Dive into the world of front-end development, where the magic of your favorite websites unfolds! Have you ever stopped to think about why websites grab your attention or why they’re so easy to use? That’s what we’re exploring when we ask, “What is front-end development?” It’s all about a talented front end developer, the creative geniuses and tech whizzes who shape what you see and interact with online. They’re the ones who ensure the buttons you click and the pages you scroll look great and work just right. So, come along as we get to the heart of front-end development. We’ll peek into the essential skills and tools these developers use to turn plain designs into vibrant, user-friendly web experiences. Are you ready to dive into the behind-the-scenes world that makes the web come alive? Let’s jump in!
What is Front End Development?
Front-end development involves designing and developing the user-facing part of a website or web application, ensuring it’s visually appealing, functional, and intuitive for users. When you visit a website, everything from the structure and design to the way it responds to your interactions is crafted by front-end developers. They use a mix of coding and design skills to build elements like menus, forms, and animations, making sure the site looks good and works well on various devices, from desktops to smartphones. Essentially, front-end development is about creating an engaging digital environment where users can easily find and interact with the information they need.
What are the Building Blocks of Front End Development?
Every website you encounter is meticulously crafted using a foundational triad of languages: HTML, CSS, and JavaScript. Let’s delve into their distinct roles in constructing the intricate tapestry of the web:
HTML (HyperText Markup Language):
HTML serves as the unobtrusive foundation, the unseen framework upon which all web content resides. Similar to the skeletal structure of a building, HTML defines the various sections and elements that comprise a webpage. It utilizes a system of tags to categorize content such as headings, paragraphs, images, and hyperlinks. These tags function as clear instructions for web browsers, dictating how the information should be displayed and organized, ensuring a well-structured and coherent webpage.
Sure, here are some beginner-friendly code examples you can include in your article:
Example – Basic Structure:
<!DOCTYPE <strong>html</strong>>
<html>
<head>
<title>My First Website</title>
</head>
<body>
<h1>Welcome to My Website!</h1>
<p>This is a simple example of an HTML page.</p>
</body>
</html>
Explanation:
- This code demonstrates the basic structure of an HTML document.
<!DOCTYPE html>
declares the document type as HTML.<html>
is the root element of the HTML document.<head>
contains meta information about the document, like the title.<title>
defines the title displayed on the browser tab.<body>
contains the visible content of the webpage.<h1>
defines a heading element with the largest size.<p>
defines a paragraph element.
CSS (Cascading Style Sheets):
Once the groundwork is laid by HTML, CSS assumes the role of the web’s stylist. Analogous to how a fashion designer transforms a basic garment into a visually captivating outfit, CSS governs the aesthetic presentation of a website. Using CSS, front-end developers meticulously control various visual elements, including:
- Color Palettes: Background colors, text colors, button colors, and more – establishing a cohesive color scheme to set the desired mood and tone.
- Typography: Selecting the typeface, size, and weight of the text – choosing the optimal font for readability and visual appeal.
- Layout: Arranging elements on the page to create a balanced and user-friendly experience – akin to positioning furniture within a room for optimal flow and functionality.
- Animations: Incorporating subtle animations for a touch of visual intrigue and user engagement – think of it as the finishing touches that imbue the website with a sense of dynamism.
Example – Styling the Text:
body {
font-family: Arial, sans-serif;
color: #333;
}
h1 {
text-align: center;
}
Explanation:
- This code defines styles using CSS.
body { ... }
defines styles for the entire body element.font-family: Arial, sans-serif;
sets the font family for the body text.color: #333;
sets the text color to a dark gray (#333 represents the color code).h1 { ... }
defines styles specifically for the<h1>
element.text-align: center;
centers the text within the<h1>
element.
Through the combined efforts of HTML and CSS, front-end developers can transform a basic webpage into a visually stunning and user-centric experience.
JavaScript:
The Web’s Powerhouse (Employed with Precision): JavaScript, often referred to as the engine that drives web interactivity, plays a crucial role in enhancing user engagement. It injects dynamism and interactive elements into websites, allowing for features like:
- Interactive Menus: Those sleek, dropdown menus that appear when you hover over an item? Crafted with the power of JavaScript.
- Engaging Animations: Eye-catching animations that grab your attention and enhance the user experience? JavaScript at work once again.
- Robust User Input Validation: Ever filled out a form and received an error message because you missed a required field? Thank JavaScript for ensuring you don’t make those inadvertent mistakes.
Example – Creating an Alert:
<script>
alert("Hello, world!");
</script>
Explanation:
- This code demonstrates a simple JavaScript action that displays an alert message in the browser.
