The world of blockchain technology develops on real-time communication and transparency. Smart contracts, the self-executing programs on blockchains, play a significant role in this dynamic environment. They can emit events to notify external applications about specific actions or state changes within the contract. Web3.js, a popular JavaScript library, provides powerful tools for interacting with Ethereum and other blockchain networks. Event handling in Web3.js allows developers to listen for these emitted events and react accordingly, creating a responsive and interactive user experience.
Understanding Events in Solidity Smart Contracts
Solidity, the primary language for smart contract development on Ethereum, provides the event keyword for defining events. These events act as communication channels for smart contracts to broadcast specific information to the outside world. They consist of a name and optional indexed arguments that provide detailed data about the event.
Here’s a simple example of an event definition in Solidity:
contract MyContract {
event Transfer(address indexed from, address indexed to, uint value);
function transfer(address recipient, uint amount) public {
// ... transfer logic ...
emit Transfer(msg.sender, recipient, amount);
}
}
In this example, the Transfer event is emitted whenever the transfer function is called. It includes three arguments:
- from: The address of the sender (indexed)
- to: The address of the receiver (indexed)
- value: The amount of tokens transferred
The indexed keyword signifies that these arguments can be used for efficient filtering when listening for events.
Listening for Events with Web3.js
Web3.js equips you with the necessary tools to listen for these events emitted by smart contracts. Here’s the basic flow of event handling:
- Obtain a Contract Instance: Create a Web3.js contract instance using the compiled contract ABI (Application Binary Interface) and the deployed contract address.
- Access Event Object: Use the events property of the contract instance to access the event object associated with the desired event name.
- Set Up Event Listener: Call the on method on the event object, specifying a callback function that will be triggered when the event is emitted.
Let’s see this in action with an example:
const Web3 = require('web3');
const MyContract = require('./MyContract.json'); // Assuming ABI is loaded
const web3 = new Web3('wss://mainnet.infura.io/v3/YOUR_INFURA_ID'); // Replace with your provider URL
const contractAddress = '0x...'; // Replace with your contract address
const myContract = new web3.eth.Contract(MyContract.abi, contractAddress);
const transferEvent = myContract.events.Transfer(); // Access the Transfer event
transferEvent.on('data', (event) => {
console.log('Transfer Event:', event);
// Process the event data (from, to, value)
});
// Listen for the event
transferEvent.watch((error, event) => {
if (error) {
console.error('Error watching for event:', error);
} else {
