Imagine a world full of bugs and weird happenings, but we’re not talking about bugs you find under a rock—we’re diving into the cool world of checking smart contracts for mistakes, called solidity mutation testing. This special testing is like a superhero for your Ethereum smart contracts, making sure they are strong, super safe, and work perfectly. Think of it as giving your contract a superhero cape to fly high in the world of cryptocurrency. Understanding this smart trick can be a bit tough, but don’t worry! , Let’s explore how this special testing can be a game-changer for your blockchain projects. It’s way more exciting than regular testing and a must-have tool for anyone making smart contracts.
What is Mutation Testing?
Imagine you’re typing away, coding your next masterpiece, but oops—you accidentally typed “>” instead of “<“. This tiny typo, or “mutation”, could change the whole game. Mutation testing is like hiring a personal trainer for your tests, deliberately introducing these typos into your code to check if your tests can spot the difference. It’s a rigorous workout regime designed to make your tests stronger, faster, and more resilient.
How Does Solidity Mutation Testing Work?
Imagine you have a simple smart contract in Solidity designed to store and retrieve a user’s age. Here’s a basic example:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract AgeStore {
uint256 private age;
function setAge(uint256 _age) public {
age = _age;
}
function getAge() public view returns (uint256) {
return age;
}
}
Now, let’s talk about how we’d apply mutation testing to this contract to ensure our tests are robust.
Step 1: Creating Mutants
Mutants are versions of your code with small, deliberate errors introduced. For our AgeStore
contract, a mutant might involve changing the equality operator in a conditional statement, though our current example doesn’t have one. To make it applicable, let’s assume we have a condition for setting age:
function setAge(uint256 _age) public {
if(_age > 18) {
age = _age;
}
}
A mutant for this might change the condition to incorrectly allow setting age only if _age
is less than or equal to 18:
function setAge(uint256 _age) public {
if(_age <= 18) { // Mutated line
age = _age;
}
}
Step 2: Running Tests Against Mutants
Assume you have a test case that checks whether the age is set correctly when a valid age is provided. With mutation testing, you run this test against both the original and mutated contract code.
Original test (simplified for illustration):
it("should set age correctly", async function () {
let instance = await AgeStore.deployed();
await instance.setAge(25);
let storedAge = await instance.getAge();
assert.equal(storedAge, 25, "The age was not stored correctly");
});
When run against the original contract, this test should pass, confirming that the age is set and retrieved as expected.
Step 3: Evaluating the Results
When you run the same test against the mutated contract, it should fail because the condition has been altered to only set age if _age
is less than or equal to 18. If the test still passes, it indicates a flaw in the test—it’s not effectively detecting the introduced error.
The goal of mutation testing is to ensure your tests can fail when the contract logic is incorrect. If your tests pass even when mutants are introduced, it suggests that your test suite might not be comprehensive enough and could miss detecting real bugs.
Mutation testing in Solidity is like giving your smart contracts a thorough workout to ensure they’re in top shape. By introducing and testing against mutants, you can identify weaknesses in your test suite and strengthen it against potential bugs. This process helps build more secure, reliable smart contracts for the Ethereum blockchain, turning your contracts into digital Fort Knoxes.