<script>
tags enclose JavaScript code within an HTML document.alert("Hello, world!");
calls the built-inalert()
function, which displays a pop-up message with the text “Hello, world!”.
Example – Changing Text Color on Click:
<p id="myText">Click me to change my color!</p>
<script>
document.getElementById("myText").addEventListener("click", function() {
this.style.color = "blue";
});
</script>
Explanation:
- This code demonstrates adding interactivity to a webpage using JavaScript.
<p id="myText">
creates a paragraph element with the ID “myText”.document.getElementById("myText")
selects that paragraph element using JavaScript.addEventListener("click", ...)
attaches an event listener to the paragraph, listening for a “click” event.this.style.color = "blue";
changes the text color to blue when the paragraph is clicked.
However, it’s important to acknowledge that with great power comes complexity. JavaScript can be more challenging to master compared to HTML and CSS. It has its intricacies and occasional moments that can perplex even seasoned developers. But when wielded with precision, JavaScript can elevate a website from static to spectacular, fostering a truly immersive and engaging user experience.
Tools and Techniques for a Front End Developer
Frameworks:
A framework is a comprehensive collection of pre-written code that developers can use to build the structure of a website or web application. It provides a foundation and set of guidelines that you can build upon and customize.
- Examples:
- Angular: Developed by Google, Angular is a powerful framework that allows developers to create dynamic, single-page applications with rich features and functionality.
- React: Created by Facebook, React is a library but often referred to as a framework due to its extensive ecosystem and capabilities. It’s known for its efficient update mechanism and is widely used for building interactive user interfaces.
- Vue.js: This is a progressive framework that is particularly admired for its simplicity and its fine balance between offering a rich feature set and maintaining a lightweight footprint.
- Benefits:
- Streamlines the development process by providing a structured approach.
- Encourages code reusability, which can significantly reduce development time and ensure consistency.
- Often comes with community support, providing access to a wealth of knowledge and resources.
Libraries:
A library is a collection of reusable code snippets and components that developers can incorporate into their projects as needed. Unlike frameworks, libraries offer more freedom and flexibility, as developers can pick and choose which features to use without adhering to a strict structure.
- Examples:
- jQuery: One of the oldest and most popular libraries, jQuery simplifies HTML document traversing, event handling, and animating, making it easier to develop interactive web pages.
- D3.js: This library is specifically focused on bringing data to life using HTML, SVG, and CSS. It’s an excellent tool for creating complex data visualizations.
- Lodash: A modern JavaScript utility library delivering modularity, performance, & extras, Lodash makes JavaScript easier by taking the hassle out of working with arrays, numbers, objects, strings, etc.
- Benefits:
- Provides specific functionality without the need to reinvent the wheel.
- Offers flexibility to use only what you need, avoiding unnecessary bloat in your project.
- Can significantly enhance productivity by providing well-tested modules and functions that speed up development.
