Web3.js is a powerful library that unlocks the potential of interacting with the Ethereum blockchain and other Web3-compliant platforms. One of its core functionalities is sending transactions, enabling users to transfer funds, interact with smart contracts, and participate in the decentralized ecosystem. This article delves into the world of using Web3.js for sending transactions, guiding you through the process, best practices, and security considerations. Specifically, we will explore how to send Ethereum transactions using Web3.js, providing you with a step-by-step approach to harness this functionality effectively within your blockchain projects.
Understanding Transactions on the Blockchain
Before diving into Web3.js, let’s establish a basic understanding of blockchain transactions. A transaction on a blockchain network like Ethereum represents the transfer of value or data between accounts. This value can be cryptocurrency (e.g., Ether) or data relevant to a smart contract execution. Each transaction holds specific details, including:
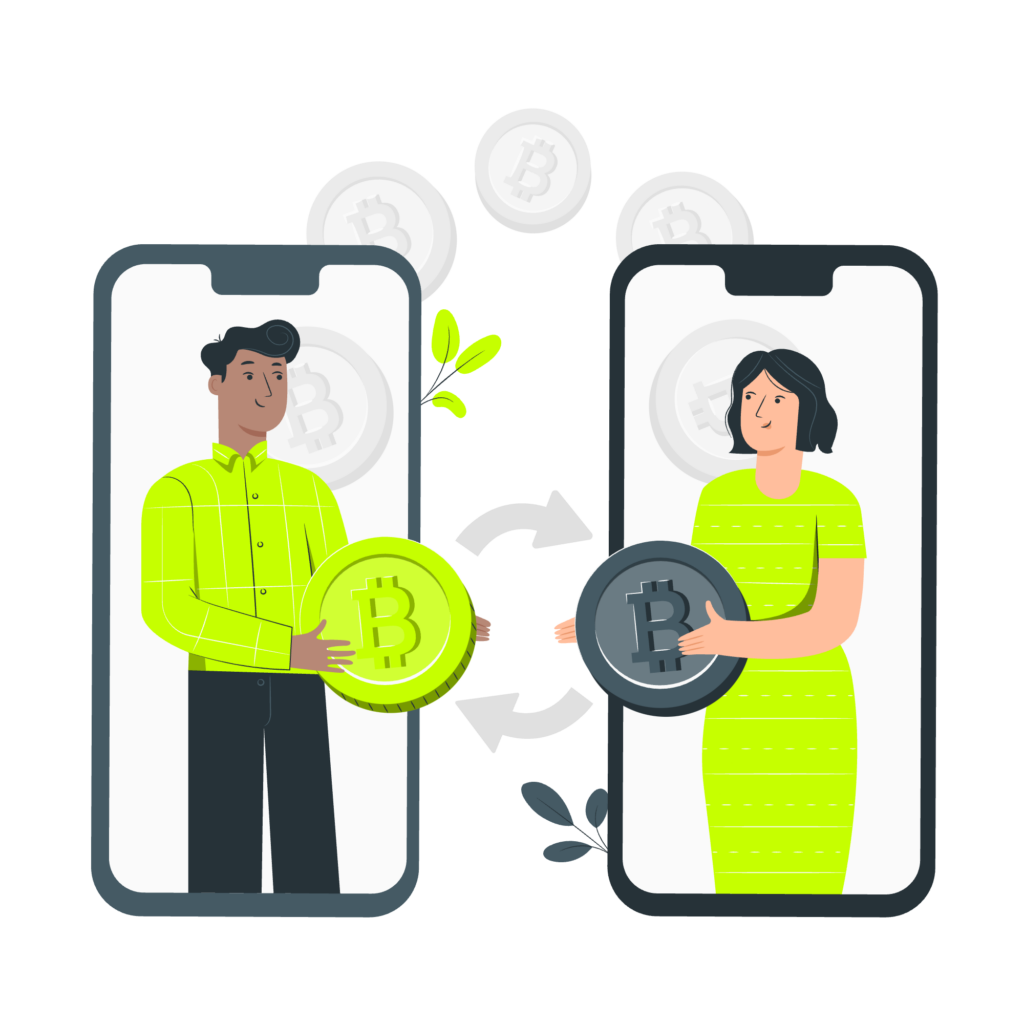
- Sender: The Ethereum address initiating the transaction.
