Web3.js, a popular JavaScript library, empowers developers to build decentralized applications (dApps) that interact with blockchains. But how do these dApps actually connect to the underlying network and perform actions like sending transactions or reading data? This important role falls to Web3.js providers.
This article discuss the world of Web3.js providers, exploring their purpose, different types, functionalities, and considerations for choosing the right one for your dApp development needs.
The Bridge Between dApps and Blockchains
Imagine a dApp as a user interface for interacting with a blockchain network. While the dApp provides the user experience, it doesn’t have direct access to the network itself. This is where Web3.js providers come in. They act as a bridge, facilitating secure and efficient communication between the dApp and the blockchain.
Here’s how Web3.js providers enable dApp functionality:
- Network Communication: Providers establish a connection between the dApp and a blockchain node, which holds a copy of the entire blockchain ledger. This allows the dApp to send requests and receive responses from the network.
- Transaction Management: Users interact with dApps to initiate transactions, like sending cryptocurrency or calling smart contract functions. Providers handle the user’s private key securely and broadcast signed transactions to the network for processing.
- Data Access: dApps often need to retrieve data from the blockchain, such as transaction history or current account balances. Providers offer methods to query the blockchain and fetch this information for the dApp to utilize.
Without Web3.js providers, dApps would be isolated islands, unable to interact with the very networks they’re built upon.
What are the Different Types of Providers?
Web3.js offers flexibility in choosing the right provider for your dApp’s specific requirements. Here’s a breakdown of the most common types:
- HTTP Provider: The simplest and most widely used, this provider communicates with a remote Ethereum node via HTTP requests and responses. It’s suitable for basic dApp functionalities and development environments.
const Web3 = require('web3');
const provider = new Web3.providers.HttpProvider('https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID');
const web3 = new Web3(provider);
web3.eth.getBlockNumber().then(console.log);
This code snippet demonstrates creating a Web3 instance using an HTTP provider from Infura. It then retrieves the latest block number using the web3.eth.getBlockNumber() method.
- WebSocket Provider: This provider establishes a persistent, real-time connection with the node using WebSockets. This allows for faster, bi-directional communication, ideal for dApps requiring constant updates like live markets or chat applications.
const Web3 = require('web3');
const provider = new Web3.providers.WebsocketProvider('wss://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID');
const web3 = new Web3(provider);
web3.eth.subscribe('newBlockHeaders', (error, blockHeader) => {
if (!error) {
console.log(blockHeader);
}
});
This code snippet creates a Web3 instance using a WebSocket provider. It then subscribes to the newBlockHeaders event to receive notifications whenever a new block is added to the blockchain.
- IPC Provider (Node.js Only): This provider is exclusive to Node.js environments and utilizes Inter-Process Communication (IPC) for direct communication with a locally running Ethereum node. It offers the fastest performance but is limited to Node.js development.
const Web3 = require('web3');
// Assuming you have a locally running Ethereum node
const provider = new Web3.providers.IpcProvider('/path/to/geth.ipc'); // Replace with your IPC path
const web3 = new Web3(provider);
web3.eth.getBlockNumber().then(console.log);
This code snippet demonstrates creating a Web3 instance using an IPC provider. It connects to a locally running Ethereum node using the IPC socket path (/path/to/geth.ipc). Replace this path with the actual location of your geth.ipc file. It then retrieves the latest block number using the web3.eth.getBlockNumber() method.
- Third-Party Providers (EIP 1193 Compliant): These providers are hosted by external services like Infura, Alchemy, or QuickNode. They offer various features like rate limiting, caching, and infrastructure management, simplifying development and scaling for complex dApps.
Choosing the right provider depends on factors like the dApp’s complexity, real-time data needs, development environment, and infrastructure preferences.
What Functionalities Do Providers Offer?
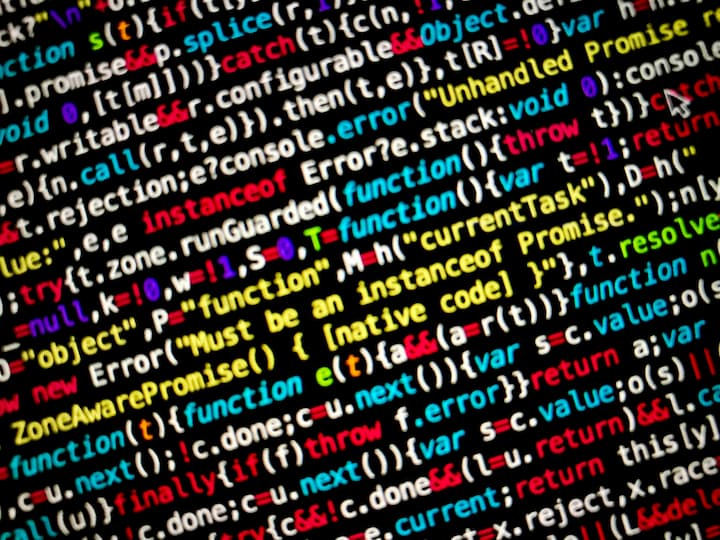
Web3.js providers equip developers with a suite of tools to create dynamic and powerful decentralized applications (dApps). They facilitate account management by connecting to user wallets, enabling access to public addresses, balances, and secure transaction signing. These providers also empower dApps to interact with smart contract interaction, allowing for the execution of contract functions and retrieval of results. Additionally, they provide mechanisms to extract specific data from the blockchain, such as blockchain data retrieval, which includes transaction histories, block information, and smart contract event logs, crucial for dApp functionality and user interfaces. Moreover, providers support event monitoring, enabling dApps to respond to blockchain events in real-time, thereby enhancing user engagement and interaction.