console.log('Transfer Event (watch):', event);
}
});
This code snippet first imports the necessary modules, establishes a connection to the Ethereum network using a Web3 provider, and retrieves the contract instance. We then access the Transfer event object and attach two listeners:
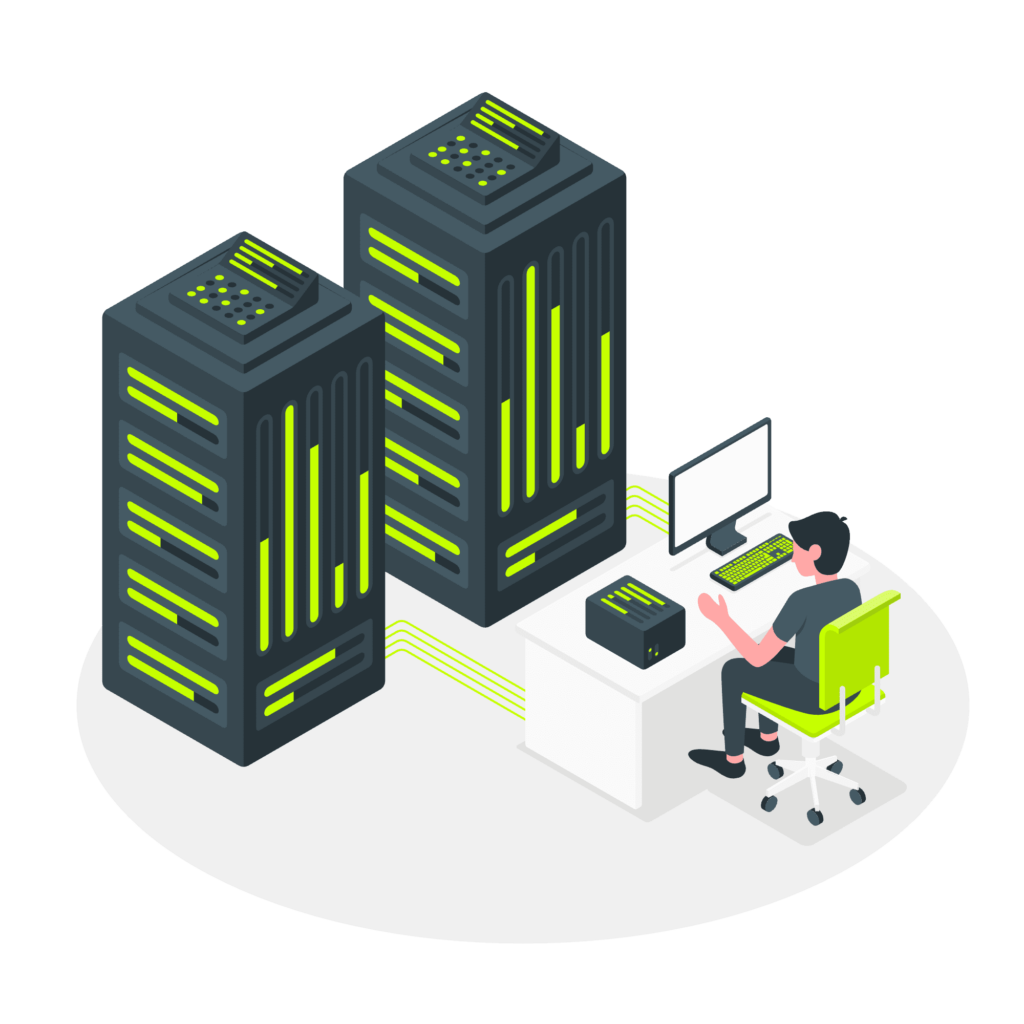
- on(‘data’): This listener fires whenever a new Transfer event is received. The callback function receives the event object as an argument, containing details about the event, including the indexed arguments.
- watch(): This method starts a continuous watch for the event. It takes a callback function with two arguments: an error object (if any) and the event object (including details about the emitted event).
By understanding these methods and the information provided within the event object, you can effectively react to smart contract activity and build responsive dApps that interact with the blockchain in real-time.
Listening for Specific Events
While the previous example listened for all instances of the Transfer event, you might want to filter for specific occurrences. Web3.js allows you to define filter options within the event listener:
const transferSubscription = transferEvent.on('data', {
from: '0x...', // Filter by sender address
}, (event) => {
console.log('Transfer from specific address:', event);
});
Here, we added a filter object to the on method, specifying that we only want to be notified for transfers originating from a particular address. You can similarly filter based on other indexed arguments like the recipient address or the transferred amount.
Filtering Events with Options
The filter options object offers various filtering capabilities:
- fromBlock: Specifies the starting block number for event retrieval (inclusive).
- toBlock: Specifies the ending block number for event retrieval (inclusive).
- address: An array of addresses to filter events by (applicable to non-indexed arguments).
- topics: An array of filters for indexed arguments. Each element can be:
- A specific value (string or number) to match the exact argument.
- An empty string (”) to match any value for that argument.
- An array of values for that argument (allows for multiple possibilities).
Here’s an example filtering for transfers with a specific amount:
transferEvent.on('data', {
topics: [null, null, web3.utils.toBN(100)], // Filter for transfers of 100 tokens (value argument)
}, (event) => {
console.log('Transfer of 100 tokens:', event);
});
Handling Event Data
The callback function you provide to the on or watch method receives the event object as an argument. This object contains valuable information about the emitted event, including:
- event: The name of the event (matches the event name in the contract).
- args: An array containing the arguments passed to the event (in the order they were defined).
- blockHash: The hash of the block where the event was emitted.
- blockNumber: The block number where the event was emitted.
- logIndex: The index of the event within the block.