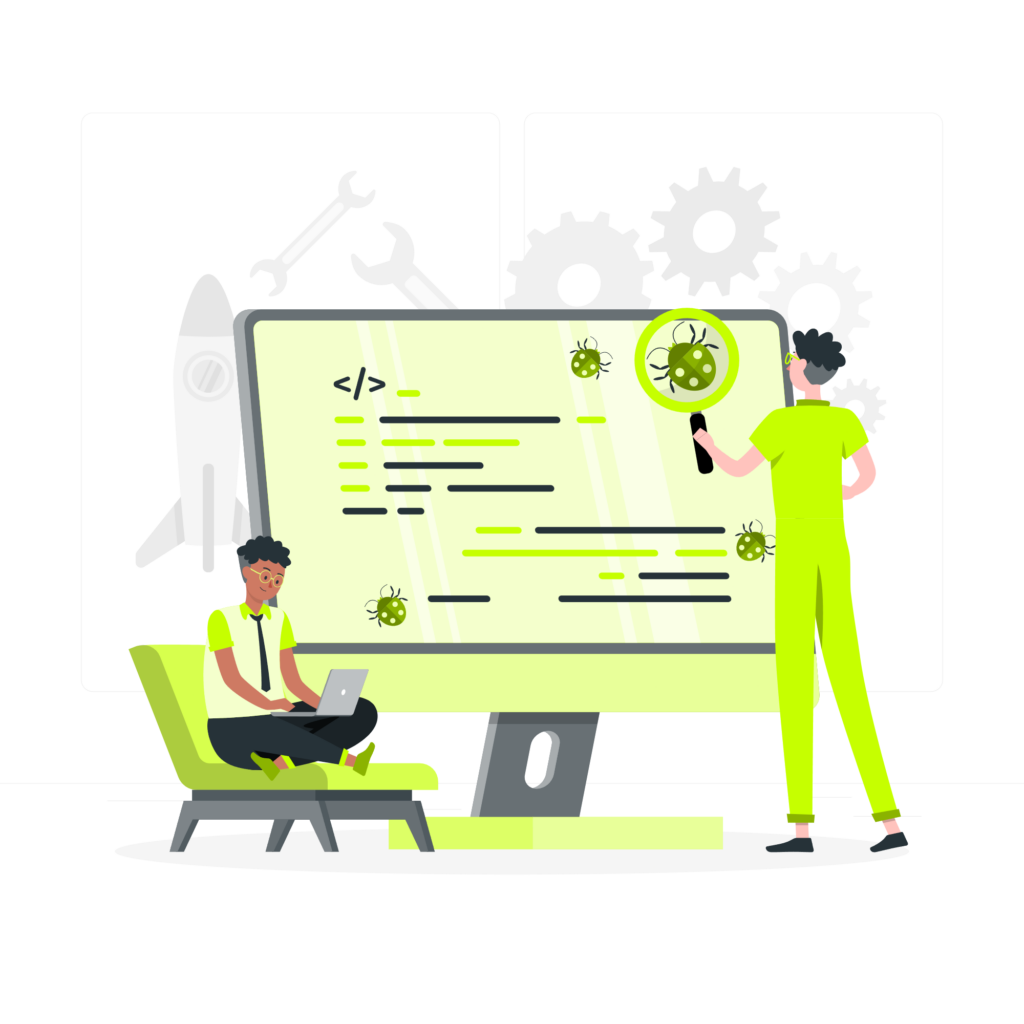
Types of Mutants
In the world of mutation testing, “mutants” are variations of the original code created by deliberately introducing small changes. These mutants serve as a test to ensure that your testing suite is robust and comprehensive. There are several types of mutants that can be generated to test different aspects of your code’s resilience. Understanding these types can help you better grasp the scope of mutation testing and its importance in developing secure, reliable software. Here’s a breakdown of some common types of mutants:
1. Value Mutants
Value mutants are created by changing the literals in the code. For instance, numerical values might be incremented or decremented, boolean values might be flipped, or string literals could be altered. This type of mutation tests whether the code can handle unexpected or incorrect values gracefully and whether the tests are sensitive to value changes.
Example:
Original Code: if (balance > 100)
Value Mutant: if (balance > 101)
2. Decision Mutants
Decision mutants modify the logical decisions in the code, such as changing conditional operators. This can involve altering comparison operators (<
, >
, ==
, etc.) or logical operators (&&
, ||
, !
, etc.). Decision mutants evaluate the effectiveness of tests in covering different logical paths through the code.
Example:
Original Code: if (userRole == "admin" && isActive)
Decision Mutant: if (userRole == "admin" || isActive)
3. Statement Mutants
These mutants introduce changes by removing or altering statements in the code. This could involve deleting a line of code, changing the sequence of statements, or replacing a statement with another. Statement mutants help assess the completeness of test cases in detecting missing or incorrect steps in the code logic.
Example:
Original Code:
user.balance += amount;
emit BalanceUpdated(user.balance);
Statement Mutant:
emit BalanceUpdated(user.balance);
4. Arithmetic Mutants
Arithmetic mutants are generated by modifying arithmetic operations within the code. This includes changing addition to subtraction, multiplying instead of dividing, and vice versa. These mutants test if the logic of mathematical calculations is correctly validated by the test suite.
