Websites that update and respond to your clicks feel like magic, but there’s a logical reason behind it. In this guide, we’ll explore the secret weapon of JavaScript – the Document Object Model, or DOM for short. It’s the key to making webpages dynamic and interactive, and you’ll be surprised at how easy it is to learn!
What is Document Object Model in JavaScript?
The Document Object Model (DOM) is a fundamental concept in web development. It serves as a tree-like representation of an HTML document, acting as a bridge between the webpage structure and programming languages like JavaScript. By interacting with the DOM, JavaScript can dynamically modify the content, style, and behavior of a webpage, creating a more interactive and engaging user experience.
Visualizing the DOM
Imagine the DOM as an upside-down tree. The entire document, including the HTML tags and their content, is represented by the root node at the top. Branches stem from the root, each representing an element or piece of content within the webpage. These elements can further branch out into child nodes, forming a hierarchical structure that mirrors the HTML code’s organization.
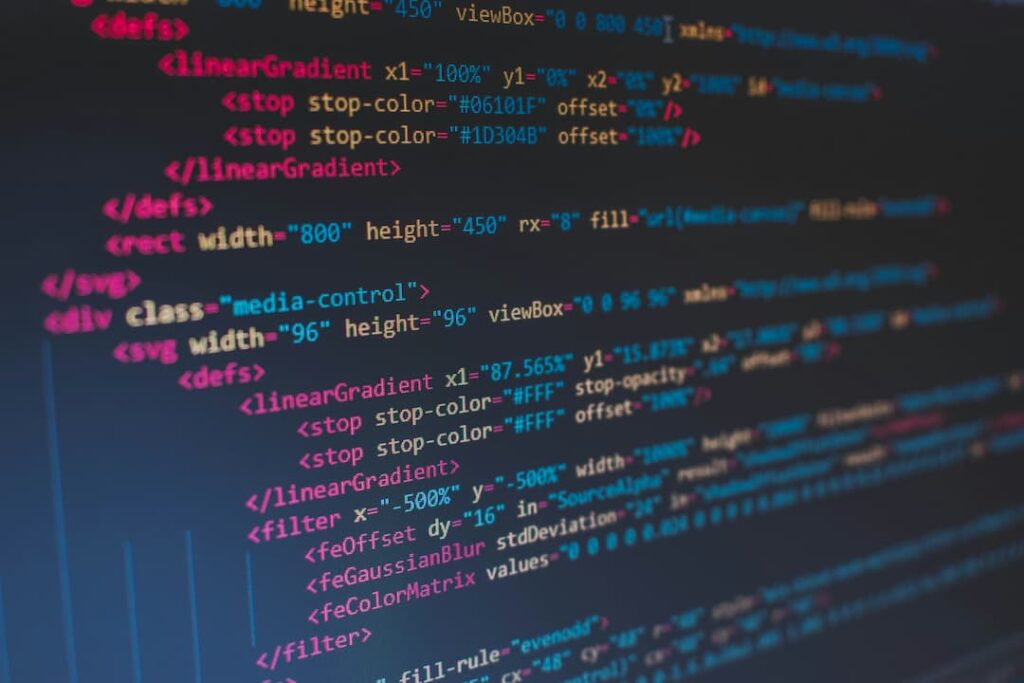
Elements, Nodes, and Attributes: Building Blocks of the DOM
The DOM consists of various elements, nodes, and attributes that work together to define the webpage’s structure and content:
- Elements: The fundamental building blocks of the DOM, corresponding to HTML tags like
<h1>
,<p>, <div>
, etc. Each element has properties (like its tag name, class, and ID) and content (the text or other elements it contains). - Nodes: More general units within the DOM tree, encompassing elements, text, comments, and processing instructions. Elements are a specific type of node.
- Attributes: Additional pieces of information attached to elements, providing details like an element’s style, class, or unique identifier (ID).
Common DOM Manipulation Techniques
Having explored the core concepts, let’s delve into some practical techniques for manipulating the DOM with JavaScript:
- Selecting Elements: JavaScript provides various methods to target specific elements in the DOM tree. Popular choices include:
document.getElementById(id)
: Retrieves an element by its unique ID (fastest for known IDs).document.querySelector(selector):
Selects the first element that matches a CSS selector (flexible for more complex targeting).document.getElementsByTagName(tagName):
Returns a collection of all elements with a specific tag name (useful for bulk operations).
