Web3.js open up the huge power of Ethereum blockchain for developers. This JavaScript library let’s you talk with Ethereum, allowing you to interact with Ethereum’s decentralized network from your web applications. With Web3.js, you can build new applications like Decentralized Exchanges (DEXs), Non-Fungible Token (NFT) marketplaces, and other blockchain-powered tools.
In this article, we will explore Web3.js, providing a complete guide on how to connect to Ethereum with Web3.js and explore its core functionalities. We’ll cover various critical aspects like,
- Demystifying Web3.js: Understanding its role in the Ethereum ecosystem.
- Setting Up Your Development Environment: Preparing your tools to work with Web3.js.
- Connecting to an Ethereum Node: Establishing communication with the blockchain.
- Interacting with Ethereum Accounts: Managing user accounts and interacting with the network.
- Working with Smart Contracts: Reading from and writing data to smart contracts.
- Sending Transactions: Initiating actions on the Ethereum network.
- Exploring Advanced Features: Unveiling additional capabilities of Web3.js.
- Best Practices and Security Considerations: Building secure and robust blockchain applications.
Demystifying Web3.js
Imagine a world where your web applications can interact with a global, decentralized network. This is precisely what Web3.js enables. It’s a JavaScript library that provides a high-level abstraction over the Ethereum protocol. This means it simplifies complex blockchain interactions, allowing developers to focus on building innovative applications without getting bogged down in the underlying technical details.
Here’s a breakdown of how Web3.js facilitates communication.
- JSON-RPC: Web3.js utilizes JSON-RPC (Remote Procedure Call) to communicate with Ethereum nodes. These nodes act as computers on the network, storing and validating blockchain data. By sending JSON-formatted requests and receiving responses, Web3.js allows you to interact with the Ethereum network.
- Functionalities: Web3.js offers a comprehensive set of functionalities. You can retrieve data about the blockchain, manage user accounts, interact with smart contracts, and send transactions. This empowers developers to build a wide range of decentralized applications (dApps).
Setting Up Your Development Environment
Before embarking on your Web3.js journey, ensure you have the necessary tools installed:
- Node.js and npm (Node Package Manager): Node.js is a JavaScript runtime environment that allows you to run JavaScript code outside of a web browser. npm, included with Node.js, helps manage dependencies (external libraries) required by your project. You can download and install Node.js from the official website (https://nodejs.org/en).
- Web3.js Library: Once Node.js is set up, open your terminal and use npm to install Web3.js:
npm install web3
- Ethereum Node Provider: To interact with the Ethereum network, you’ll need a connection to an Ethereum node. There are two primary options:
- Run your own Ethereum node: This gives you complete control but requires significant technical expertise and computational resources. Tools like Geth or Parity can be used for this purpose.
- Use a public Ethereum node provider: Several providers offer publicly accessible Ethereum nodes. These are convenient options, especially for development purposes. Popular providers include Infura, Alchemy, and QuickNode. Each provider has its own setup instructions and pricing plans.
Choosing an Editor/IDE: While not strictly mandatory, having a code editor or Integrated Development Environment (IDE) can significantly enhance your development experience. Popular options for working with JavaScript include Visual Studio Code, Atom, and WebStorm. These editors offer features like syntax highlighting, code completion, and debugging tools, making development more efficient.
Connecting to an Ethereum Node
With your environment set up, let’s establish a connection to an Ethereum node using Web3.js. Here’s a basic example assuming you’re using a public node provider like Infura:
// **WARNING: Replace "YOUR_INFURA_URL" with your actual Infura node URL but keep it out of production code.**
const infuraUrl = "YOUR_INFURA_URL";
const provider = new Web3.providers.HttpProvider(infuraUrl);
const web3 = new Web3(provider);
// Check connection
web3.eth.getBlockNumber().then((blockNumber) => {
console.log("Connected to Ethereum network. Current block number:", blockNumber);
}).catch((error) => {
console.error("Error connecting to Ethereum node:", error);
});
Explanation:
We import the Web3 library using require(‘web3’). Infura is used here as an example public node provider. Remember to replace “YOUR_INFURA_URL” with your actual Infura node URL, but be sure to keep it out of production code. There are other reputable providers available, so feel free to explore options that best suit your needs.