- Receiver: The Ethereum address receiving the value or data.
- Value: The amount of cryptocurrency being transferred (in Wei, the smallest unit of Ether).
- Gas: The computational effort required to execute the transaction on the network. Miners or validators compete to solve cryptographic puzzles, and the winner receives a reward in cryptocurrency. Gas serves as a fee to incentivize miners and prevent spam transactions.
- Gas Price: The amount a sender is willing to pay per unit of gas. Setting a higher gas price increases the transaction’s priority and processing speed.
- Data (Optional): Additional data relevant to smart contract execution, if applicable.
- Nonce: A unique identifier to prevent replay attacks (rebroadcasting a previously executed transaction).
Gateway to Sending Transactions in Javascript
Web3.js is a JavaScript library that provides an abstraction layer for interacting with Ethereum nodes. It acts as a bridge between your application and the underlying blockchain network. By leveraging Web3.js, you can construct and send transactions programmatically, automating tasks and integrating blockchain functionalities into your applications.
Steps to Sending Transactions with Web3.js
Here’s a breakdown of the key steps involved in sending transactions with Web3.js:
- Setting Up Web3.js:
- Install the Web3.js library using npm or yarn:
npm install web3
- Import the library in your JavaScript code:
const Web3 = require('web3');
- Connecting to a Provider:
- Web3.js needs a provider to communicate with an Ethereum node. This node can be:
- A local Ethereum node (Geth, Parity): Requires running your own node software.
- A remote node provider: Services like Infura, Alchemy, and Etherscan provide access to public nodes.
- Here’s an example connecting to a remote node using Infura:
- Web3.js needs a provider to communicate with an Ethereum node. This node can be:
const infuraUrl = "<https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID>"; //Replace "YOUR_INFURA_PROJECT_ID" with your actual Infura project ID
const web3 = new Web3(new Web3.providers.HttpProvider(infuraUrl));
- Specifying Transaction Details:
- Create a transaction object containing the necessary information:
- Create a transaction object containing the necessary information:
const tx = {
from: "0xSenderAddress",
to: "0xReceiverAddress",
value: web3.utils.toWei("1", "ether"), // Convert value to Wei
gas: 21000, // Estimated gas limit for a simple transaction
gasPrice: web3.utils.toWei("50", "gwei"), // Gas price in Gwei
// Optional data for smart contract interactions
};
- Replace the placeholder addresses with your actual sender and receiver addresses.
- Consider using tools like https://etherscan.io/gastracker to estimate appropriate gas limits.
- Signing the Transaction (Private Key Approach):
- Web3.js allows signing transactions using a private key. This approach grants full control but requires secure storage of your private key. Never store private keys in plain text! Consider using a secure keystore or hardware wallet.
- Web3.js allows signing transactions using a private key. This approach grants full control but requires secure storage of your private key. Never store private keys in plain text! Consider using a secure keystore or hardware wallet.
const privateKey = "YOUR_PRIVATE_KEY"; // Replace "YOUR_PRIVATE_KEY" with your actual private key (**Hide your key in production!**)
const signedTx = await web3.eth.accounts.signTransaction(tx, privateKey);
- Sending the Transaction :
- Once the transaction is signed, use the sendTransaction method to broadcast it to the Ethereum network:
const txHash = await web3.eth.sendTransaction(signedTx);
console.log("Transaction hash:", txHash);
- The sendTransaction method returns a promise that resolves to the transaction hash upon successful broadcast. The transaction hash is a unique identifier used to track the transaction on the blockchain explorer.
