes, potentially allowing the attacker to manipulate the state of the contract multiple times within a single transaction.
Here’s a simplified example:
contract Vulnerable {
mapping(address => uint) public balances;
function withdraw(address payable recipient, uint amount) public {
balances[msg.sender] -= amount;
recipient.transfer(amount);
}
}
In this example, the withdraw function first subtracts the withdrawal amount from the sender’s balance and then sends the funds to the recipient. An attacker could exploit this by creating a malicious contract that, upon receiving funds in its fallback function, re-enters the withdraw function recursively, draining the sender’s balance before the initial transfer completes.
Prevention:
There are several ways to prevent reentrancy attacks:
- Checks and Effects Interaction Pattern: This pattern dictates performing all checks (e.g., balance sufficiency) before modifying the contract state. This ensures the function cannot be re-entered after a state change.
contract SecureWithChecks {
mapping(address => uint) public balances;
function withdraw(address payable recipient, uint amount) public {
require(balances[msg.sender] >= amount, "Insufficient funds");
balances[msg.sender] -= amount;
recipient.transfer(amount);
}
}
- Solidity’s nonReentrant modifier: Introduced in Solidity v2.5, this modifier automatically checks for reentrancy before allowing a function to call another contract.
contract SecureWithModifier {
mapping(address => uint) public balances;
modifier nonReentrant() {
_;
}
function withdraw(address payable recipient, uint amount) public nonReentrant {
require(balances[msg.sender] >= amount, "Insufficient funds");
balances[msg.sender] -= amount;
recipient.transfer(amount);
}
}
- Third-party libraries: Secure libraries like OpenZeppelin Contracts offer well-tested reentrancyGuard implementations that can be integrated into your contracts.
Integer Overflow/Underflow
Solidity uses integer data types for various calculations. However, these types have limitations – exceeding their maximum value can lead to overflow, and underflowing below the minimum can result in unexpected behavior.
An attacker could exploit these vulnerabilities to manipulate calculations within your contract, potentially leading to unintended effects like transferring more funds than intended.
Prevention:
- Use safe math libraries: Libraries like OpenZeppelin Contracts’ SafeMath provide functions for arithmetic operations that check for overflow and underflow, throwing an exception if encountered.
contract SecureMath {
using SafeMath for uint;
mapping(address => uint) public balances;
function transfer(address recipient, uint amount) public {
balances[msg.sender] = balances[msg.sender].sub(amount);
balances[recipient] = balances[recipient].add(amount);
}
}
- Consider alternative data types: If calculations involve large numbers, explore using libraries that offer big integer support for more precise calculations.
Uninitialized Storage Pointers: A Recipe for Disaster
In Solidity, storage variables are automatically initialized to zero. However, storage pointers (like mappings and arrays) are not automatically initialized, leaving them vulnerable to uninitialized state bugs.
contract UninitializedStorage {
mapping(address => uint) public balances;
function setBalance(address _user, uint _amount) public {
require(msg.sender == owner); // Only owner can set balances
balances[_user] = _amount;
}
}
- In this contract, if owner tries to set a user’s balance without initializing it first, it may result in unexpected behavior. For example, attempting to access balances[_user] before it’s set could return a random value or zero, depending on the blockchain state.
Prevention:
Always initialize storage pointers before using them. Use explicit default values or constructor functions to set initial values for mappings, arrays, and other storage variables.
contract InitializedStorage {
mapping(address => uint) public balances;
address public owner;
constructor() {
owner = msg.sender;
balances[msg.sender] = 0; // Initialize sender's balance to 0
}
function setBalance(address _user, uint _amount) public {
require(msg.sender == owner);
balances[_user] = _amount;
}
}
Denial of Service (DoS) Attacks: Bottlenecks and Loops
Smart contracts that involve loops or operations that consume excessive gas are susceptible to Denial of Service (DoS) attacks. Attackers can exploit these contracts to consume all available gas, rendering them unusable for legitimate transactions.
contract DoS {
uint[] public data;
function addData(uint _value) public {
data.push(_value); // Add data to the array
}
}
- In this contract, an attacker can repeatedly call addData with a large value, causing the array to grow indefinitely. This will eventually consume all available gas during the transaction, preventing further interactions with the contract until the attacker stops or the gas limit is reached.
Prevention:
- Implement gas limits for functions. This restricts the amount of gas a single transaction can consume, preventing infinite loops from halting the contract.
