TL;DR
- JavaScript objects are key-value pairs that store data and functions.
- Objects can be created using object literals, constructor functions, or ES6 classes.
- Property access can be done using dot notation or bracket notation (for dynamic keys).
- Methods allow objects to perform actions using the
this
keyword to reference their properties. - Shallow copying objects can be done using the spread operator (
...
), while deep copying requiresstructuredClone()
. - By following the best practices outlined in this article and avoiding common mistakes, you can confidently master JavaScript objects and write more efficient, maintainable code.
In the realm of web development, JavaScript remains one of the most widely used programming languages. It powers interactive web pages, server-side applications, and even mobile apps through various frameworks. At the core of this language are JavaScript objects, which serve as powerful data structures enabling developers to store, manipulate, and transfer information within their applications. Understanding how objects work is important to harnessing the full potential of JavaScript.
This article explores everything you need to know about JS objects. Additionally, we’ll discuss best practices, common mistakes to avoid, and important some other concepts. By the end, you’ll have a thorough understanding of JS objects and be prepared to integrate them seamlessly into your development projects.
What Are JavaScript Objects?
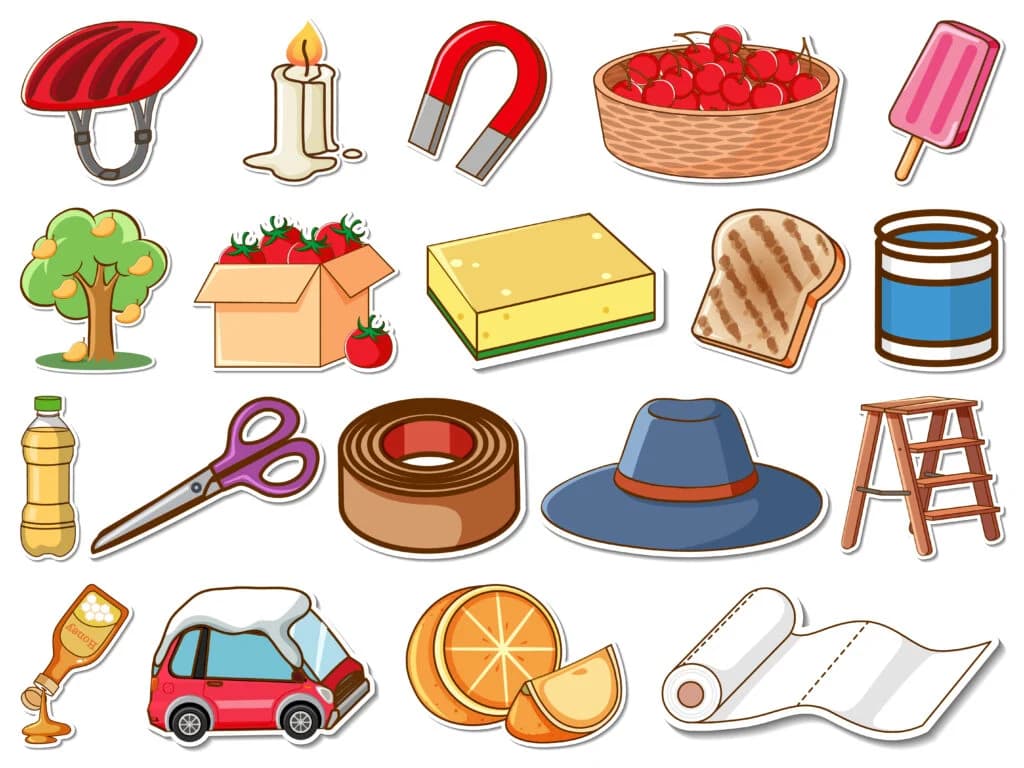
Before exploring deeper, it’s important to understand precisely what JavaScript objects are. At its core, an object in JavaScript is a collection of key-value pairs. Keys are also referred to as properties or methods. Each property within an object is associated with a value, which can be of any data type, including strings, numbers, booleans, arrays, and even other objects.
In simpler terms, If you imagine data as real-world entities, then an object is just an entity with attributes and behaviors.