- transactionHash: The transaction hash that triggered the event.
By accessing these properties within your callback function, you can effectively process and react to the data provided by the smart contract.
Unsubscribing from Events
It’s crucial to unsubscribe from event listeners when you no longer need them to avoid resource leaks and unexpected behavior. Web3.js provides two methods for unsubscribing:
- removeListener: This method removes a specific listener attached to the event object using the on method.
- stopWatching: This method stops the continuous watch initiated with the watch method.
Here’s an example of unsubscribing from the Transfer event subscription:
transferSubscription.removeListener('data', (event) => {
// ... processing logic ...
});
transferEvent.stopWatching();
Remember to unsubscribe from event listeners when your application logic no longer requires listening for them.
Advanced Event Handling Scenarios
Beyond basic listening for new events, Web3.js offers advanced functionalities for event handling. This includes techniques for:
- Retrieving past events that have already occurred on the blockchain.
- Subscribing to events emitted by the entire network, like new block additions.
- Implementing robust error handling mechanisms to ensure smooth operation during event processing.
These advanced features allow developers to create more sophisticated dApps that can not only react to real-time events but also analyze historical data and handle potential issues during event retrieval or subscription.
Listening for Past Events
Sometimes, you might need to access historical events that have already been emitted on the blockchain. Web3.js allows you to retrieve past events using the getPastLogs method:
const pastTransferEvents = await transferEvent.getPastLogs({
fromBlock: 10000, // Starting block number
toBlock: 10100, // Ending block number
});
pastTransferEvents.forEach((event) => {
console.log('Past Transfer Event:', event);
});
This code retrieves all Transfer events emitted between blocks 10000 and 10100 (inclusive).
Event Listeners with Providers
While the previous examples focused on event listeners attached to contract instances, Web3.js also allows listening for events emitted by the entire network through the Web3 provider:
web3.eth.subscribe('newBlock', (error, block) => {
if (error) {
console.error('Error subscribing to newBlock event:', error);
} else {
console.log('New Block:', block);
}
});
Here, we subscribe to the newBlock event emitted by the provider, which fires whenever a new block is added to the blockchain. You can similarly subscribe to other provider events like pendingTransactions or syncing.
Error Handling and Considerations
When working with event listeners, it’s essential to consider error handling:
- The on and watch methods can potentially throw errors during subscription or event retrieval. Implement proper error handling mechanisms (try-catch blocks) in your callback functions.
- The getPastLogs method might return an empty array if no events matching the filter criteria are found. Check for empty results before processing the retrieved events.
Remember that event listeners can consume resources, especially when monitoring frequently emitted events. Be mindful of the frequency of events and optimize your listener logic to avoid overloading the application.
Conclusion
Web3.js event handling provides a powerful mechanism for staying in sync with the blockchain and responding to smart contract activity. This step by step guide has provided you with the knowledge and practical examples to use event listeners effectively in developing dApps. Remember to explore the documentation for advanced features and stay updated on the latest developments in Web3.js to get to know the full potential of event handling in your blockchain applications.