Interacting with Ethereum Accounts
Web3.js empowers you to manage user accounts on the Ethereum network. Here’s how you can interact with accounts,
- Creating Accounts: You can generate new Ethereum accounts using Web3.js. However, it’s important to note that these accounts are for development purposes only. For production environments, users will typically manage their own accounts through web wallets or hardware wallets.
const account = web3.eth.accounts.create();
console.log("New account address:", account.address);
console.log("Private key (keep secret!):", account.privateKey);
- Obtaining Account Information: Web3.js allows you to retrieve information about existing accounts, such as their balance.
const address = "0x..."; // Replace with an existing account address
web3.eth.getBalance(address).then((balance) => {
console.log("Balance of account:", web3.utils.fromWei(balance, 'ether'), "ETH");
});
- Sending Transactions: To interact with the Ethereum network, you’ll need to send transactions. These transactions typically involve transferring Ether (ETH), the native currency of Ethereum, or interacting with smart contracts. Sending transactions requires a private key, which should be kept confidential as it grants access to the associated account’s funds.
const fromAddress = "0x..."; // Account sending the transaction (with private key)
const toAddress = "0x..."; // Recipient of the transaction
const value = web3.utils.toWei("1", 'ether'); // Amount of ETH to send
web3.eth.sendTransaction({ from: fromAddress, to: toAddress, value: value })
.then((transactionHash) => {
console.log("Transaction sent! Hash:", transactionHash);
}).catch((error) => {
console.error("Error sending transaction:", error);
});
Important Note: Sending transactions consumes gas, a unit used to measure the computational effort required for processing the transaction on the Ethereum network. The gas price determines the fee paid to miners for including the transaction in a block.
Working with Smart Contracts
Smart contracts are self-executing programs deployed on the Ethereum blockchain. They define a set of rules and can hold and manage assets. Web3.js allows you to interact with smart contracts by:
- Deploying Contracts: You can deploy your own smart contracts to the Ethereum network. This involves compiling the smart contract code (often written in Solidity) and submitting a transaction to deploy it on the blockchain.
- Interacting with Deployed Contracts: Once a smart contract is deployed, you can interact with its functions. Web3.js provides methods for:
- Reading Contract Data: Retrieving information stored within a smart contract.
- Writing to Contracts: Sending transactions to invoke functions within a smart contract, potentially modifying its state.
Here’s a simplified example of reading data from a deployed smart contract.
const contractAddress = "0x..."; // Address of the deployed smart contract
const contractABI = [ ]; // Array defining the contract's functions and variables // Replace with actual ABI
const contract = new web3.eth.Contract(contractABI, contractAddress);
contract.methods.getValue().call() // Calls the "getValue" function of the contract
.then((value) => {
console.log("Value stored in contract:", value);
}).catch((error) => {
console.error("Error reading from contract:", error);
});
Understanding ABIs: The contractABI variable in the example above represents the Application Binary Interface (ABI) of the smart contract. The ABI defines the functions and variables available within the contract, enabling Web3.js to interact with it correctly.
Sending Transactions
As mentioned earlier, sending transactions is a crucial aspect of interacting with the Ethereum network. Let’s delve deeper into this concept:
- Transaction Parameters: When sending a transaction, you need to specify various parameters:
- from: The address of the account sending the transaction (requires a private key).
- to: The recipient address (can be a smart contract or another user account).
- value: The amount of Ether (ETH) to be transferred (if applicable).
- gas: The maximum amount of gas the sender is willing to spend for the transaction to be processed.
- gasPrice: The price the sender is willing to pay per unit of gas (determines transaction fee).
- data: Optional data to be included in the transaction, often used when interacting with smart contracts (e.g., function call arguments).
- Estimating Gas: Estimating the required gas for a transaction is essential. Overestimating gas leads to wasted fees, while underestimating can result in the transaction failing. Web3.js offers methods like estimateGas to help estimate gas usage for specific transactions.
- Sending Transactions with Signed Messages: Sending transactions directly with private keys can be insecure. A more secure approach is to use signed messages. Web3.js provides methods for signing transactions with a private key and then broadcasting the signed message to the network. This approach decouples the signing process from the actual transaction sending, enhancing security.