- Minimize gas consumption in loops and complex operations. Analyze code to identify areas for optimization and reduce unnecessary computations.
- Consider using designs that avoid the need for loops whenever possible. Explore alternative approaches that achieve the desired functionality without iterative processes.
contract SecureDoS {
uint[] public data;
function addData(uint _value) public {
require(data.length < 100); // Enforce a gas limit on array size
data.push(_value);
}
}
Gas Limitation: Dealing with Out-of-Gas Situations
Ethereum imposes gas limits on transactions to prevent infinite loops and resource exhaustion. Smart contract developers must be aware of these limits and handle out-of-gas situations gracefully.
contract GasLimitation {
function consumeGas() public {
while (true) { // Infinite loop
// Do something that consumes gas
}
}
}
In this contract, the infinite loop will eventually run out of gas and cause the transaction to fail. This can lead to a frustrating user experience and unexpected behavior.
Prevention:
- Use gasleft() to check the remaining gas in a function. This allows you to monitor gas consumption and avoid exceeding the limit.
- Avoid infinite loops or ensure they have an exit condition. Loops should have a clear termination point to prevent them from running indefinitely.
- Implement fallback functions to handle failed transactions gracefully. Provide informative error messages or revert the state changes to minimize disruption in case of out-of-gas errors.
contract SecureGasLimitation {
function consumeGas() public {
while (gasleft() > 10000) { // Continue loop until gas is low
// Do something with limited gas
}
revert("Out of gas"); // Handle out-of-gas gracefully
}
}
Unchecked External Calls: Trusting the Unknown
External contract calls can introduce vulnerabilities when not properly validated. Trusting external contracts without proper checks can lead to unintended behavior or even attacks.
- In this contract, the transferFunds function trusts the _receiver address without any validation beyond a null address check. A malicious contract could be deployed to receive the funds and then exploit a vulnerability within itself to steal them or disrupt the intended functionality.
Prevention:
- Always check the return value of external calls and handle any errors or exceptions. Ensure the called contract executed successfully and handle potential failures gracefully.
- Implement checks to verify the integrity of the receiving contract and its behavior. Consider using trusted contract registries or on-chain oracles to validate the reputation and expected behavior of external contracts before interacting with them.
contract SecureExternalCall {
function transferFunds(address _receiver, uint _amount) public {
require(_receiver != address(0));
(bool success, ) = _receiver.call{value: _amount}("");
require(success, "Transfer failed"); // Handle failed call
}
}
Typosquatting and Phishing Attacks
Solidity code and smart contract deployment are susceptible to typosquatting and phishing attacks. These social engineering techniques aim to trick users into interacting with malicious contracts that resemble legitimate ones.
Example:
- Typosquatting: An attacker might deploy a contract with a name very similar to a well-known and trusted contract, hoping users will accidentally interact with the malicious one instead.
- Phishing: Attackers may create fake websites or social media posts that appear to be from legitimate sources, encouraging users to interact with a malicious contract disguised as a real one.
Prevention:
- Double-check contract addresses before interacting with them. Verify that the address matches the one from the official source.
- Use code linters and static analysis tools to identify potential typos or naming conflicts in your code.
- Be cautious of unsolicited links or recommendations to interact with smart contracts. Only interact with contracts from trusted sources.
Conclusion: Solidity Security Vulnerabilities
Solidity offers immense potential for building secure and innovative DApps. However, developers must be aware of the security vulnerabilities that can arise and take proactive steps to mitigate them. By understanding these common pitfalls and adhering to secure coding practices, you can create robust smart contracts that are less susceptible to attacks and inspire trust within the blockchain ecosystem.
Remember, security is an ongoing process. Regularly audit your code and stay updated on the latest security threats and best practices to ensure your smart contracts remain secure in the ever-evolving blockchain landscape.
If you want to know about Smart contract testing, why wait!? Go check our recent article on Smart Contract Testing.