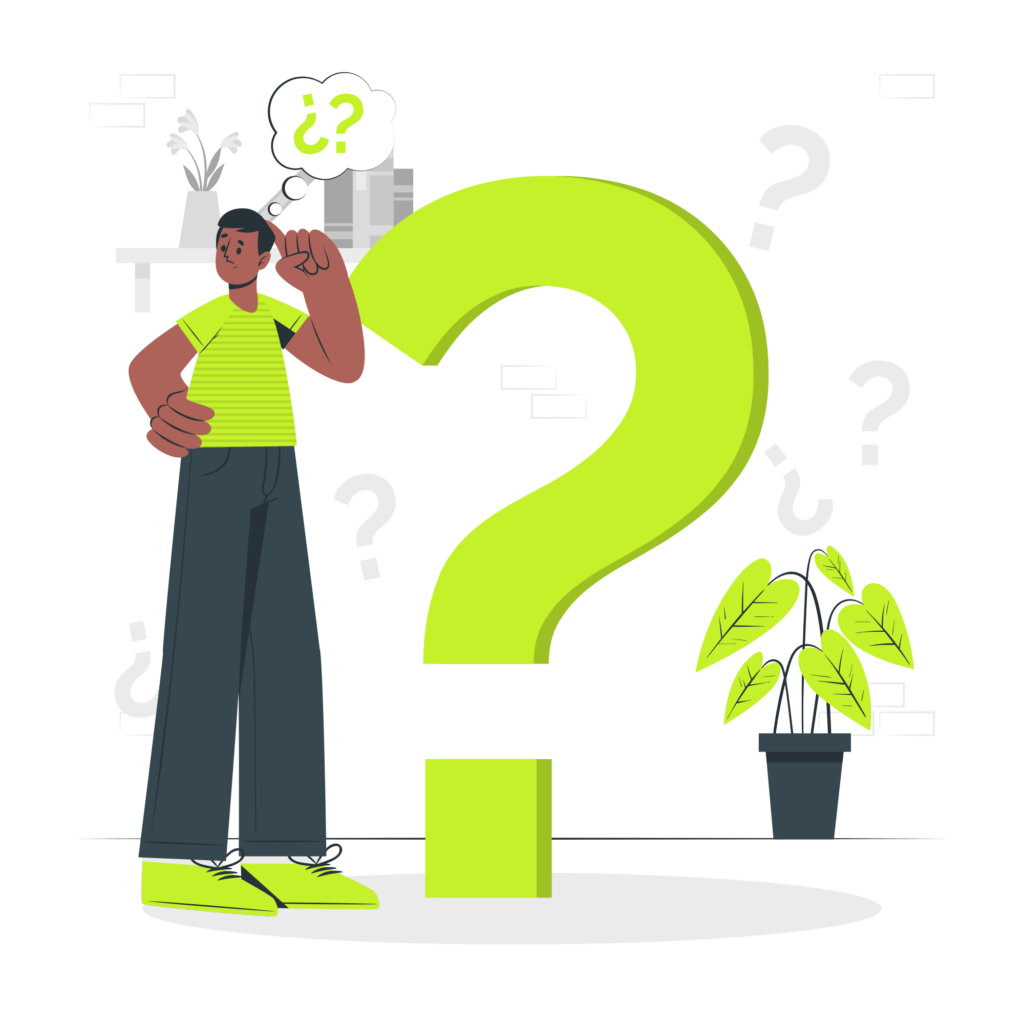
FAQs
What is event handling in web3.js?
- Event handling in web3.js refers to the process of listening to and responding to events emitted by smart contracts on the blockchain, facilitating interactive dApp features.
How do you listen to smart contract events using web3.js?
- Use web3.js’s
contract.events
API to subscribe to and react to specific contract events, enabling your application to update in real-time based on blockchain activities.
Why is event handling important in blockchain development?
- It allows developers to create responsive and dynamic dApps that react to on-chain events, enhancing user experience and enabling real-time data updates.
Can web3.js handle events from past blocks?
- Yes, web3.js can retrieve and listen to events from past blocks, allowing developers to access historical blockchain data for their applications.
How does web3.js integrate with frontend frameworks for event handling?
- Web3.js can be integrated with frontend frameworks like React or Angular to dynamically update the UI in response to blockchain events, linking backend blockchain activities with frontend user interfaces.
What is web3.js?
- Web3.js is a JavaScript library that enables interaction with a local or remote Ethereum node, facilitating the development of applications that interact with the Ethereum blockchain.
What are smart contracts?
- Smart contracts are self-executing contracts with the terms of the agreement directly written into code, running on the blockchain and automatically enforcing their terms.
What is a dApp?
- A decentralized application (dApp) is an application that runs on a blockchain or P2P network, offering functionality without a central point of control.
How do JavaScript developers benefit from web3.js?
- JavaScript developers can leverage web3.js to build blockchain-based applications, utilizing their existing skills to interact with Ethereum and other compatible blockchains.
What are the key components of blockchain event handling?
- Key components include event listeners, event response functions, and integration with the application’s frontend, ensuring dynamic updates in response to blockchain activities.