- Modifying Content: Once you have a reference to an element, you can change its content using properties like:
element.textContent:
Sets or retrieves the text content within an element.element.innerHTML:
Sets or retrieves the entire HTML content of an element, including child elements (use with caution as it can introduce security risks).
- Adding and Removing Elements: Dynamically add or remove elements from the DOM using methods like:
parent.appendChild(child):
Inserts a child element as the last child of a parent element.parent.removeChild(child):
Removes a child element from its parent.
- Creating New Elements: JavaScript allows you to create new elements on the fly using document.createElement(tagName). You can then configure them with attributes and content before appending them to the DOM.
- Styling Elements: Modify the visual appearance of elements by manipulating their CSS styles using properties like:
element.style.backgroundColor = 'red';
element.style.color = 'white';
element.style.fontSize = '16px';
Advanced DOM Manipulation Techniques
While the fundamental techniques provide a solid foundation, the DOM offers more advanced capabilities for complex web interactions:
- Event Handling: The DOM allows you to attach event listeners to elements. These listeners watch for specific user interactions (clicks, key presses, mouse movements) and trigger JavaScript code in response. This forms the core of user interactivity on webpages.
- Asynchronous Operations: The DOM can be used in conjunction with techniques like AJAX (Asynchronous JavaScript and XML) to fetch data from servers without reloading the entire page. This enables features like live chat updates or dynamic content loading based on user actions.
- DOM Traversal: Navigate the DOM tree efficiently using methods like:
parentNode:
Access the parent element of a node.childNodes:
Retrieve a collection of a node’s child nodes.nextSibling:
Get the next sibling node of a node.
- DOM Manipulation Libraries: Popular JavaScript libraries like jQuery offer a simplified and performant way to interact with the DOM, providing abstraction over the core methods and additional features.
DOM Manipulation in Action
Let’s consider a scenario where you have a button on your webpage that, when clicked, changes the background color of a specific element. Here’s how you might achieve this using JavaScript and DOM manipulation:
const button = document.getElementById('change-color-button');