In summary, frameworks and libraries are indispensable tools in the front end developer’s toolbox. They not only streamline the development process but also enable developers to build more complex, interactive, and responsive websites efficiently.
Responsiveness:
Let’s take a look at Metana’s website on a PC and a Mobile phone.
On a PC:
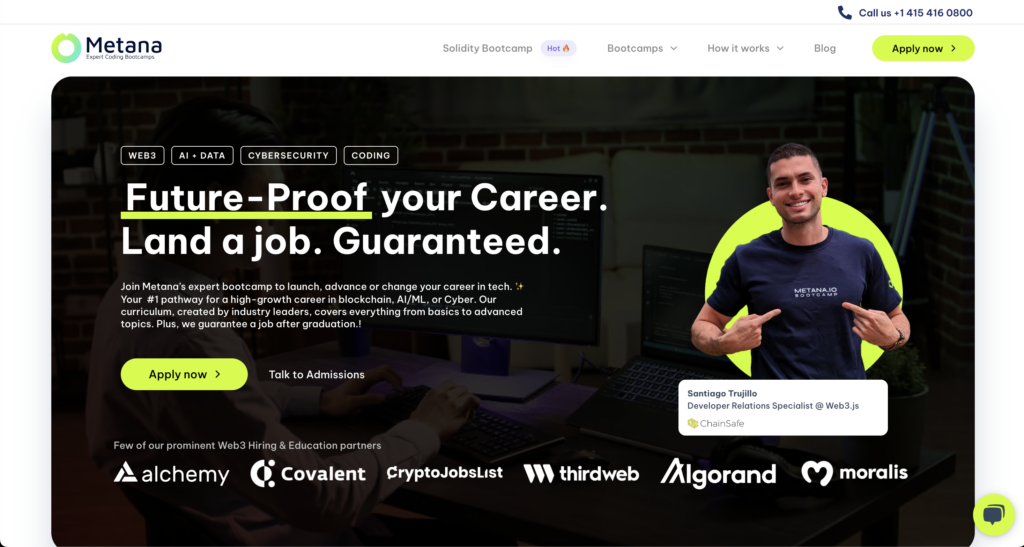
On a mobile phone:
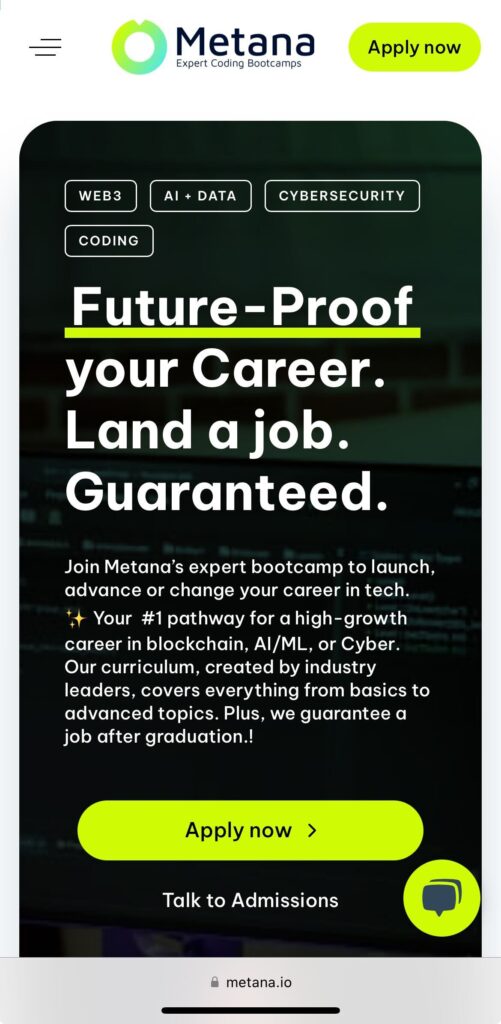
As you can see all the elements that are seen when it’s shown through a PC are right there on the mobile phone view. Nicely placed at a given space. This is what we basically call the responsiveness. Let’s explore deeper in to that concept.
Adaptable Layouts:
Responsive design means that a website’s layout adjusts based on the screen size and orientation of the device it’s being viewed on. This adaptability ensures that whether a user is on a desktop, tablet, or smartphone, the website’s content is always readable and accessible.
For example, a three-column layout on a desktop might stack into a single column on a mobile device, making it easier to scroll through on a smaller screen.
Flexible Images and Media:
In a responsive design, images and media are also flexible. They scale in size or change aspect ratios to fit different screen sizes without losing quality or becoming distorted.
This means an image that fills the screen width on a mobile phone will shrink proportionally for larger screens, ensuring it looks good and fits well within the page’s structure.
Media Queries:
One of the technical tools behind responsive design is the use of CSS media queries. These allow developers to apply different styles based on the characteristics of the device, such as its width, height, orientation, and resolution.
Media queries enable a website to present a custom, optimized layout across various devices, enhancing user experience and engagement.
User-Friendly Navigation:
Responsive design also involves rethinking navigation to ensure it’s user-friendly on different devices. A menu that’s easy to use on a desktop might need a different approach for a touchscreen device.
Dropdown menus might be converted into off-canvas menus or touch-friendly dropdowns to accommodate the different ways users interact with mobile devices versus desktops.
Testing and Optimization:
Ensuring a website is truly responsive involves thorough testing on multiple devices and screen sizes. Developers often use emulators and real devices to test and tweak designs, ensuring that the website is not just functional but also intuitive and easy to navigate on any device.
Continuous optimization is key, as new devices and screen sizes emerge, requiring ongoing adjustments to maintain an optimal user experience.
Conclusion
Front-end development unveils itself as a captivating field, meticulously crafting user-centric websites. By wielding HTML, CSS, and JavaScript, front-end developers act as the invisible architects of the web. They define structure (HTML), design aesthetics (CSS), and inject interactivity (JavaScript), all to ensure a seamless user experience.
This exploration has hopefully provided a springboard into the core technologies of front-end development. The journey, however, is far from over. For the curious, the world of full-stack development beckons, offering mastery over both front-end and back-end aspects (but that’s a tale for another time).
Continue your exploration! Dive into the resources provided (or visit our social media for more development content) and embark on your exciting front-end development adventure!
