TL;DR
The Browser Object Model (BOM) allows JavaScript to interact with the browser, enabling dynamic web experiences. Key components include:
- Window Object: Represents the browser window and provides methods like
alert()
,location
, andopen()
. - Navigator Object: Provides information about the browser and system.
- History Object: Manages browser history and navigation.
- Location Object: Handles the current URL and navigation.
BOM enables tasks like responsive design, user feedback, form validation, and geolocation. To build interactive sites, you must understand how to use BOM with the DOM to manipulate content and behavior. Always consider security practices like user permissions and input validation.
Imagine you’re a web developer and your web page is a stage play. The actors are the HTML elements, the script is your JavaScript code, and the audience experiences everything through the web browser. But what if you, the director, need to interact with the audience directly? Here’s where the Browser Object Model (BOM) comes in!
What is Browser Object Model? The BOM is like a hidden toolbox within the browser specifically designed for JavaScript. It provides a set of objects that act as bridges, allowing your JavaScript code to interact with various aspects of the browser window, history, navigation, and even the user’s device.
This article will be your friendly guide to the wonders of the JavaScript BOM. We’ll explore its key objects, properties, and methods, empowering you to create dynamic and interactive web experiences.
The Window Object
Think of the window
object as the main character in the BOM play. It represents the entire browser window, including the document you’re working on, the address bar, and the scrollbars. Through the window
object, you can access various properties and methods to control different aspects of the browser’s behavior.
Properties:
window.location
: This property holds information about the current web page’s URL. You can even use it to navigate to new pages or manipulate the URL itself. Imagine usingwindow.location.href = "https://www.example.com"
to redirect users to a new website!
window.history
: This property allows you to play with the user’s browsing history. You can access previously visited pages or even add new entries, giving you the power to control the user’s navigation flow.
window.document
: This property refers to the entire HTML document loaded within the browser window. It’s like a gateway to all the HTML elements on your web page, allowing you to manipulate them using JavaScript.
window.screen
: This property provides details about the user’s screen, such as its width, height, and color depth. This information can be crucial for creating responsive web designs that adapt to different screen sizes.
Methods:
window.alert()
: This is a classic method that displays a pop-up message box familiar to most internet users. While it can be useful for quick notifications, overuse is discouraged as it can disrupt the user experience.
window.confirm()
: This method presents the user with a confirmation dialog box with “OK” and “Cancel” buttons. Ideal for situations where you need user confirmation before performing an action.
window.open()
: This method opens a new browser window or tab. Useful for creating pop-up windows or opening external links within your application.
Exploring Other BOM Objects
While the window
object is the star of the show, the BOM offers a supporting cast of other objects with unique functionalities. Here are a few noteworthy ones:
- Navigator Object: This object provides information about the user’s browser, operating system, and platform.
- Location Object: As mentioned earlier, this object delves deeper into the details of the current URL. You can access components like hostname, protocol, and search parameters.
- History Object: This object allows you to track and manipulate the user’s browsing history within your web application.
- Document Object: This object acts as a gateway to the entire HTML document, providing access to all its elements like headings, paragraphs, and images.
Practical Applications of the BOM
Now that you’ve met the key players, let’s see how the BOM can be used to create dynamic and interactive web experiences:
- Responsive Design: Using
window.screen
properties, you can detect the user’s screen size and adjust your web page layout accordingly, ensuring an optimal experience on desktops, tablets, and mobile devices. - Interactive Forms: By manipulating form elements within the
window.document
object, you can create real-time validation, implement progress bars while forms are submitted, or even pre-fill form fields based on user preferences. - Navigation Control: Using methods like
window.history.back()
andwindow.history.forward()
, you can create custom navigation buttons that allow users to move back and forth through their browsing history within your web application. - User Feedback: Methods like
window.alert()
andwindow.confirm()
can be strategically used to provide clear feedback to users about their actions, like confirmation messages before deleting data or pop-up notifications.
Exploring Advanced BOM Techniques
The BOM offers more than just basic functionalities. Let’s delve into some advanced techniques to truly unlock its potential:
- Managing Browser Events: The BOM allows you to capture various events that occur within the browser window. These events can be triggered by user actions like clicking buttons, resizing the window, or even pressing keyboard keys. By using event listeners attached to specific objects, you can create dynamic and responsive web pages.
var button = document.getElementById("submitBtn");
button.addEventListener("click", function() {
// Code to be executed when the button is clicked
// For example, form validation or data submission logic
});
- Timers and Intervals: The BOM provides methods like
window.setTimeout()
andwindow.setInterval()
to control the timing of events in your web application. You can use these methods to create animations, timed messages, or even automatic tasks that run periodically in the background. - Geolocation: With user permission, the BOM allows you to access the user’s geographical location through the
navigator.geolocation
object. This information can be used to personalize content based on the user’s location, such as displaying weather forecasts or nearby restaurants.
Security Considerations and Best Practices
While the BOM offers great flexibility, it’s important to prioritize security in your web development practices. Here are some key considerations:
- User Permissions: Always obtain user consent before accessing sensitive information like location or manipulating browser history.
- Validation: Validate user inputs to prevent malicious code injection techniques like Cross-Site Scripting (XSS) attacks.
- Sandboxing: Consider using techniques like sandboxing to restrict the capabilities of external scripts running on your web page.
