Solidity, the programming language powering Ethereum smart contracts, offers robust tools for code reusability. Two key approaches dominate this landscape: inheritance and libraries. While both serve the purpose of reducing code redundancy, they differ significantly in functionality and implementation. Understanding these differences is crucial for crafting efficient and secure smart contracts.
This article delves into the concepts of inheritance and libraries in Solidity. We’ll explore their characteristics, applications, and best practices to guide you in making informed decisions while building your smart contracts.
Inheritance: Building Upon Existing Functionality
Inheritance allows you to create a new contract (derived contract) that inherits functionalities and state variables from an existing contract (base contract). This establishes an “is-a” relationship. The derived contract can access and potentially override functions and variables defined in the base contract, extending its functionality.
In this example, MyContract inherits from Ownable. This grants MyContract access to the owner variable and the onlyOwner modifier from Ownable. Additionally, MyContract defines its own variable value and a function setValue.
contract Ownable {
address public owner;
constructor() public {
owner = msg.sender;
}
modifier onlyOwner() {
require(msg.sender == owner, "Only owner can call this function.");
_;
}
}
contract MyContract is Ownable {
uint public value;
function setValue(uint _value) public onlyOwner {
value = _value;
}
}
Benefits of Inheritance:
- Reduced Code Duplication: By inheriting common functionalities, you avoid rewriting the same code in multiple contracts. This simplifies development and maintenance.
- Enforced Functionality: Base contracts can define essential functions, ensuring derived contracts inherit necessary functionalities.
- Code Organization: Inheritance promotes code organization by grouping related functionalities into base contracts.
Challenges of Inheritance:
- Increased Contract Size: Derived contracts become larger as they inherit code from the base contract. This can lead to higher deployment costs (gas fees).
- Tight Coupling: Changes made to the base contract can potentially affect all derived contracts. Careful planning is required to avoid unintended consequences.
- Diamond Problem: In complex inheritance hierarchies, the “diamond problem” can arise where a derived contract inherits the same variable or function from multiple base contracts, leading to ambiguity.
Libraries: Reusable Utility Functions
Solidity libraries are collections of functions that can be used by other contracts. Unlike inheritance, libraries do not have their own storage and cannot hold state variables. They primarily provide reusable utility functions.
library SafeMath {
function add(uint a, uint b) public pure returns (uint) {
uint c = a + b;
require(c >= a, "SafeMath: addition overflow");
return c;
}
}
contract MyContract {
using SafeMath for uint;
function increment(uint value) public {
value = value.add(1);
}
}
In this example, the SafeMath library provides a safe add function that prevents overflow errors. The MyContract uses the using statement to import the add function and make it available with a shorter syntax (value.add(1)).
Benefits of Libraries:
- Reduced Gas Costs: Libraries are deployed only once on the blockchain, and their code is reused by referencing contracts. This minimizes gas costs associated with deploying code repeatedly.
- Modular Code: Libraries promote modularity by separating utility functions from core contract logic.