For example, suppose you have a user in your system. This user can be thought of as an object with attributes like name
, age
, and email
, as well as behaviors (functions) like logIn()
or logOut()
.
Why Are JavaScript Objects Important?
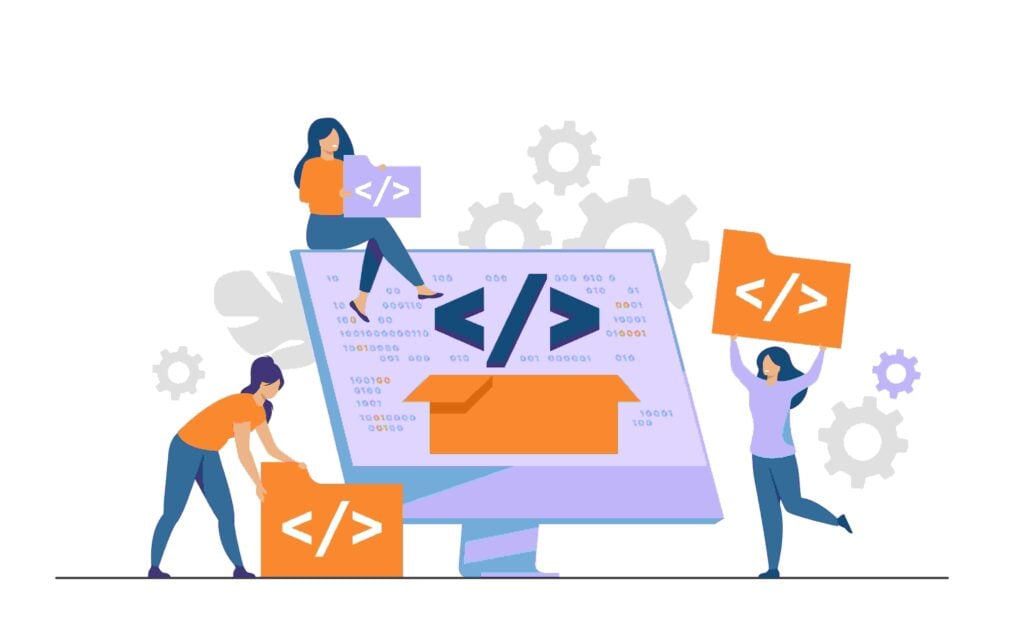
JS objects are important for several reasons. The following are some of those.
- Structure
- Objects provide a systematic way to organize and encapsulate data, preventing disorganized code and facilitating clearer, modular development.
- Flexibility
- They can contain various data types, including other objects, making them highly versatile.
- Reusability
- Objects facilitate the reuse of code, especially when paired with prototypes or ES6 classes.
- Efficient Data Handling
- Objects allow developers to handle and manipulate data in a single entity, making complex applications easier to maintain.
Modern web applications rely on object-based structures for everything from state management in frameworks like React and Vue.js to complex Node.js backends. The deeper your understanding of JavaScript objects, the more effective and efficient you’ll be when writing modern JavaScript code.
Creating Objects in JavaScript
There are multiple ways to create JavaScript objects. Let’s explore the most common methods.
1. Object Literal
Object literals are a fundamental feature in JS objects. They provide a succinct way to create and initialize an object in a single expression. An object literal consists of a list of key-value pairs inside curly braces. Each key can be defined either as a string or an identifier, and its value can be of any valid JavaScript type.
Let’s look at the following example to understand the correct syntax for creating an object using object literals.
const person = {
name: "John Doe",
age: 30
};
Here, person
is an object with two properties, name
and age
.
ES6 introduced a convenient property shorthand that allows you to omit key names in an object when they match the variable names. This makes your code cleaner and more concise, reducing redundancy while improving readability.
const title = "To Kill a Mockingbird";
const author = "Harper Lee";
const novel = {
title,
author,
publishYear: 1960
};
In the above example, title
and author
are used both as variable names and property names in the novel
object, reducing redundancy and making code easier to read.