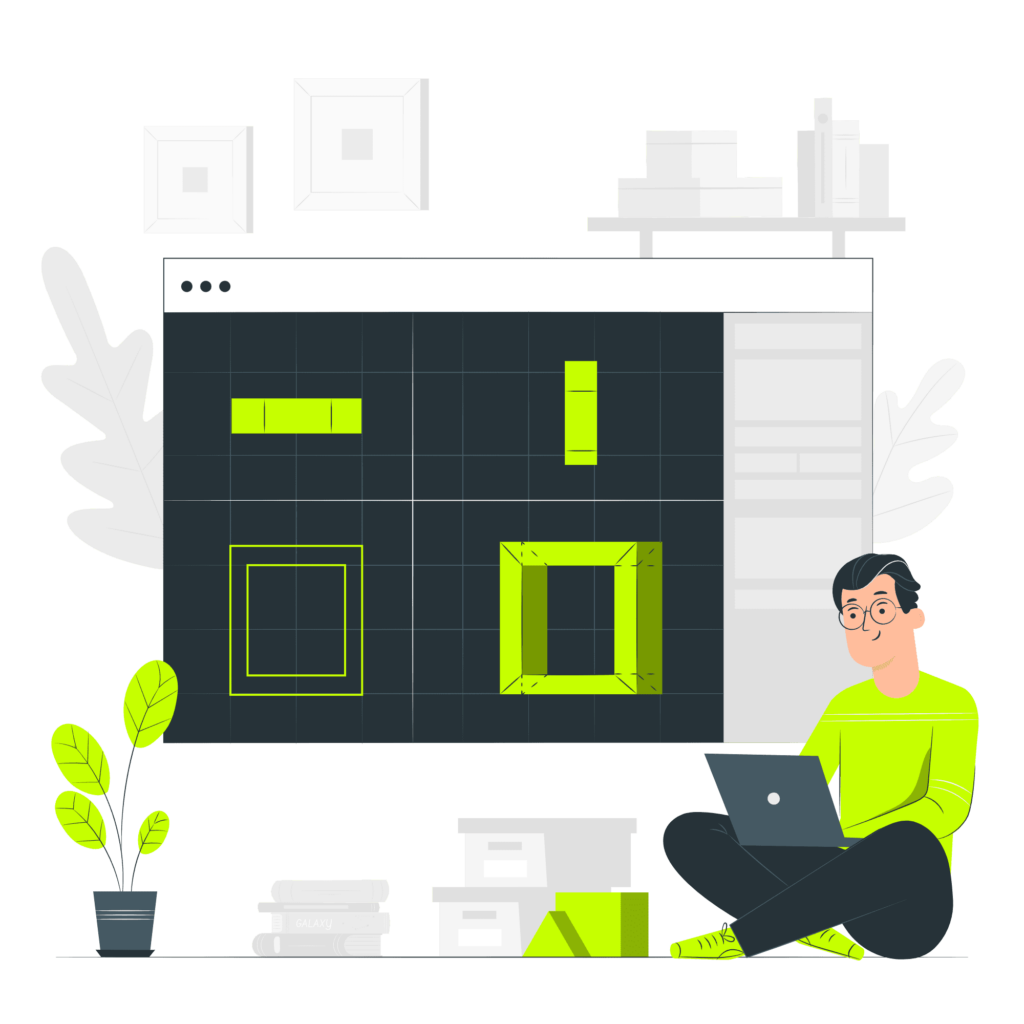
The DOM Connection
The BOM works hand-in-hand with another crucial concept in web development: the Document Object Model (DOM). The DOM represents the hierarchical structure of your HTML document, allowing you to access and manipulate individual elements like paragraphs, images, and forms.
Imagine the BOM as the conductor of the orchestra, while the DOM represents the individual instruments. The conductor (BOM) uses its tools to coordinate the instruments (DOM) and create a harmonious experience for the audience (user).
Understanding both the DOM and BOM is essential for creating truly interactive and dynamic web experiences.
Conclusion: What is Browser Object Model (BOM)
The Browser Object Model (BOM) is a powerful tool that empowers JavaScript to interact with the browser window, history, navigation, and even the user’s device. By understanding the key objects, properties, and methods of the BOM, you can create dynamic and engaging web experiences that go beyond static content.
FAQs:
What is the JavaScript Window object?
- The JavaScript Window object represents the browser window and provides methods and properties to interact with it.
How does the Browser Object Model (BOM) differ from the Document Object Model (DOM)?
- BOM refers to browser-related objects, while DOM deals with document content structure.
What are some common methods of the JavaScript Window object?
- Common methods include
alert()
,confirm()
,prompt()
, andopen()
.
What is Browser Object Model?
- The Browser Object Model (BOM) is a collection of objects that allows JavaScript to interact with the browser (e.g., manipulating the browser window, controlling history, or managing alerts). It primarily includes objects like
window
,navigator
, andhistory
that represent the browser’s environment.
How can I open a new browser window using JavaScript?
- Use the
window.open()
method to open a new browser window.
What is the purpose of the window.location
property?
- The
window.location
property allows you to get or set the current URL of the browser window.
How do you close a browser window with JavaScript?
- Use the
window.close()
method to close the current browser window.
Can the JavaScript Window object be used to handle cookies?
- Yes, the
document.cookie
property within the Window object can be used to manage cookies.
What is the window.navigator
object?
- The
window.navigator
object contains information about the browser, such as its version and user agent.
How can you resize a browser window using JavaScript?
- Use the
window.resizeTo(width, height)
method to resize the browser window to specified dimensions.
What are the differences between window.innerHeight
and window.outerHeight
?
window.innerHeight
measures the height of the window’s content area, whilewindow.outerHeight
includes interface elements like toolbars and scrollbars.