Imagine the early days of the Wild West. Untamed potential, a rush of opportunity, but also the risk of chaos without the proper tools. Smart contracts, the backbone of blockchain technology, share some similarities. They offer immense power but require the right approach to ensure security and efficiency. Here’s where smart contract design patterns come in – they’re your essential toolkit for building strong and reliable smart contracts, just like a trusty six-shooter for a pioneer. These pre-defined solutions for common challenges will help you write clean, secure, and efficient code, making your mark on the exciting world of blockchain development.
What are Smart Contract Design Patterns?
Think of smart contract design patterns like cooking recipes, but instead of making meals, you’re building smart contracts. These patterns are like proven guides that help you solve common problems in smart contract development efficiently and effectively. By using these “recipes,” you save time and avoid common pitfalls, ensuring your smart contracts are strong and reliable. It’s about using the wisdom of others to build something great on the blockchain.
Common Smart Contract Design Patterns
Just like any skilled craft, smart contract development benefits from a well-defined set of tools. Here, we explore some of the most prevalent design patterns that empower developers to build secure, efficient, and maintainable smart contracts, along with example code snippets in Solidity to illustrate their functionality:
Factory Pattern:
This fundamental pattern streamlines the creation of new smart contract instances. Imagine a factory churning out identical units – the Factory Pattern allows for the efficient deployment of multiple instances of your smart contract, each with its own unique state, while adhering to a consistent and pre-defined logic.
Example:
contract MyTokenFactory {
// Address of the deployed token contract
address public tokenAddress;
// Function to deploy a new MyToken instance
function createToken() public {
MyToken token = new MyToken();
tokenAddress = address(token);
}
}
contract MyToken {
// Token properties (name, symbol, etc.)
// ...
constructor() public {
// Initialization logic for the token
}
}
State Machine Pattern:
This pattern excels at managing the lifecycle of a smart contract. Think of it like the different courses of a fancy meal – each stage, from order placement to payment to delivery, represents a distinct state within the contract. The State Machine Pattern ensures a smooth and logical progression through these states, preventing unexpected transitions and errors.
Example:
enum OrderState { Created, Paid, Delivered }
contract Order {
OrderState public state;
// Function to transition to the next state
function placeOrder() public {
require(state == OrderState.Created, "Order already placed");
state = OrderState.Paid;
}
// Function to transition to the next state (only after payment)
function markDelivered() public {
require(state == OrderState.Paid, "Order not yet paid");
state = OrderState.Delivered;
}
}
Proxy Pattern:
Flexibility is key in the ever-evolving world of blockchain technology. The Proxy Pattern allows for the creation of an upgradeable layer for your smart contract. This acts like a protective barrier, safeguarding the core logic of your contract while enabling the outer layer to be updated without affecting the underlying functionality.
Example (Simplified):
contract MyContract {
// Core logic functions
// ...
}
contract MyContractProxy {
MyContract public implementation;
constructor(MyContract _implementation) public {
implementation = _implementation;
}
// Delegate calls to the implementation contract
fallback() external payable {
address impl = address(implementation);
assembly {
let ptr := mload(0x40) // Load function pointer from calldata
let result := delegatecall(gas, impl, ptr, calldatasize, 0, 0)
returndatacopy(ptr, returndatasize)
switch result
case 0 { revert(returndatasize, returndatacopy(ptr, returndatasize)) }
default { return(returndatasize) }
}
}
}
Note: This is a simplified example for illustration purposes. Real-world implementations of the Proxy Pattern involve additional complexities.
Access Control Patterns:
Security is paramount within the realm of blockchain. Access Control Patterns provide a structured approach to managing who can interact with your smart contract. These patterns establish clear boundaries, differentiating between functions restricted to the contract owner and actions accessible to the public.
Example:
contract AccessControl {
address public owner;
constructor() public {
owner = msg.sender;
}
modifier onlyOwner() {
require(msg.sender == owner, "Only owner can call this function");
_;
}
function publicFunction() public {
// Anyone can call this function
}
function onlyOwnerFunction() public onlyOwner {
// Only the contract owner can call this function
}
}
Oracle Pattern:
The power of smart contracts lies in their ability to interact with the outside world. The Oracle Pattern serves as a bridge, enabling your smart contract to retrieve external data feeds. This data, such as stock prices or weather information, can then be integrated into the logic of your contract, unlocking a wider range of functionalities.
Note: Implementing the Oracle Pattern securely involves additional considerations beyond the scope of this article.
Remember that these only few examples of the vast number of smart contract design patterns that are available.
Why You Should Use Design Patterns?
The incorporation of design patterns into your smart contract development process offers a multitude of benefits, streamlining the creation of secure, efficient, and maintainable code. Here are some key advantages to consider:
- Enhanced Efficiency: Design patterns provide pre-defined solutions for common challenges, eliminating the need to “reinvent the wheel” for each project. This translates to a significant reduction in development time, allowing you to focus on the unique aspects of your smart contract.