Example:
Original Code: total = price * quantity
Arithmetic Mutant: total = price / quantity
5. Function/Method Call Mutants
This type involves altering the function or method calls. It could be changing the function being called, modifying the arguments passed to a function, or removing the function call entirely. These mutants check whether the tests are effectively verifying the integration and interaction between different parts of the code.
Example:
Original Code: calculateTotal(price, quantity)
Function/Method Call Mutant: calculateTotal(price, quantity, discount)
Each type of mutant targets different aspects of your code and tests, challenging them to catch a wide range of potential errors. By understanding and utilizing these various mutants, developers can ensure their testing suite is comprehensive, thereby improving the quality and reliability of their software.
Popular Mutation Testing Tools for Solidity
1. Mythril:
- Strengths: Offers a comprehensive suite of security analysis features, including mutation testing, symbolic execution, and taint analysis. Provides detailed reports and visualizations to facilitate vulnerability identification and remediation.
- Considerations: Utilizing Mythril’s advanced features effectively necessitates a moderate level of technical knowledge. Therefore, it might not be the most user-friendly option for beginners.
2. SmartContractTester:
- Strengths: Specializes exclusively in mutation testing. Features a user-friendly interface and delivers detailed reports on test coverage and effectiveness. Additionally, it supports various mutation operators and provides customization options for advanced users.
- Considerations: Primarily focuses on mutation testing, lacking the broader security analysis capabilities offered by tools like Mythril. Furthermore, it may have a smaller community and less extensive documentation compared to other options.
3. Drizzle:
- Strengths: Presents a versatile and customizable testing framework encompassing mutation testing alongside functionalities like unit testing and integration testing. Seamlessly integrates with popular Solidity development environments and provides thorough documentation and extensive community support.
- Considerations: While Drizzle offers mutation testing capabilities, they might not be as advanced as dedicated tools like SmartContractTester. Additionally, effective utilization of Drizzle requires familiarity with the framework itself.
4. Solium:
- Strengths: Open-source tool offering mutation testing capabilities alongside linting and static analysis features. Integrates with popular build tools and provides customizable mutation operators.
- Considerations: Primarily focused on code quality and security, with mutation testing functionality being a recent addition. May require familiarity with the command line interface for effective use.
5. Oyente:
- Strengths: Open-source security analysis tool offering various functionalities, including symbolic execution, taint analysis, and mutation testing. Provides detailed reports and visualizations to aid in vulnerability identification.
- Considerations: Requires a moderate level of technical knowledge to navigate the advanced features effectively. May not be the most user-friendly option for beginners, similar to Mythril.
6. Manticore:
- Strengths: Commercial, comprehensive security analysis platform offering mutation testing alongside other functionalities like symbolic execution and fuzzing. Provides advanced features like automated vulnerability repair suggestions.
- Considerations: Requires a paid subscription to access the full suite of features, including mutation testing. Might not be the most suitable option for individual developers or small projects due to the pricing model.
This comparative analysis highlights the key features and considerations associated with several prominent mutation testing tools. It is essential to remember that the selection of the most suitable tool depends on individual needs, technical expertise, and project requirements. Developers should continuously research and evaluate the evolving landscape of blockchain development tools to stay informed about the latest advancements in mutation testing for Solidity development.