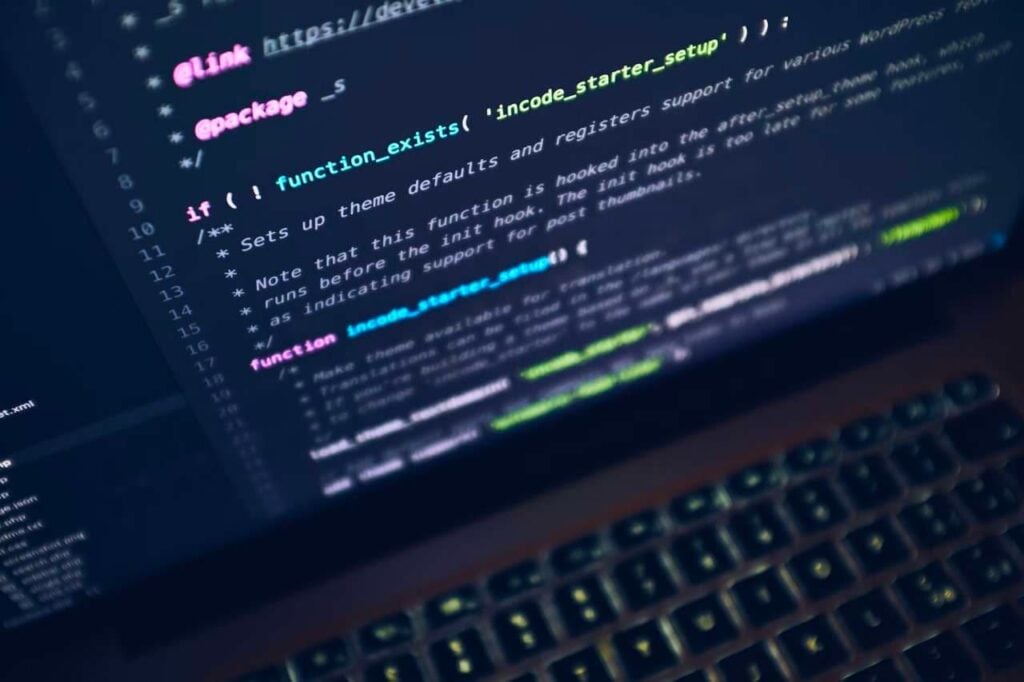
Exploring Advanced Features
Web3.js offers a rich set of functionalities beyond the basics covered so far. Here’s a glimpse into some advanced features:
- Filters and Events: Filters allow you to monitor specific events happening on the Ethereum network, such as new block additions or transactions involving a particular address. This facilitates building real-time applications that react to network activity.
- Web3 Providers: Web3.js supports various provider types, allowing you to connect to different types of Ethereum nodes. For instance, you can connect to private Ethereum networks using a custom provider implementation.
- Web3 IPC and WebSockets: Web3.js can interact with Ethereum nodes using IPC (Inter-Process Communication) or WebSockets for faster, real-time communication compared to the standard HTTP provider.
Best Practices and Security Considerations
Building secure and robust blockchain applications is paramount. Here are some best practices to follow when using Web3.js:
- Securely Manage Private Keys: Never expose private keys in your code or share them with anyone. Consider using hardware wallets to store private keys securely.
- Input Validation: Always validate user input before including it in transactions. This helps prevent malicious attacks like transaction injection.
- Error Handling: Implement robust error handling to gracefully handle potential issues that may arise during interactions with the Ethereum network.
- Stay Updated: Keep Web3.js and your other dependencies updated to benefit from bug fixes and security improvements.
- Test Thoroughly: Thoroughly test your application to ensure its functionality and identify potential vulnerabilities before deployment.
Conclusion: How to Connect to Ethereum with Web3.js
Web3.js is a door to the huge power of the Ethereum chain of blocks. By knowing its main uses and doing things the right way, you can use Web3.js to make new and safe decentralized applications. This article gave you a basic idea of Web3.js and how to connect to Ethereum with Web3.js. As you dig in more, look at the wide range of guides and help papers online to grow your abilities and make cutting-edge apps that use blocks of data.