Alternative Signing Methods
- Web3 Provider with Wallet:
- Some Web3 providers, like Infura and Alchemy, offer integration with popular wallets like MetaMask. This allows users to sign transactions directly within their wallets, enhancing security and user experience.
- Instead of using a private key, you can leverage the provider’s signing functionality:
const web3 = new Web3(window.ethereum); // Assuming MetaMask is injected
// ... transaction object definition
const txHash = await web3.eth.sendTransaction(tx);
console.log("Transaction hash:", txHash);
Error Handling and Monitoring
- Sending transactions can encounter errors. It’s crucial to implement proper error handling to catch potential issues like insufficient funds, invalid gas parameters, or network congestion.
- Utilize the catch block in your promises to capture errors and provide informative feedback to the user.
- Monitoring the transaction status is also vital. You can use the getTransactionReceipt method to retrieve information about a specific transaction by its hash:
const receipt = await web3.eth.getTransactionReceipt(txHash);
console.log("Transaction receipt:", receipt);
- The transaction receipt object provides details like the block number, transaction status (success/failure), and gas used.
Nonce Management and Gas Optimization
- The nonce in a transaction ensures each transaction is unique and prevents replay attacks. Web3.js can automatically manage the nonce for you in most cases.
- However, in complex scenarios or when dealing with multiple transactions, manual handling might be necessary. Refer to the Web3.js documentation for advanced nonce management techniques.
- Gas optimization is essential for minimizing transaction fees. Tools like https://etherscan.io/gastracker can help estimate gas limits. Consider setting a slightly higher gas limit to ensure smooth transaction processing, but avoid excessive overestimation.
Security Considerations
- Sending transactions with Web3.js involves handling sensitive information like private keys. Here are some critical security practices:
- Never store private keys in plain text.
- Consider using a secure keystore or hardware wallet for private key management.
- Implement robust access control mechanisms within your application to prevent unauthorized access to signing functionalities.
- Stay updated on the latest Web3.js vulnerabilities and security best practices.
Advanced Topics
This article provides a foundational understanding of sending transactions with Web3.js. As you delve deeper, you can explore more advanced topics like:
- Interacting with Smart Contracts: Web3.js facilitates interacting with smart contracts deployed on the Ethereum blockchain. You can call contract functions, send data, and manage contract state.
- Custom Providers: You can develop custom Web3 providers to interact with private blockchains or specialized node configurations.
Conclusion
Web3.js is an essential tool for developers, enabling interaction with the Ethereum blockchain. This guide has emphasized the importance of security and the advanced features of Web3.js, particularly focusing on “how to send Ethereum transactions using Web3.js.” This critical skill involves setting up the Web3.js environment, connecting to an Ethereum node, creating, signing, and broadcasting transactions. Mastering this process allows developers to efficiently build innovative applications that can transfer value and interact with smart contracts on the Ethereum network.