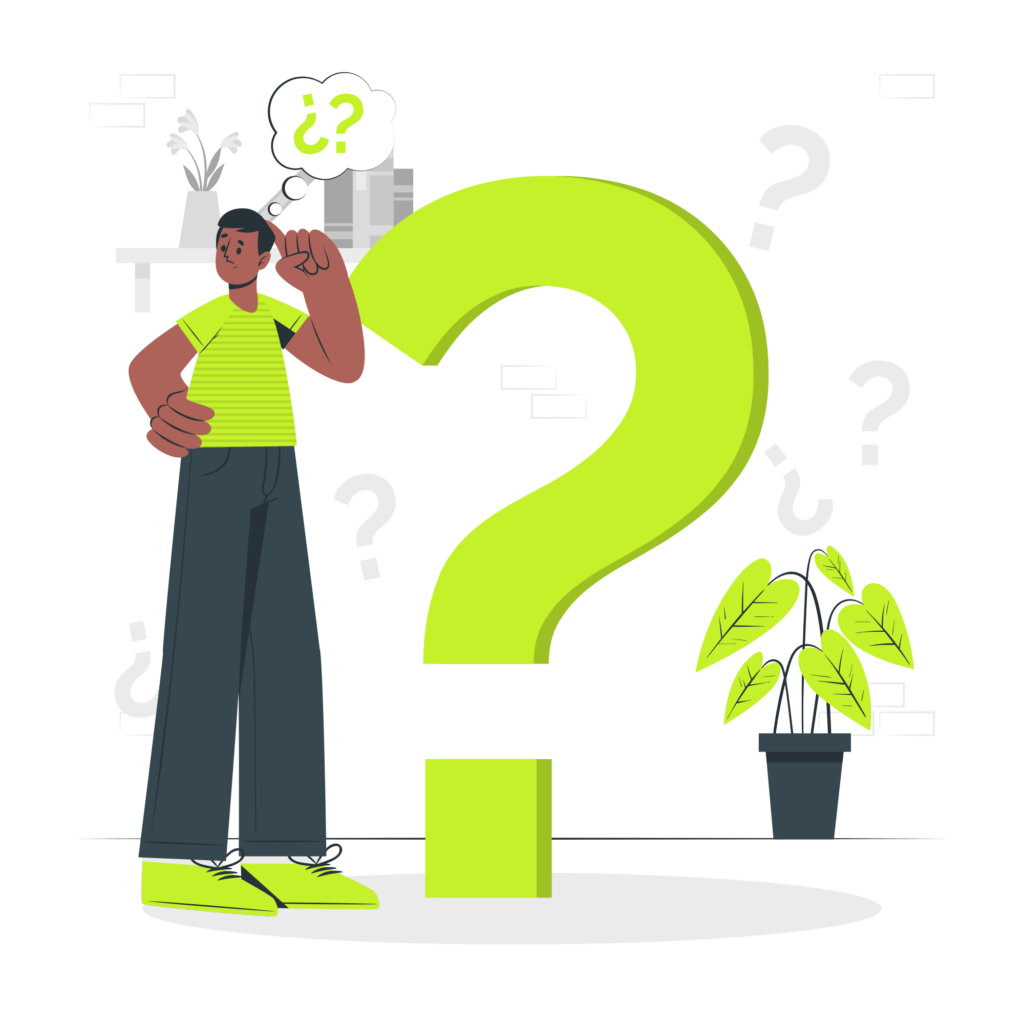
FAQs
What is Solidity mutation testing?
- Solidity mutation testing involves modifying certain aspects of the smart contract code to test the robustness of the contract’s test suite, ensuring that it can detect errors and vulnerabilities effectively.
Why is mutation testing important for Solidity smart contracts?
- Mutation testing ensures the reliability and security of smart contracts by verifying that the test suite can catch potential bugs, enhancing the quality of DApp development.
How does mutation testing improve smart contract development?
- It identifies weaknesses in test cases and coverage, pushing developers to write more comprehensive tests, thereby improving code quality and contract reliability.
What tools are available for Solidity mutation testing?
- Tools like Mutant and Stryker offer frameworks for applying mutation testing to Solidity code, allowing developers to assess the effectiveness of their tests.
Can mutation testing be automated in Solidity development?
- Yes, several tools and frameworks enable the automation of mutation testing in the development process, streamlining the identification of untested code paths.
What are the best practices for testing Solidity smart contracts?
- Best practices include writing comprehensive unit and integration tests, employing static analysis tools, conducting thorough security audits, and utilizing mutation testing to ensure code reliability.
How does continuous integration (CI) benefit Solidity development?
- CI enables automatic testing and deployment of smart contracts, ensuring that changes are reliable and secure before integration into the main codebase, enhancing development efficiency.
What role does security auditing play in smart contract development?
- Security audits are critical in identifying vulnerabilities and ensuring the integrity and safety of smart contracts before deployment, preventing potential exploits.
How can developers ensure the scalability of their DApps?
- By optimizing contract code, efficient gas usage, leveraging layer-2 solutions, and conducting load testing, developers can enhance DApp scalability.
What are the challenges in smart contract testing?
- Challenges include dealing with the immutable nature of contracts, estimating gas costs, simulating real-world interactions, and ensuring comprehensive test coverage to catch all potential vulnerabilities.