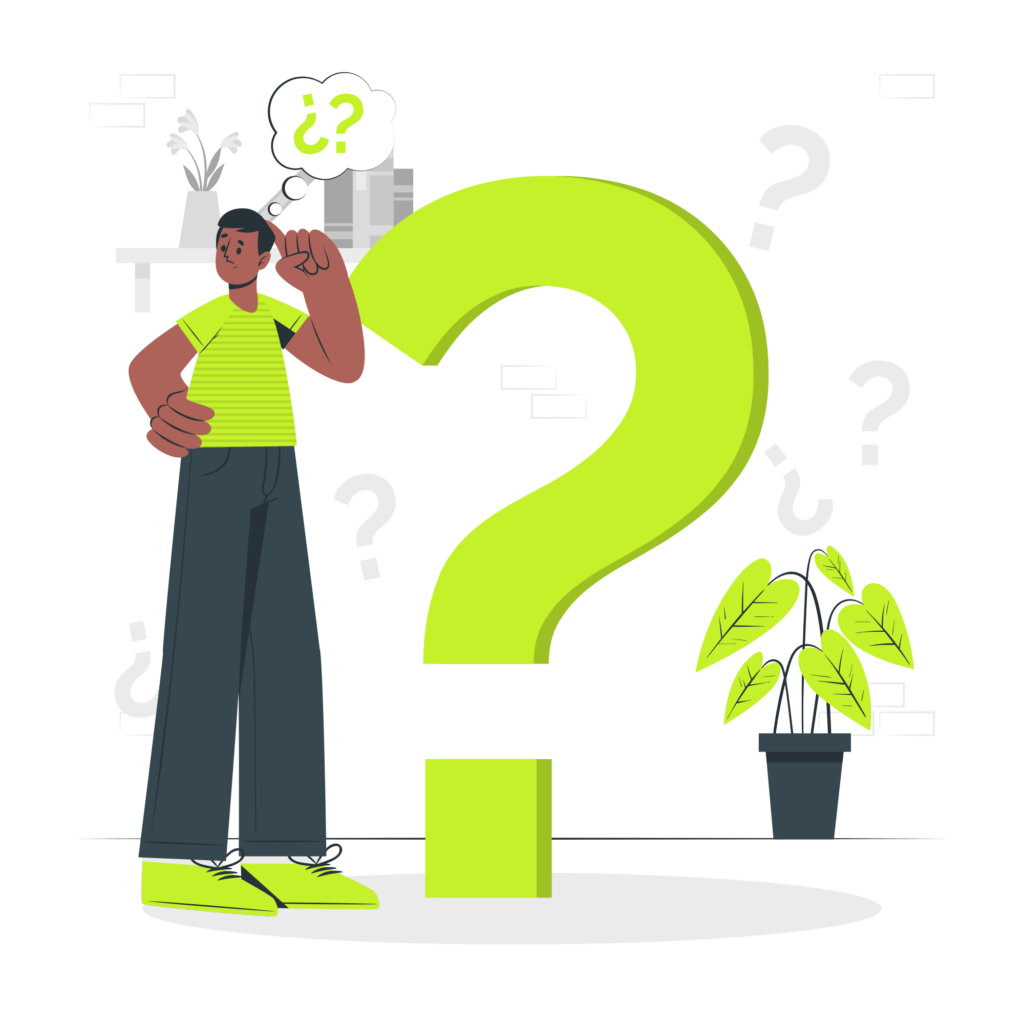
FAQs
What is Web3.js and why is it important for Ethereum development?
- Web3.js is a JavaScript library that allows you to interact with the Ethereum blockchain, enabling the development of client-side applications that connect to Ethereum nodes.
How do I set up Web3.js to connect with Ethereum?
- To connect with Ethereum using Web3.js, you need to install the library, set up a connection to an Ethereum node, and use the Web3.js API to interact with the blockchain.
What are the prerequisites for using Web3.js in a project?
- Understanding JavaScript and basic blockchain concepts are essential. Additionally, having Node.js installed is crucial for utilizing Web3.js in your project.
Can Web3.js be used for both frontend and backend applications?
- Yes, Web3.js can be integrated into both frontend and backend applications, providing a versatile tool for developers to interact with the Ethereum blockchain.
What are some common use cases of Web3.js in Ethereum development?
- Common use cases include creating wallets, sending transactions, interacting with smart contracts, and building decentralized applications (dApps).
What is Ethereum and how does it differ from other blockchains?
- Ethereum is a decentralized platform that enables the creation of smart contracts and decentralized applications (dApps), distinguishing itself with its Turing-complete virtual machine, the Ethereum Virtual Machine (EVM).
What are smart contracts and how do they work on Ethereum?
- Smart contracts are self-executing contracts with the terms directly written into code. On Ethereum, they run on the EVM and automatically enforce the terms of the contract.
How does blockchain technology impact web development?
- Blockchain technology introduces new possibilities in web development, such as creating more secure, decentralized applications and changing how data is stored and transacted on the web.
What is the significance of blockchain connectivity in web applications?
- Blockchain connectivity allows web applications to interact with blockchain networks, enabling features like cryptocurrency transactions, smart contract interactions, and decentralized data storage.
What resources are recommended for beginners to learn about Web3.js and Ethereum?
- Beginners should explore official Web3.js documentation, Ethereum development tutorials, online courses, and community forums to gain a comprehensive understanding of these technologies.