- Improved Security: Commonly used and well-tested libraries like SafeMath can enhance the security of your contracts by preventing vulnerabilities.
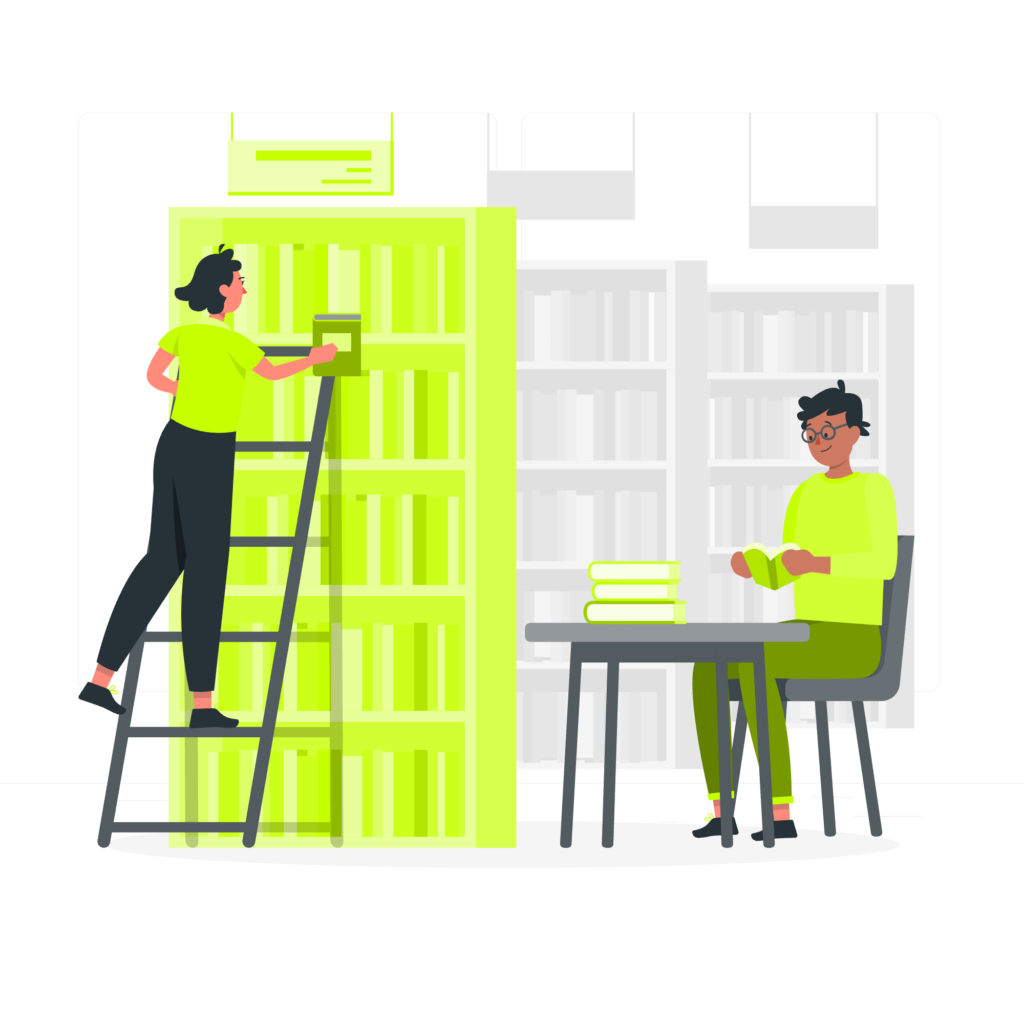
Challenges of Libraries:
- Limited Functionality: Libraries cannot hold state or manage storage, limiting their applicability for functionalities requiring persistent data.
- Increased Reliance on External Code: Using libraries introduces dependencies on external code. Choosing well-maintained and secure libraries is crucial.
When to Use Inheritance vs. Libraries
The choice between inheritance and libraries depends on your specific needs:
Use Inheritance When:
- Extends Functionality: New contract builds upon and modifies existing contract behavior.
- Enforces Behavior: Derived contracts inherit essential functions from a base contract.
- Clear “Is-A” Hierarchy: Building related contracts with a clear parent-child relationship.
Use Libraries When:
- Reusable Functions: Functions don’t require storage and need reuse across contracts.
- Minimize Gas Costs: Frequently used code is deployed once and referenced by multiple contracts.
- Modular Code: Separate core contract logic from reusable functions for better maintainability.
Note: If you’re familiar with web3.js, a popular library for interacting with Ethereum from JavaScript applications, libraries in Solidity function similarly. They provide reusable functionalities that can be integrated into your smart contracts. However, unlike web3.js libraries that reside outside the blockchain, Solidity libraries are deployed on the blockchain itself.
Best Practices for Inheritance and Libraries
Following best practices ensures you leverage inheritance and libraries effectively while mitigating associated challenges:
Inheritance:
- Favor Shallow Inheritance Hierarchies: Deep inheritance hierarchies can become complex and difficult to maintain. Aim for a maximum of two or three levels of inheritance.
- Abstract Contracts: Define abstract contracts as base contracts that cannot be directly instantiated. This ensures derived contracts implement essential functionalities before being deployed.
- Virtual Functions: Utilize virtual functions in base contracts for functions that derived contracts can override with customized behavior.
- Carefully Consider Base Contract Modifications: Thoroughly test the impact of changes made to base contracts on all derived contracts to avoid unintended consequences.
Libraries:
- Choose Well-Tested Libraries: Opt for well-established and thoroughly tested libraries from reputable sources like OpenZeppelin to minimize security risks.
- Understand Library Dependencies: Be aware of potential dependencies introduced by libraries and ensure compatibility with your project’s requirements.
- Clearly Document Library Usage: Document how you’re using libraries within your contracts to improve code clarity and maintainability.
Advanced Techniques:
- Multiple Inheritance: While generally discouraged due to the diamond problem, careful planning and use of abstract contracts can allow for controlled multiple inheritance in specific scenarios.
- Inheritance with Libraries: You can combine inheritance and libraries by having a base contract inherit from a library, providing derived contracts with access to both functionalities. However, exercise caution to avoid unnecessary complexity.
Conclusion
Inheritance and libraries are powerful tools in the Solidity developer‘s arsenal. Understanding their strengths and weaknesses allows you to create modular, reusable, and secure smart contracts. By following best practices and carefully considering your project’s needs, you can leverage these techniques to build efficient and robust blockchain applications.
