JavaScript array comprehension provides a powerful way to construct arrays from existing data without the need to explicitly write loops, providing a more cleaner and readable code structure. This feature could simplify the development process by allowing developers to apply transformations, filtering, and mapping operations in a concise manner, making it easier to manipulate data within arrays. Array comprehension improves code efficiency and readability, reducing the chances of errors that often come with manually written loops.
This article offers a comprehensive guide to the concept of array comprehension in JavaScript, exploring its benefits and how it can be used to manage larger datasets effectively. Understanding these concepts is important for developers looking to build robust, full-stack applications.
What is Array Comprehension ?
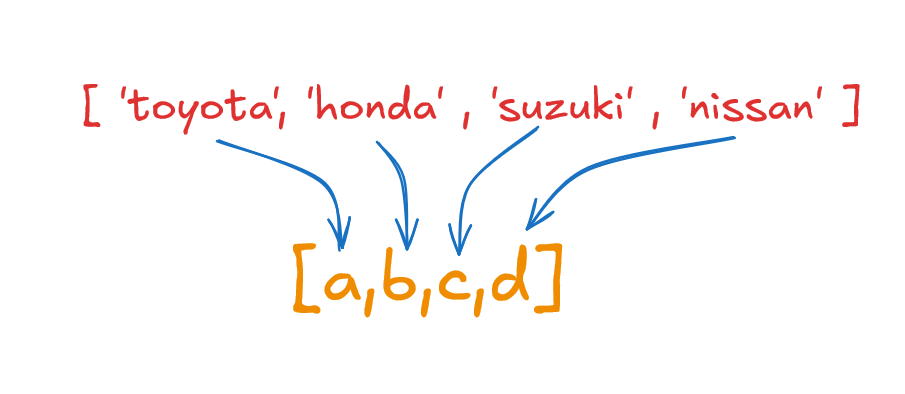
Array comprehensions are expressions that enable the creation of new arrays by specifying how each element in the new array is derived from the corresponding elements of an existing array. While this feature was historically available in JavaScript, it has not been widely adopted in the official ECMAScript specification, limiting its use in modern development practices. However, modern javascript provides a functional approach to achieve the same results using array methods.
The general structure of an array comprehension in JavaScript is as follows
let newArray = [expression for element in array if condition]
The expression
refers to the operation applied to each element of the original array, where the element
serves as a variable representing the current item being processed. The array
represents the original data set, and the condition
is optional that can be included to filter elements based on specific criteria.
Array comprehensions has two main functionalities.
- Transformation
- Applying a function or expression to each element of an array
- Filtering
- Filtering out elements and only Include elements that meet a specific criteria.
Benefits of Array Comprehension
Array comprehension in JavaScript streamlines the process of managing larger datasets. It enables developers to write concise, efficient code when working with arrays. Following are some key advantages of using array comprehension.
Benefit | Description |
---|---|
Code Readability | Using array comprehension makes your code more readable and concise. Instead of managing loops and temporary arrays, you can simply focus on the transformation logic itself. |
Functional Programming | Array comprehension align well with functional programming principles, emphasizing immutability and declarative style. |
Performance | Array comprehension is optimized by Javascript engines, making is more efficient than traditional loop based approaches. |
Chaining for complex operations | Array methods can be chained together, which allows you to write complex operations in a clean, expressive way. |
Array Comprehension before ES6
Now that we have a clear understanding of array comprehension, let’s explore some practical use cases of how this concept was applied in earlier versions of JavaScript.
1. Filtering Elements
To understand how to filter elements from an array, consider this example use case. Let’s try to filter out the even numbers from a given original array.
const originalArray = [1,2,3,4,5,6,7,8,9,10]
const newArray = [x for x in originalArray if x % 2 == 0]
console.log(newArray) // [2,4,6,8,10]
In this code, we start with an originalArray
containing numbers from 1 to 10. The second line creates a new array by selecting only the even numbers from the originalArray
. It does this by checking if each number in the originalArray
is divisible by 2 . If the number is even, it gets added to the newArray
. The result, when printed, is [2, 4, 6, 8, 10]
.
2. Squaring Elements
Let’s explore how we can easily square the elements of an original array by referring to the following example.
const originalArray = [1,2,3,4,5]
const newArray = [x * x for x in originalArray]
console.log(newArray) // [1,4,9,16,25]
In this code, we begin with an originalArray
containing numbers from 1 to 5. The second line generates a newArray
by squaring each element of the originalArray
. It achieves this by multiplying each element by itself and adding the squared values to the newArray
. Since we want to include all elements, no condition is specified. When printed, the result is [1, 4, 9, 16, 25]
.