const elementToChange = document.getElementById('color-changeable-element');
button.addEventListener('click', function() {
elementToChange.style.backgroundColor = 'lightblue';
});
In this example, the button
and elementToChang
e variables store references to the corresponding DOM elements using getElementById. An event listener is attached to the button, and when clicked, it sets the backgroundColor style property of the target element to ‘lightblue’.
DOM Applications in Web Development
The DOM is a fundamental building block for various web development practices:
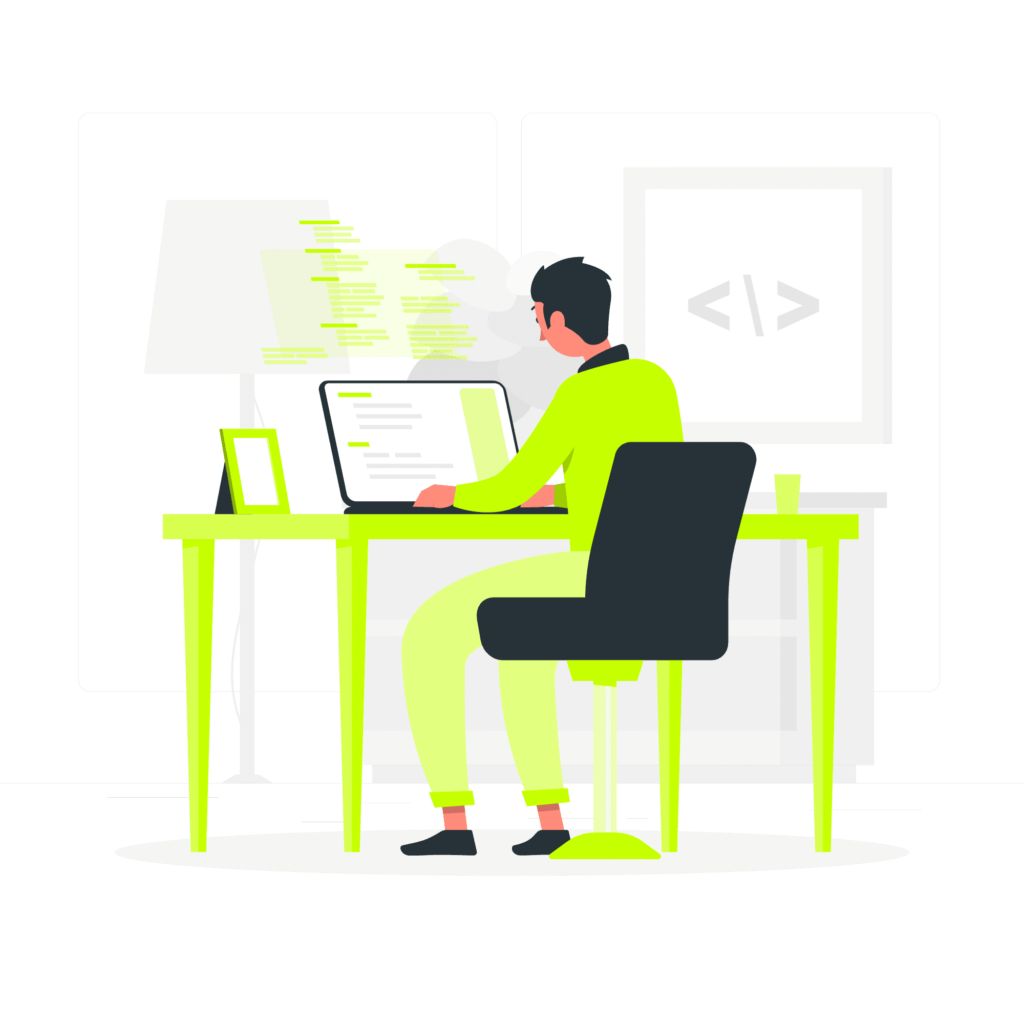
- Single-Page Applications (SPAs): SPAs rely heavily on DOM manipulation to create a seamless user experience where the page appears static but content updates dynamically.
- Web Components: Reusable UI components can be built using the DOM, allowing you to create modular and maintainable web applications.
- Accessibility: DOM manipulation plays a crucial role in making webpages accessible to users with disabilities by dynamically adapting content and presentation based on user preferences or assistive technologies.
The Benefits of a Dynamic Web Experience
By leveraging the DOM, JavaScript unlocks a plethora of benefits that make webpages more engaging and user-friendly:
- Improved User Experience (UX): Dynamic functionalities like real-time updates, responsive interfaces, and interactive elements enhance user engagement and create a more intuitive browsing experience.
- Enhanced Accessibility: By dynamically adapting content and presentation based on user preferences or device capabilities, the DOM helps cater to a wider audience and ensures accessibility for users with disabilities.
- Reduced Page Reloads: DOM manipulation allows you to update specific parts of a webpage without reloading the entire page. This leads to a faster and more seamless user experience.
- Richer and More Engaging Content: The capabilities of the DOM enable the creation of dynamic content like image carousels, animated charts, and interactive games, adding depth and interest to your webpages.
Conclusion
The Document Object Model (DOM) is more than just a technical concept; it’s a paradigm shift in web development. It empowers developers to move beyond static webpages and create dynamic, responsive, and user-centric experiences. By understanding and mastering DOM manipulation, you unlock a vast array of tools to bring your web creations to life and connect with your audience in a more impactful way.