Understanding these functionalities is crucial for developers to effectively utilize Web3.js providers and build powerful dApps.
What Advanced Considerations Should Developers Keep in Mind?
While Web3.js providers offer a solid foundation for dApp development, there are additional considerations for advanced users:
- Security: Since providers handle private keys for transaction signing, security is paramount. Choose a reputable provider with robust security measures and best practices for key management.
- Reliability: dApps rely on the provider’s uptime and performance. Consider factors like infrastructure redundancy, geographical distribution, and historical uptime records when making a choice.
- Scalability: As dApp usage grows, the provider needs to scale to accommodate increased traffic and transaction volume. Look for providers with infrastructure designed for handling high loads.
- Cost: Some providers offer free tiers with limited features, while others have paid plans with higher transaction quotas, faster speeds, and additional functionalities. Evaluate your dApp’s needs and choose a cost-effective option.
- Feature Set: Different providers offer varying functionalities beyond core functionalities. Look for providers with features that align with your dApp’s specific requirements, such as built-in IPFS gateways, oracle integration, or support for specific blockchains.
- Community and Support: A strong community and active support can be invaluable for developers. Choose a provider with well-documented APIs, tutorials, and responsive support channels to address any challenges you may encounter.
By carefully considering these factors, developers can ensure they choose a Web3.js provider that optimizes their dApp’s performance, security, and scalability.
How are Web3.js Providers Expanding Their Multi-chain Capabilities?
While this article has primarily focused on Ethereum, it’s important to note that Web3.js and compatible providers are not limited to a single blockchain. As the blockchain ecosystem expands, so does the support for alternative networks.
Here’s a glimpse into the future of Web3.js providers:
- Multichain Support: Many providers are extending their support to include popular blockchains like BNB Chain (formerly Binance Smart Chain), Polygon, Avalanche, and Solana. This opens doors for developers to build dApps that interact with a wider range of blockchain ecosystems.
- Interoperability Solutions: Emerging solutions like LayerZero and Axelar are enabling seamless communication between different blockchains. Web3.js providers might integrate with these solutions in the future, allowing dApps to leverage functionalities across multiple chains.
Staying updated on the evolving landscape of Web3.js providers and their multichain capabilities will be crucial for developers building future-proof dApps.
What is the Future Like for Web3.js Providers?
Web3.js providers are the unsung heroes of the dApp development world. They empower developers to bridge the gap between user interfaces and the complex machinery of blockchains. Understanding their functionalities and choosing the right provider are essential steps in building secure, performant, and scalable dApps.
As the blockchain landscape continues to evolve, Web3.js providers will play a critical role in fostering innovation and mainstream adoption of decentralized applications. With an understanding of the concepts presented in this article, developers can harness the power of Web3.js providers and contribute to shaping the future of a decentralized web.
Here are some additional resources for developers to delve deeper into Web3.js providers:
- Web3.js Documentation: https://docs.web3js.org/guides/web3_providers_guide/
- Infura Documentation: https://docs.infura.io/api
- Alchemy Documentation: https://docs.alchemy.com/
- QuickNode Documentation: https://www.quicknode.com/docs/welcome
- Ethereum Stack Exchange: https://ethereum.stackexchange.com/
Remember, the world of Web3.js providers is vast and ever-changing. By staying informed and exploring the available options, developers can unlock the full potential of blockchain interaction and build the next generation of decentralized applications.
Conclusion: Web3.js Providers
Web3.js providers are the essential bridge between user interfaces and the complex world of blockchains. Understanding their functionalities, choosing the right provider, and exploring advanced features empower developers to build secure, performant, and innovative dApps.
As the blockchain ecosystem continues to evolve, Web3.js providers will play a critical role in shaping the future of decentralized applications. By staying informed about the latest developments and best practices, developers can leverage the power of Web3.js providers to unlock the full potential of blockchain technology and contribute to a more decentralized future.