2. Using the new Object()
Constructor
Another way to create objects in JavaScript is by using the new Object()
constructor. This approach is less commonly used than object literals but can be useful in certain scenarios.
const car = new Object();
car.brand = "Toyota";
car.model = "Camry";
car.year = 2020;
While functionally similar, the literal syntax is generally preferred due to simplicity and readability.
3. ES6 Classes
Introduced in ECMAScript 2015 (ES6), classes provide a more familiar, object-oriented syntax for creating and working with JavaScript objects. Under the hood, they still use prototypes but offer a cleaner, more readable syntax.
class Animal {
constructor(name, species) {
this.name = name;
this.species = species;
}
describe() {
console.log(`${this.name} is a ${this.species}.`);
}
}
const lion = new Animal("Leo", "Lion");
lion.describe(); // "Leo is a Lion."
Classes in JavaScript also support inheritance, enabling you to create a new class that extends a base class and inherits its properties and methods. This allows for code reusability, making it easier to manage and scale applications by building on existing functionality.
class Bird extends Animal {
constructor(name) {
super(name, "Bird");
}
fly() {
console.log(`${this.name} is flying!`);
}
}
const eagle = new Bird("Eddy");
eagle.describe(); // "Eddy is a Bird."
eagle.fly(); // "Eddy is flying!"
By using extends
, the Bird
class inherits from the Animal
class, gaining access to the describe()
method, while also being able to define its own specialized behavior like fly()
.
Accessing and Modifying Object Properties
With JavaScript objects, you can access and modify properties in two primary ways.
Using Dot Notation
Dot notation is generally the more straightforward and commonly used method for accessing or modifying properties of an object. It is simple, easy to read, and widely supported in JavaScript. By using dot notation, you can directly refer to a specific property or method of an object, making your code cleaner and more readable.
const product = { name: "Laptop", price: 1200 };
console.log(product.name); // "Laptop"
product.price = 1100;
Using Bracket Notation
Bracket notation is particularly useful when you need to access properties with dynamic names or when the property name contains special characters, spaces, or starts with a number. Unlike dot notation, bracket notation allows you to use strings as property keys, which makes it flexible and suitable for working with object properties whose names aren’t known ahead of time. This notation is essential when dealing with more complex scenarios where dot notation wouldn’t be applicable.
const product = { name: "Laptop", price: 1200 };
console.log(product["price"]); // 1200
product["price"] = 1300;
Methods in JavaScript Objects
In JS objects, a property can be a function, known as a method. Methods allow objects to encapsulate logic alongside data, creating a more robust structure.
const calculator = {
a: 5,
b: 3,
add: function() {
return this.a + this.b;
},
multiply() {
// Shorthand method declaration
return this.a * this.b;
}
};
console.log(calculator.add()); // Output: 8
console.log(calculator.multiply()); // Output: 15
In the example above, calculator.add()
and calculator.multiply()
are methods that perform operations on the properties of the calculator
object. The keyword this
is used inside these methods to refer to the current object, allowing access to its properties and enabling the methods to interact with the object’s own data.
Prototypes in JavaScript Object
Every object in JavaScript has an internal property called [[Prototype]]
. This prototype is another object from which the current object can inherit properties and methods. When you try to access a property on an object, JavaScript will look first at the object itself. If it doesn’t find it, it will search the object’s prototype, then the prototype’s prototype, and so on. This process is called the prototype chain.
The Prototype Object
Each function in JavaScript has a prototype
property, which is the object that will be assigned as the [[Prototype]]
of instances created by that function. This is how shared methods and properties are established among all object instances.
function Person(name) {
this.name = name;
}
Person.prototype.greet = function() {
console.log(`Hello, my name is ${this.name}.`);
};
const sam = new Person("Sam");
sam.greet(); // "Hello, my name is Sam."
In this example, greet()
is defined on the Person.prototype
, so all Person
instances can access the greet()
method via their prototype without duplicating the function each time.
Modifying the Prototype
You can also add properties and methods to an existing prototype at any time:
Person.prototype.sayGoodbye = function() {
console.log(`${this.name} says goodbye.`);
};
All new and existing instances of Person
will then have access to sayGoodbye()
.