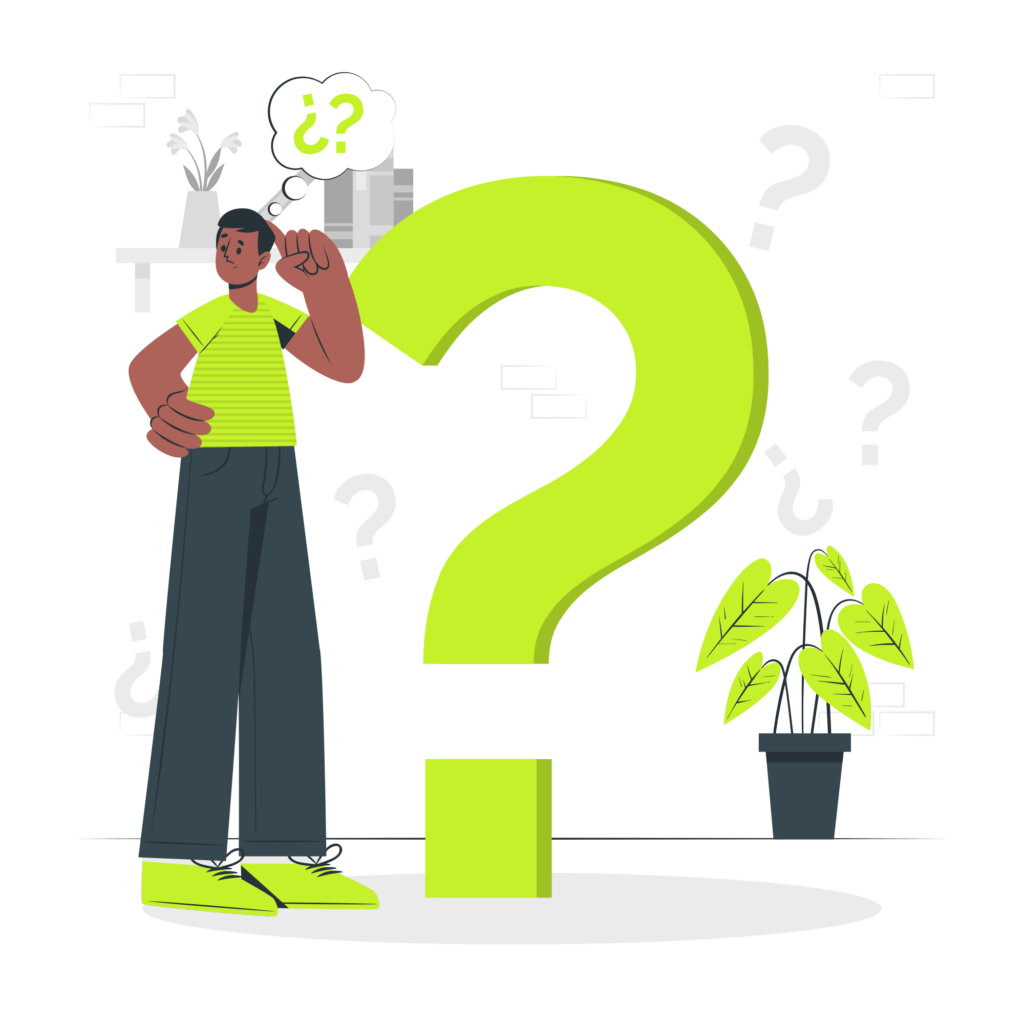
FAQs
What is Web3.js and how does it relate to Ethereum?
- Web3.js is a JavaScript library that allows you to interact with the Ethereum blockchain, enabling the creation and management of transactions.
How do I set up Web3.js to send Ethereum transactions?
- Set up Web3.js by installing the library, connecting it to an Ethereum node, and then using it to create and send transactions.
What information is needed to send an Ethereum transaction using Web3.js?
- You’ll need the recipient’s address, amount of Ether to send, gas limit, and your account’s private key for transaction signing.
How can I ensure the security of my Ethereum transactions with Web3.js?
- Always use secure methods to manage private keys and consider using hardware wallets or encrypted storage for keys.
What are some common errors to watch out for when sending Ethereum transactions with Web3.js?
- Common errors include incorrect nonce values, insufficient gas limits, and incorrect address formats.
What is Ethereum and why is it important in blockchain technology?
- Ethereum is a blockchain platform that enables smart contracts and decentralized applications, playing a crucial role in the blockchain ecosystem.
What are smart contracts and how do they work on Ethereum?
- Smart contracts are self-executing contracts with the terms directly written into code, automatically executed on the Ethereum blockchain.
How can developers ensure the security of their blockchain applications?
- Developers should conduct thorough testing, utilize security audits, and follow best practices in smart contract development.
What is the role of gas in Ethereum transactions?
- Gas is the fee required to conduct a transaction or execute a contract on the Ethereum network, compensating for the computing energy required.
What resources are available for learning more about Web3.js and Ethereum development?
- Developers can explore Ethereum’s official documentation, Web3.js documentation, online courses, and community forums for in-depth learning.