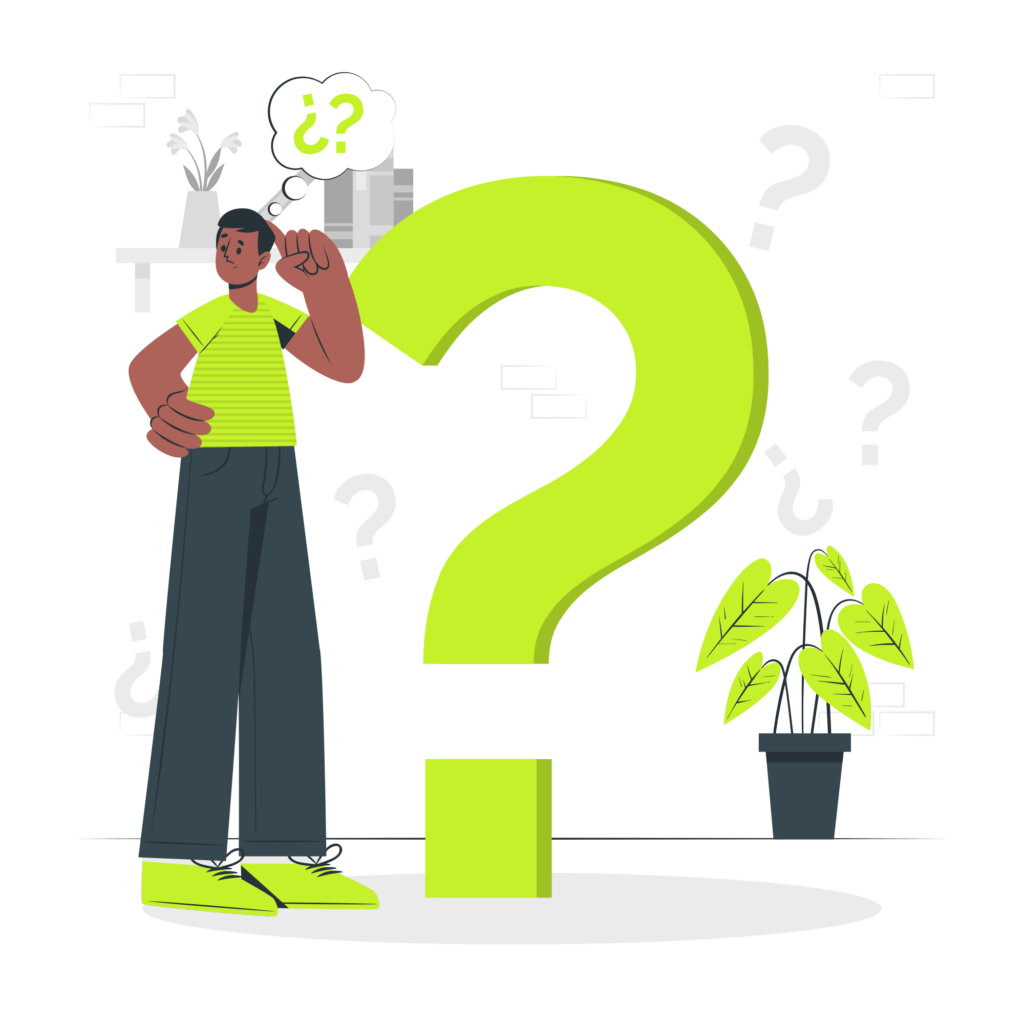
FAQs
What are Web3.js Providers?
- Web3.js Providers are essential components in Web3.js that allow your application to communicate with an Ethereum node, enabling interactions with the blockchain.
How do Web3.js Providers work?
- They act as a communication bridge between your application and the Ethereum blockchain, transmitting data and requests back and forth.
Why are Web3.js Providers important?
- They enable your application to read data from and write transactions to the Ethereum blockchain, facilitating various decentralized actions.
Can you change Web3.js Providers in an application?
- Yes, developers can switch between different providers in an application to connect to various Ethereum nodes or networks.
What are some examples of Web3.js Providers?
- Examples include HTTP, WebSocket, and IPC providers, each supporting different types of connections to Ethereum nodes.
What is Web3.js?
- Web3.js is a JavaScript library that allows you to interact with the Ethereum blockchain, enabling the creation of decentralized applications.
What is the Ethereum blockchain?
- Ethereum is a decentralized platform that runs smart contracts: applications that run exactly as programmed without any possibility of downtime, fraud, or third-party interference.
How do decentralized applications differ from traditional ones?
- Decentralized applications (DApps) run on a blockchain network, offering increased transparency, security, and resistance to censorship compared to traditional centralized applications.
What is blockchain technology?
- Blockchain is a distributed database that maintains a continuously growing list of records, called blocks, secured from tampering and revision.
How does blockchain technology impact Web3 development?
- Blockchain technology is foundational to Web3, providing the infrastructure for decentralized applications, enhancing security, and promoting data integrity.