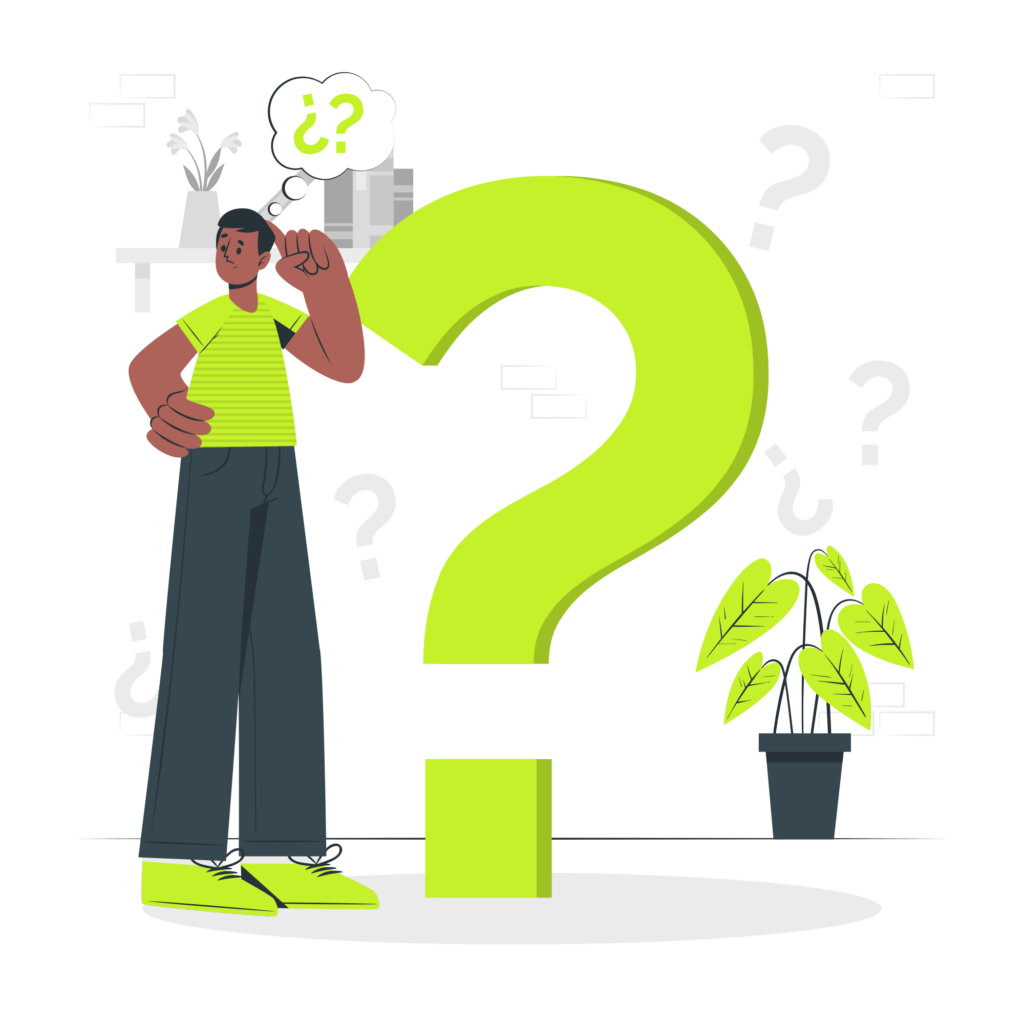
FAQs
What is front-end development?
- Front-end development is the practice of using HTML, CSS, and JavaScript to create the visual and interactive aspects of a website or web application that users interact with directly.
Which languages are primarily used in front-end development?
- The core languages used in front-end development are HTML (for structure), CSS (for styling), and JavaScript (for interactivity).
How does front-end development differ from back-end development?
- Front-end development focuses on the user interface and experience, while back-end development deals with the server, database, and application logic.
Why is responsive design important in front-end development?
- Responsive design ensures that a website is accessible and user-friendly across various devices and screen sizes, enhancing user experience.
What are some common tools and frameworks used in front-end development?
- Popular tools and frameworks include React, Angular, Vue.js, Bootstrap, and jQuery, which help developers build robust and dynamic interfaces.
What is web development?
- Web development encompasses all the tasks associated with developing websites for hosting via intranet or internet, including front-end and back-end development.
What is the role of a web designer in front-end development?
- A web designer focuses on the look and feel of a website, often working closely with front-end developers to ensure the design translates well into a functional user interface.
How important is accessibility in front-end development?
- Accessibility ensures that all users, including those with disabilities, can access and interact with websites, making it a crucial aspect of front-end development.
What is user experience (UX) design in the context of front-end development?
- UX design focuses on optimizing the user’s experience on a website, ensuring it’s intuitive, engaging, and easy to navigate.
How do front-end developers optimize websites for performance?
- Front-end developers optimize websites by minimizing code, optimizing images, and utilizing techniques like lazy loading to improve loading times and overall performance.