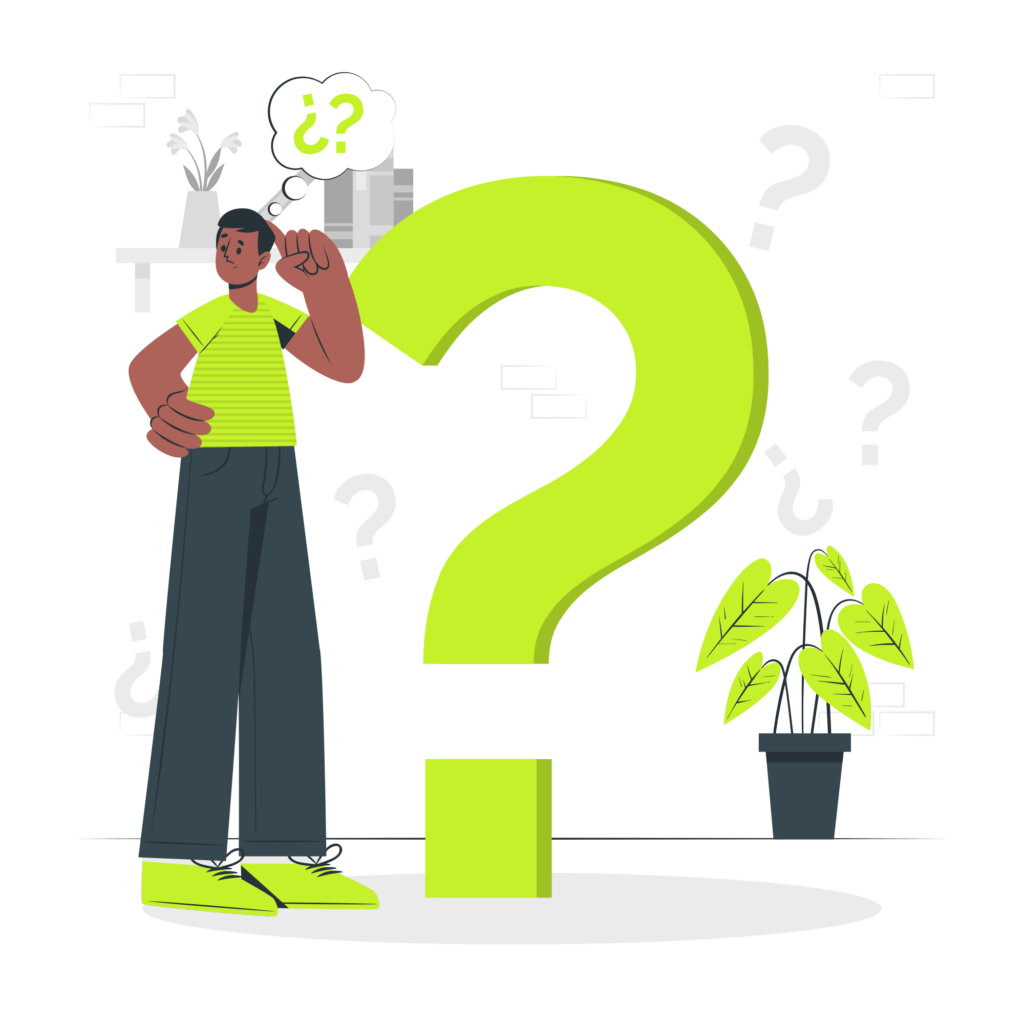
FAQs
What are Solidity libraries?
- Solidity libraries are reusable pieces of code deployed once and used across multiple contracts, optimizing gas costs and code organization in Ethereum development.
How does inheritance work in Solidity?
- Inheritance in Solidity allows contracts to derive properties and functions from one or more parent contracts, promoting code reusability and logical structure in smart contract design.
What are the benefits of using libraries in Solidity?
- Libraries in Solidity reduce redundancy, lower gas costs, and improve contract organization, making them a crucial tool for efficient and cost-effective blockchain development.
Can a Solidity library inherit from another contract or library?
- Yes, Solidity libraries can inherit from other libraries but not from contracts, which ensures modular, efficient, and reusable code in smart contract ecosystems.
How do I implement a library in my Solidity contract?
- Implement a library in Solidity by declaring it with the
library
keyword, deploying it, and then using it in your contract with theusing
keyword for specific data types.
What is Ethereum programming?
- Ethereum programming involves developing decentralized applications (dApps) and smart contracts using languages like Solidity, facilitating transactions and agreements without intermediaries.
How do smart contracts work?
- Smart contracts are self-executing contracts with the terms of the agreement directly written into lines of code, automatically enforced and executed on the blockchain.
What is blockchain development?
- Blockchain development involves creating decentralized databases and applications that allow secure, transparent, and tamper-proof transactions and data storage.
How does code reusability benefit smart contract development?
- Code reusability enhances smart contract development by saving time, reducing errors, and ensuring consistent behavior across different parts of a blockchain application.
What is code efficiency in smart contracts?
- Code efficiency in smart contracts refers to writing optimized, gas-efficient code that minimizes execution costs and enhances performance on the blockchain.