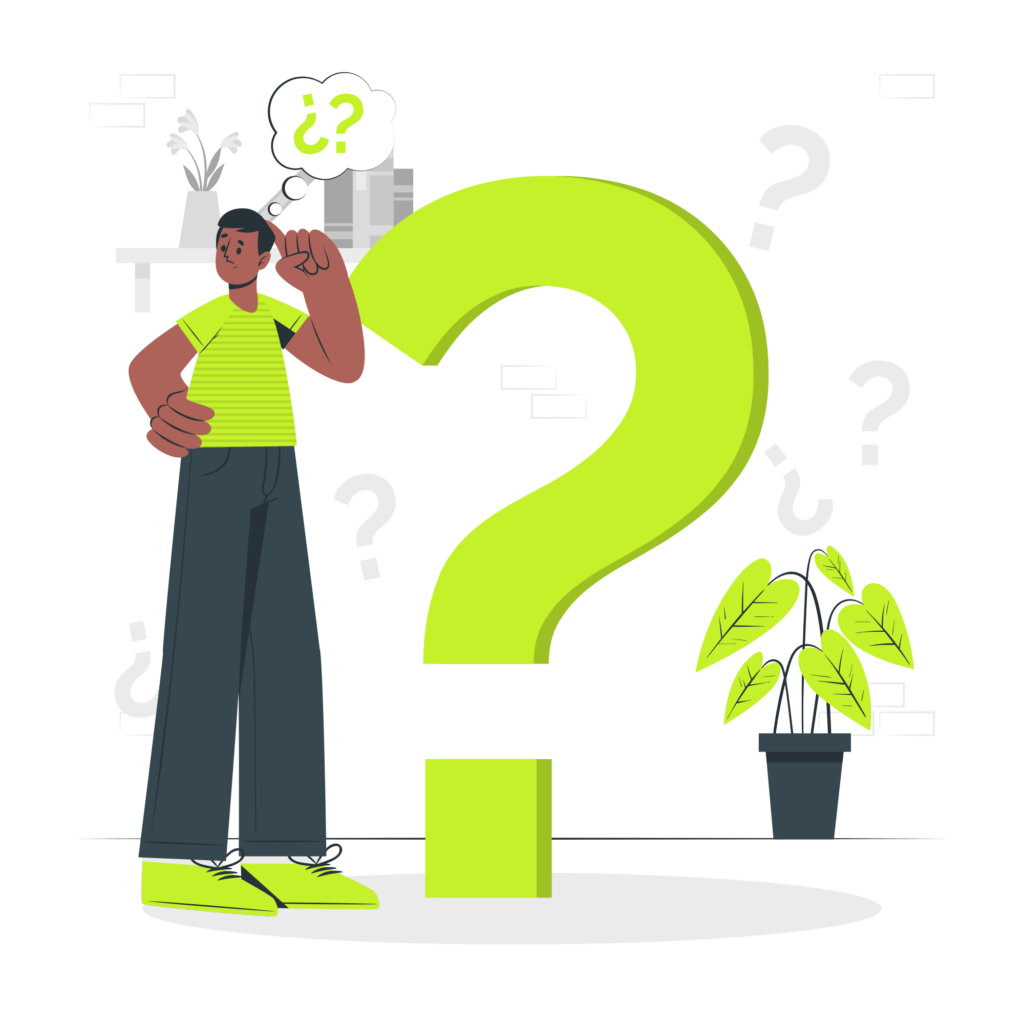
FAQs
What are common Solidity security vulnerabilities?
- Common vulnerabilities include reentrancy attacks, integer overflow and underflow, and improper access control.
How can I prevent reentrancy attacks in Solidity?
- Use the Checks-Effects-Interactions pattern and consider utilizing reentrancy guards to prevent such attacks.
What is integer overflow and how can it be avoided in Solidity?
- Integer overflow occurs when a number exceeds its storage limit. Use safe math libraries to prevent this issue.
Why is access control important in Solidity?
- Proper access control ensures that only authorized entities can execute certain functions, enhancing contract security.
How can I enhance the security of my Solidity smart contracts?
- Conduct thorough testing, perform audits, and adhere to best practices in smart contract development.
What are best practices for smart contract development?
- Best practices include code simplicity, regular audits, avoiding common pitfalls, and staying updated with the latest security trends.
How does blockchain technology influence smart contract security?
- Blockchain’s inherent features like immutability and transparency offer a robust foundation, but smart contract code must also be secure.
Can smart contract vulnerabilities affect the overall blockchain network?
- While they primarily impact the specific contracts, severe vulnerabilities can have broader implications on trust and network stability.
What resources are available for learning about Solidity security?
- Numerous resources like official Solidity documentation, security-focused blogs, and community forums provide valuable insights.
How do updates in Solidity versions impact security?
- New versions often address known vulnerabilities and introduce enhanced security features, so staying updated is crucial for security.