TL;DR
- JavaScript iterators return a sequence of values, which may contain duplicates.
- You can use a
Set
to easily get unique values by filtering out duplicates. - A
Map
can be used if you need to track the number of occurrences of each unique value. - Using
Set
andMap
simplifies code and improves performance when working with large datasets. - Extracting unique values from an iterator ensures cleaner, more efficient code.
When working with iterators in JavaScript, it’s not uncommon to encounter situations where you need to extract unique values. Whether you’re handling user input, processing data streams, or simply working with large datasets, getting unique values from an iterator can simplify your code and enhance its performance. In this article, we’ll dive into the techniques you can use to get unique values of an iterator in JavaScript, providing you with practical and straightforward solutions.
Understanding Iterators in JavaScript
Before we dive into how to get unique values from an iterator, it’s essential to first understand what an iterator is in JavaScript. An iterator is an object that defines a sequence and potentially a return value upon iteration. It is typically used in for...of
loops, Map
, Set
, and other iterable structures. When you work with an iterator, it returns an object with a value
and a done
property. The done
property indicates if the iterator has finished iterating, while the value
property holds the current element.
In many cases, iterators can produce duplicate values, which may not be desirable, especially when dealing with large datasets. This is where the ability to extract unique values becomes crucial.
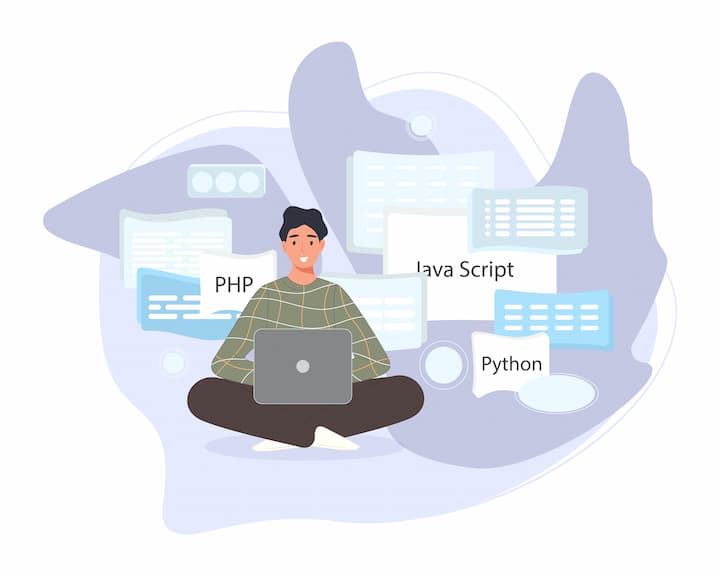
Getting Unique Values from an Iterator in JavaScript
Using a Set to Filter Out Duplicates
One of the easiest ways to get unique values from an iterator is by using a Set
. A Set
is a collection of values that automatically removes duplicates. You can iterate through the values in the iterator, add them to a Set
, and then convert the set back into an array if necessary.
Here’s how you can achieve that:
<code>function getUniqueValues(iterator) {
const uniqueValues = new Set();
for (const value of iterator) {
uniqueValues.add(value);
}
return [...uniqueValues];
}
const data = [1, 2, 3, 3, 4, 5, 5, 6];
const iterator = data[Symbol.iterator]();
console.log(getUniqueValues(iterator)); // Output: [1, 2, 3, 4, 5, 6]
</code>
In this example, the Set
automatically removes duplicate values as the iterator goes through the data
array. The result is an array of unique values.
Using a Map to Track Occurrences
If you need to keep track of how many times each value appears in the iterator, you can use a Map
. A Map
allows you to store the count of each unique value, which can be useful in certain situations.
Here’s an example of how to use a Map
to get unique values and their counts:
<code>function getUniqueWithCount(iterator) {
const countMap = new Map();
for (const value of iterator) {
countMap.set(value, (countMap.get(value) || 0) + 1);
}
return Array.from(countMap.keys());
}
const data = [1, 2, 2, 3, 3, 3, 4, 4, 5];
const iterator = data[Symbol.iterator]();
console.log(getUniqueWithCount(iterator)); // Output: [1, 2, 3, 4, 5]
</code>
In this case, we use a Map
to count the occurrences of each value. We then return just the keys from the map, which represent the unique values.
Other Methods to Get Unique Values from an Iterator
While Set
and Map
are often the go-to methods for extracting unique values, there are other techniques you can explore, depending on your specific needs. For instance, if you’re working with more complex data types or have specific filtering criteria, you could use custom logic within a for...of
loop. However, using a Set
is generally the most efficient and straightforward approach for most scenarios.
Conclusion: Simplify Your Code
In JavaScript, getting unique values from an iterator is a simple and effective way to ensure that your data is free of duplicates. By leveraging Set
or Map
, you can easily filter out duplicate values or even track their occurrences. No matter the complexity of your data, these techniques will help you write cleaner, more efficient code. So, next time you need to get unique values of an iterator, remember the power of these simple yet powerful JavaScript features.
FAQs
What is an iterator in JavaScript?
An iterator is an object that provides access to the elements of an iterable object (like arrays, maps, or sets) in a sequence.
How does a Set
help get unique values?
A Set
automatically removes duplicate values when you add them, ensuring that only unique values are retained.
Can I use Map
to get unique values?
Yes, while a Map
tracks the frequency of values, you can extract just the keys, which will represent the unique values in the iterator.
Are there performance considerations when using Set
?
Using a Set
is generally efficient for removing duplicates. However, performance may vary depending on the size of the dataset and the operations you perform.
What other methods can be used to get unique values from an iterator?
Apart from using Set
and Map
, you could use custom logic in loops or other data structures to filter unique values, but Set
is usually the most efficient.