- Improved Readability and Maintainability: Well-established design patterns often lead to a more modular and structured codebase. This promotes clarity and organization within your smart contracts, making them easier to understand and modify for both the original developer and future collaborators. Clear and maintainable code becomes crucial as the complexity of your smart contracts grows.
- Elevated Security: By leveraging established best practices, design patterns can contribute to the creation of more robust and secure smart contracts. They can help mitigate vulnerabilities that might otherwise be introduced through custom-built solutions, reducing the potential for exploits by malicious actors.
How To Choose The Right Design Pattern?
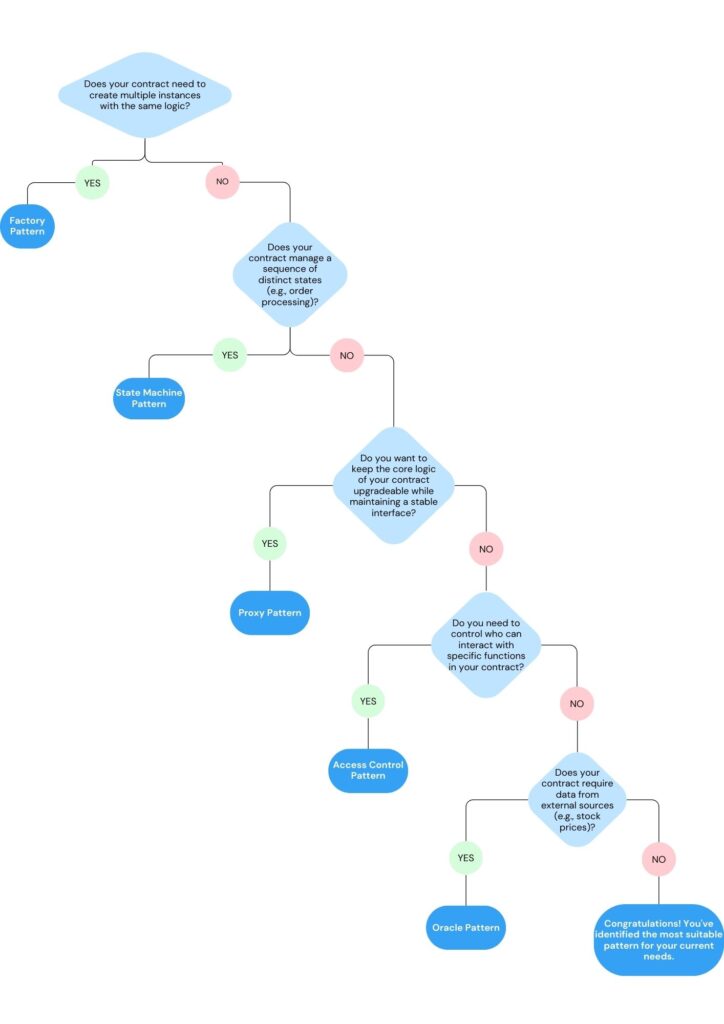
Conclusion: Level Up Your Smart Contract Skills with Design Patterns
Smart contract design patterns are like cheat codes for smart contract development! They save you time, make your code cleaner, and – most importantly – keep it secure.
Think of them as building blocks that help you construct robust and efficient smart contracts. So, grab your metaphorical toolbelt, explore these patterns further (link to resources on design patterns!), and get ready to impress everyone in the blockchain arena (or at least avoid any rookie mistakes!).
Bonus Section (For the Enthusiasts)
Now, for those who are eager to delve even deeper into the world of smart contract design patterns, let’s explore an advanced concept that’s become a cornerstone in the blockchain community.
The ERC-721 Standard: A New Frontier for NFTs
The ERC-721 standard represents a significant leap in blockchain technology, specifically in the realm of non-fungible tokens (NFTs). Unlike traditional tokens, which are interchangeable, NFTs are unique digital assets. The ERC-721 standard provides a blueprint for creating these distinct assets, offering a range of applications from digital art to ownership records. Embracing this standard means stepping into a world where each token is a masterpiece, with its own story and value.
Further Reading
To enhance your journey in mastering smart contract design patterns, here are some resources that can provide deeper insights and broader perspectives:
- Solidity Documentation: The official Solidity documentation is an invaluable resource for developers at all levels. It offers comprehensive guides, best practices, and detailed explanations of design patterns.
- Ethereum.org: Ethereum’s own website provides a wealth of information on smart contracts and design patterns, with community-contributed tutorials and examples.
- OpenZeppelin: A library for secure smart contract development. It offers implementations of common contract architectures, including ERC-721.
- GitHub Repositories: Many open-source projects on GitHub offer real-world examples of smart contract design patterns in action. Exploring these can provide practical insights and inspiration.
- Blockchain Development Blogs and Forums: Engaging with the community through blogs and forums can provide fresh perspectives, problem-solving strategies, and the latest trends in smart contract development.
By exploring these resources, you’ll not only deepen your understanding of design patterns but also stay abreast of the latest developments in the fast-evolving blockchain landscape. Whether you’re a novice or a seasoned developer, the journey through the world of smart contract design is a continuous path of learning and discovery.