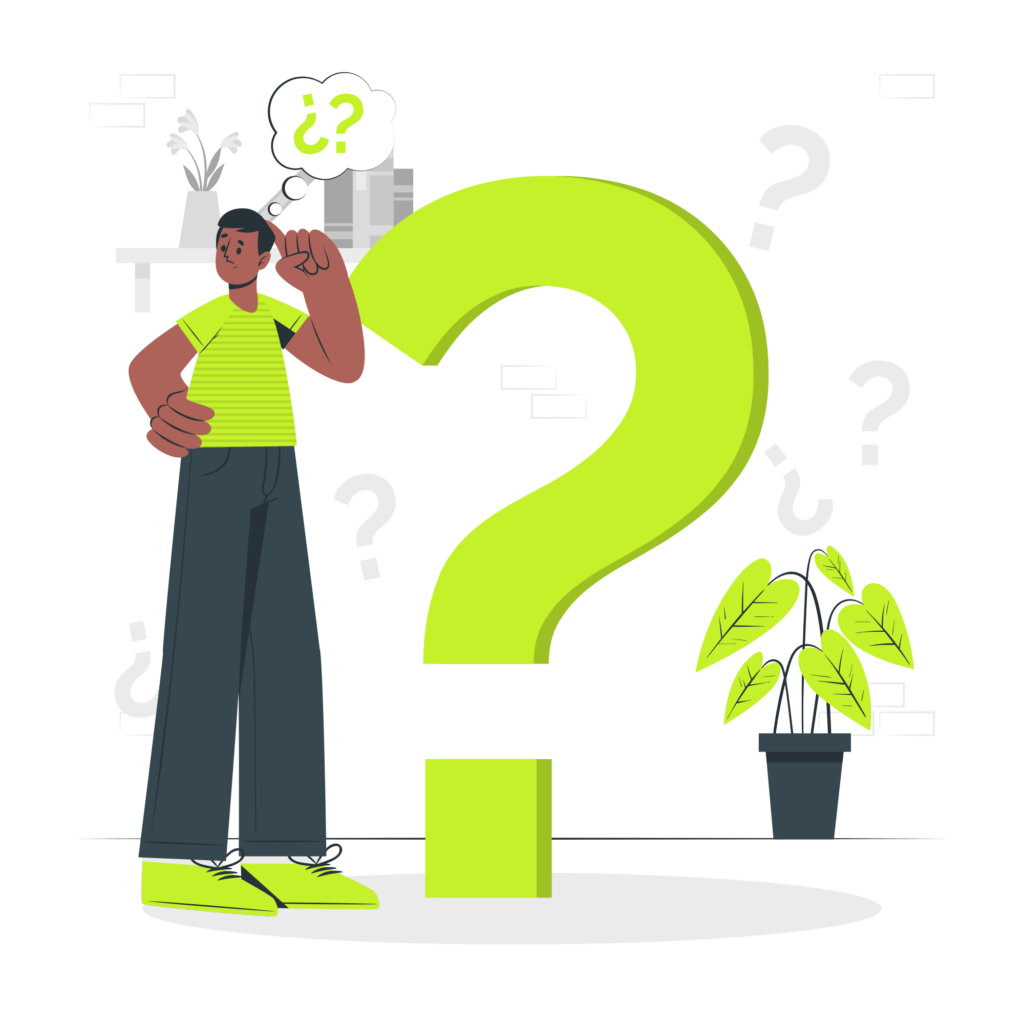
FAQs:
What is the Document Object Model (DOM) in JavaScript?
- The DOM is a programming interface that allows scripts to update the content, structure, and style of a webpage dynamically.
How does the DOM work with JavaScript?
- JavaScript uses the DOM to interact with and modify the webpage, allowing developers to respond to user actions and update the page content dynamically.
What are some common methods used in DOM manipulation?
- Common DOM methods include
getElementById()
,appendChild()
,removeChild()
, andaddEventListener()
.
Why is understanding the DOM important for web developers?
- Mastery of the DOM enables developers to create interactive, dynamic web applications that improve user experience.
Can you give an example of a simple DOM manipulation in JavaScript?
- A simple example is changing the text of an HTML element using
document.getElementById('id').innerText = 'New Text';
.
What are the basics of web development?
- Web development involves building and maintaining websites, incorporating aspects like web design, web publishing, web programming, and database management.
How do you add event listeners in JavaScript?
- Event listeners are added using the
addEventListener()
method, specifying the event to listen for and the callback function to execute.
What is the difference between HTML and the DOM?
- HTML is the markup language that defines the structure of web pages, whereas the DOM is an object-oriented representation of the web page that scripts can manipulate.
What resources can help further understand the DOM and JavaScript?
- Resources include coding bootcamps, MDN Web Docs, JavaScript tutorials on platforms like freeCodeCamp, and interactive coding sites such as Codecademy and Udemy.