3. Mapping Objects
Let’s explore how we can utilize array comprehension with an array containing custom objects. This approach allows us to create new arrays based on specific properties or attributes of the objects within the original array.
const originalArray = [
{ name: 'Alice', age: 30 },
{ name: 'Bob', age: 25 },
{ name: 'Charlie', age: 35 }
];
const newArray = [user.name for user in originalArray]
console.log(newArray) // ['Alice', 'Bob', 'Charlie']
In this code snippet, we have an originalArray
containing user objects with name
and age
properties. The second line uses array comprehension to create a newArray
that extracts just the name
of each user. The result, ['Alice', 'Bob', 'Charlie']
, showcases how efficiently we can retrieve specific properties from an array of objects.
4. Nested Array Comprehension
Let’s explore how we can utilize array comprehension with nested arrays, which represent a more complex data structure. This approach allows us to efficiently access and manipulate elements within multi-dimensional arrays.
To understand the concept effectively, let’s consider the following example, which demonstrates how to combine all elements from a nested array into a single array.
const originalNestedArray = [[1,2], [3,4], [5,6]]
const newArray = [x for row in originalNestedArray for x in row]
console.log(newArray)
In this code, we start with an originalNestedArray
that contains multiple arrays. The second line creates a new array by flattening the nested arrays. It does this by iterating through each row
in the originalNestedArray
and then extracting each element from those rows. As a result, the newArray
contains all the elements from the nested arrays combined into a single array. When printed, the output is [1, 2, 3, 4, 5, 6]
.
Array Methods in JavaScript
Even though array comprehension makes process simple while handling larger datasets it didn’t make it into ECMAScript. However, modern javascript achieves the same goals using higher-order array methods. These methods are part of the functional programming paradigm and allow you to write clean, declarative code.
filter()
The filter()
method allows you to create a new array that includes only the elements that meet a specific condition.
const originalArray = [1, 2, 3, 4, 5, 6];
const newArray = originalArray.filter(n => n % 2 === 0);
console.log(newArray); // [2, 4, 6]
In this code, the filter function generates a new array, containing only the even numbers from the originalArray
.
map()
The map()
method creates a new array by applying a function to each element of an existing array.
const originalArray = [1, 2, 3, 4];
const newArray = originalArray.map(n => n * n);
console.log(newArray); // [1, 4, 9, 16]
In this code, the map
function creates a new array that contains the squared values of each element from the originalArray
.
reduce()
The reduce()
method allows you to combine elements of an array into a single value.
const originalArray = [1, 2, 3, 4];
const sum = originalArray.reduce((acc, n) => acc + n, 0);
console.log(sum); // 10
In this code, the reduce
function iterates through all elements of the array and calculates their total, assigning the final sum to the variable sum
.
Conclusion
Array comprehensions are a valuable tool in JavaScript for creating new arrays in a concise and expressive manner. Their ability to express complex transformations and filtering in a single line of code makes them a valuable tool for both beginners and experienced developers. Even though array comprehensions are not yet part of the official ECMAScript standard, developers can achieve similar results using JavaScript methods. These methods promote clean and concise code, encouraging functional programming practices that enhance maintainability and readability.
By understanding the fundamentals of array comprehensions, you can write more efficient and expressive JavaScript code.
FAQs
What are array comprehensions in JavaScript?
- Array comprehensions are a syntactic feature that allows you to create a new array from an existing one by applying transformations or filtering criteria. Although they were once proposed for JavaScript, they are not part of the official ECMAScript specification. Instead, modern JavaScript uses methods like
map()
,filter()
, andreduce()
to achieve similar functionality.
Why didn’t array comprehensions make it into the ECMAScript specification?
- Array comprehensions were proposed as a feature in earlier drafts of ECMAScript but were ultimately left out due to concerns about complexity, readability, and potential confusion with existing array methods. The JavaScript community favored using existing higher-order functions like
map()
,filter()
, andreduce()
, which provide clear and concise alternatives for array transformations and filtering without introducing new syntax.
Can array comprehensions be used to modify existing arrays?
- No, array comprehensions create new arrays with the modified data. If you need to modify an existing array, you’ll still need to use traditional loop-based approaches or methods like
map
,filter
, andreduce
.
Are array comprehensions supported in all JavaScript environments?
- No, array comprehensions are not supported in older JavaScript environments. You might need to use a transpiler like Babel to ensure compatibility with older browsers or environments.