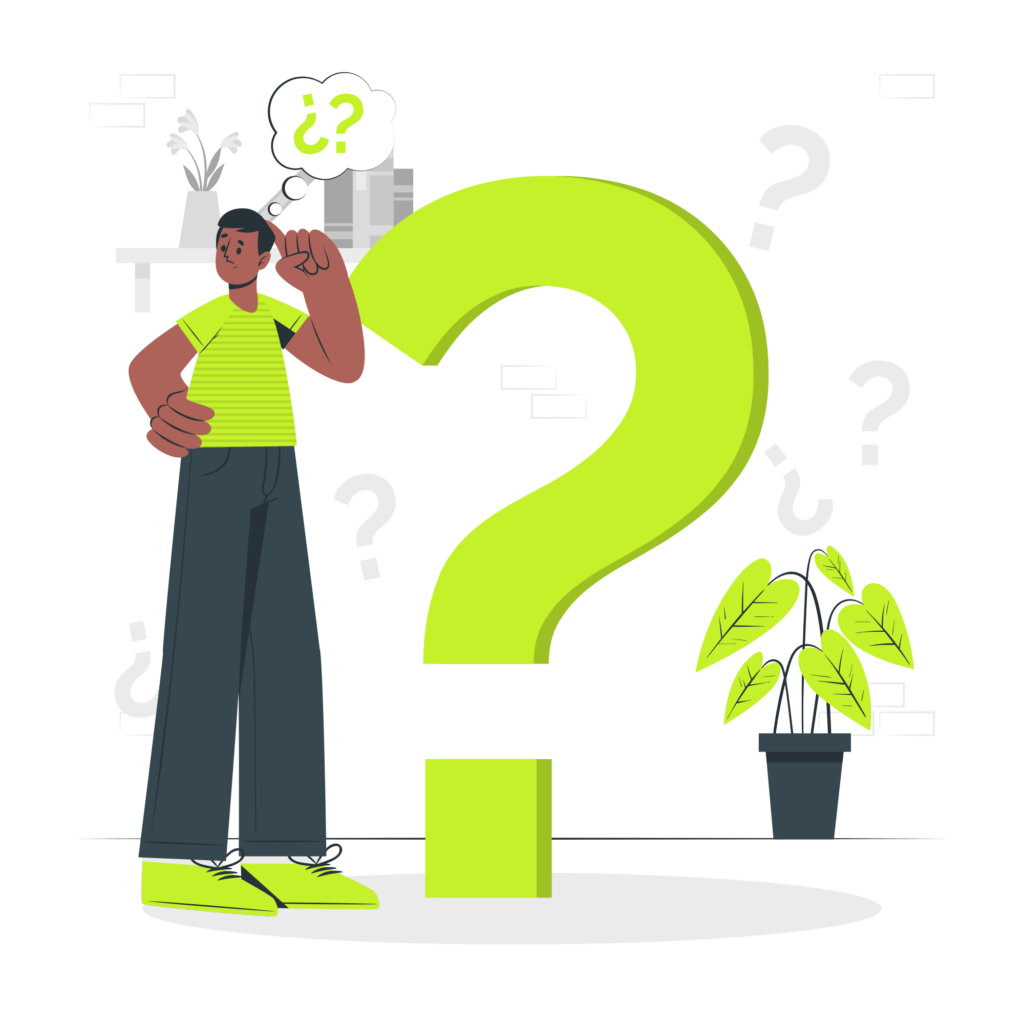
FAQs
What are smart contract design patterns?
- Smart contract design patterns are standard solutions to common problems in contract development, ensuring secure and efficient blockchain applications.
Why are design patterns important for smart contracts?
- Design patterns provide a framework for developing robust and secure smart contracts, reducing the risk of vulnerabilities and inefficiencies.
Can you name a few common smart contract design patterns?
- Common patterns include the Factory Pattern, Proxy Pattern, and Withdrawal Pattern, each addressing specific development challenges in smart contracts.
How do design patterns improve smart contract security?
- By following established best practices, design patterns help prevent common security issues, ensuring that contracts function as intended without vulnerabilities.
What resources can help me learn more about smart contract design patterns?
- Online tutorials, blockchain development courses, and community forums are great resources for learning about smart contract design patterns.
What is a smart contract in blockchain?
- A smart contract is a self-executing contract with the terms of the agreement directly written into lines of code, automatically enforced on the blockchain.
How do I get started with smart contract development?
- Start by learning a blockchain programming language like Solidity, understand the basics of smart contracts, and practice by developing simple contracts.
What are the best practices for smart contract development?
- Best practices include thorough testing, code audits, following design patterns, and staying updated with the latest security practices in blockchain development.
How can I ensure the security of my smart contracts?
- Regularly audit your contracts, follow design patterns, stay informed about common vulnerabilities, and engage with the developer community for insights.
Are there any tools to assist in smart contract design?
- Yes, there are various IDEs, testing frameworks, and security analysis tools specifically designed to assist in smart contract development.