Object Methods in JavaScript
Modern JavaScript provides some convenient built-in methods for working with object properties.
Object.keys(obj)
- Returns an array of the object’s own property names.
Object.values(obj)
- Returns an array of the object’s own property values.
Object.entries(obj)
- Returns an array of
[key, value]
pairs.
- Returns an array of
const fruit = {
name: "Apple",
color: "Red",
quantity: 10
};
console.log(Object.keys(fruit)); // ["name", "color", "quantity"]
console.log(Object.values(fruit)); // ["Apple", "Red", 10]
console.log(Object.entries(fruit));// [["name", "Apple"], ["color", "Red"], ["quantity", 10]]
These methods are especially helpful when you need to iterate over an object’s properties or transform object data into another format.
Common Use Cases for JavaScript Objects
JS objects are incredibly versatile and are widely used in various scenarios to represent and manage data. They allow you to store collections of related information in a structured way, making it easier to work with complex data. Below are some common use cases where JS objects prove to be extremely useful:
- Storing and Transferring Data
- Objects are often used as a convenient format for sending data across the network. They are a go-to choice for representing structured data in a readable, easily modifiable format.
- Modeling Real-World Entities
- From users to products in an e-commerce platform, JavaScript objects are the perfect representation for real-world entities. They naturally encapsulate the attributes and behaviors that define any entity.
- State Management in Front-End Frameworks
- Modern front-end frameworks like React, Vue, and Angular rely heavily on objects to manage state. By manipulating object properties, these frameworks can efficiently track changes and render components accordingly.
- Configuration and Options
- Many libraries and frameworks accept configuration objects to modify behavior. This approach allows for flexible, human-readable settings.
Best Practices When Working with JavaScript Objects
The following best practices makes sure that your code is efficient, maintainable, and less prone to errors.
Use Literals Wherever Possible | Object literals are more readable and less error-prone than using new Object() . |
Consistent Property Naming | Stick to naming conventions like camelCase for consistency and readability. |
Freeze or Seal Objects When Necessary | Use Object.freeze() or Object.seal() to prevent unintended changes when immutability is required. |
Use Descriptive Keys | Choose clear and meaningful property names to improve code readability. |
Watch Out for Name Collisions | Be cautious of dynamic property names that may override existing properties when using bracket notation. |
Common Pitfalls to avoid
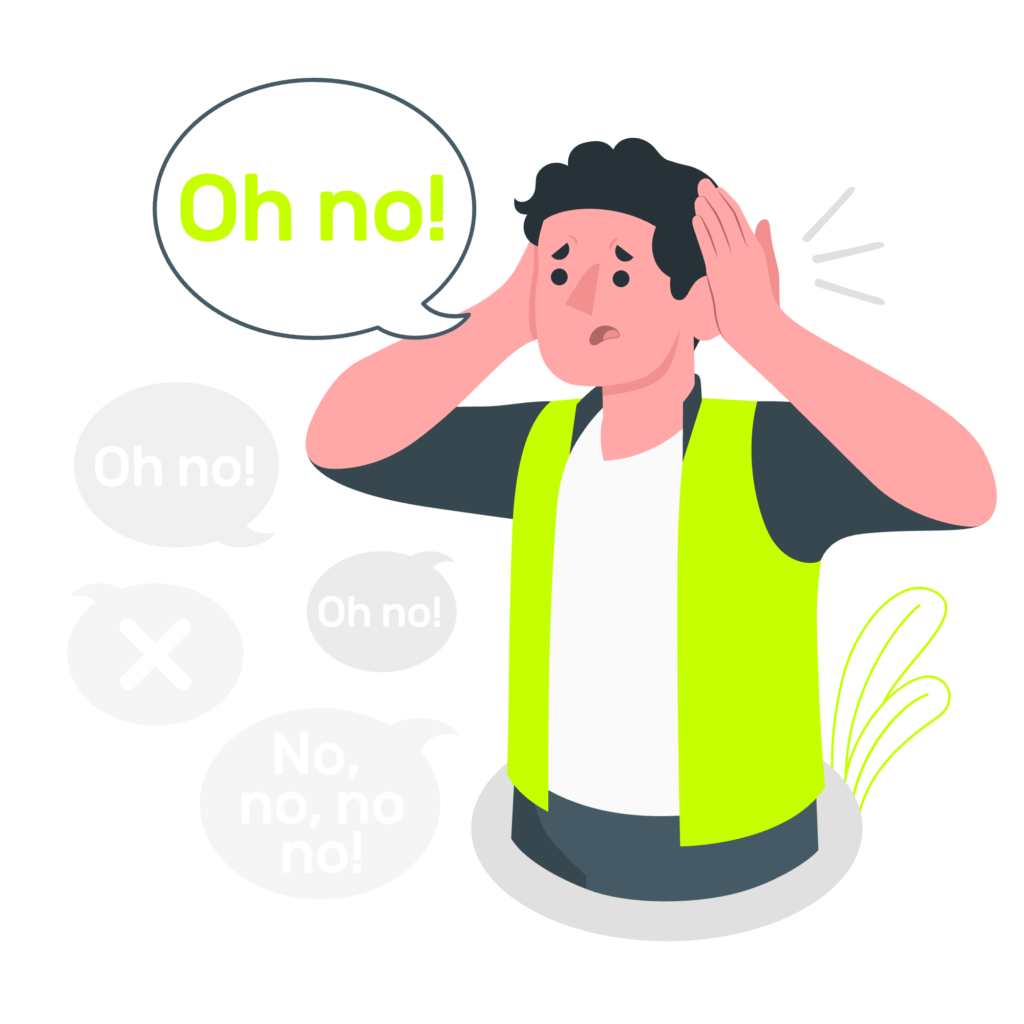
When working with JavaScript objects, it’s important to be aware of common pitfalls that can lead to unexpected behavior or errors in your code. Avoiding these mistakes ensures that your code remains clean, efficient, and bug-free. Here are some key pitfalls to watch out for:
- Accidental Global Variables
- When working with constructor functions or prototypes, forgetting to use the
new
keyword can lead to assigning properties to the global object instead of your intended object.
- When working with constructor functions or prototypes, forgetting to use the
- Misuse of
this
- The value of
this
can change depending on context. Always make sure that methods are invoked with the correct binding if you rely onthis
.
- The value of
- Prototype Pollution
- Be cautious when merging objects or working with external data, as you may inadvertently modify the prototype of an object, potentially leading to security vulnerabilities.
- Deep vs. Shallow Copying
- When copying objects, realize that a shallow copy only copies the top-level properties. Nested objects or arrays are still passed by reference. For complete isolation, use deep cloning techniques.
Conclusion
JavaScript objects are fundamental building blocks for any successful web or server-side application written in JavaScript. Their flexibility and simplicity allow developers to structure code more effectively, leading to cleaner, more maintainable projects.
Whether you’re working on a small front-end feature or building a large-scale enterprise application, completely understanding JavaScript objects will significantly elevate your programming capabilities. By applying the tips and techniques in this article, you can create robust, modular, and efficient applications that utilize objects to their fullest potential.
FAQs
How do ES6 classes differ from constructor functions?
- While both serve similar purposes, ES6 classes provide a more concise and familiar object-oriented syntax. Under the hood, classes still use prototypes. They offer features like class inheritance and improved readability compared to traditional constructor functions.
How can I copy a JavaScript object without reference?
- A common way to create a shallow copy of an object is by using the spread operator (
...
). This creates a new object, but the nested objects are still shared between the original and the copy.
const original = { a: 1, b: { c: 2 } };
const copy = { ...original };
To create a deep copy, where even nested objects are copied and not shared between the original and the copy, you can use the structuredClone()
method
const deepCopy = structuredClone(original);
With this approach, changes to a property in the deepCopy
object won’t affect the original
object, as all nested properties are copied independently.
Are JavaScript objects pass-by-reference?
- Yes. When you assign an object to a variable, the variable holds a reference to the object’s memory location. If you assign that variable to another variable, they both reference the same underlying object. This means changes through one reference will affect the object accessed by the other reference.