Ever found yourself staring at your computer screen, wondering if Solidity has a personal vendetta against you? You’re not alone. Picture this: It’s 2 AM, you’ve been coding for hours, and you’re just about to deploy your masterpiece when—bam!—Solidity throws a runtime error faster than you can say “blockchain.” It’s like the compiler is saying, “Nice try, but you’re not getting any sleep tonight.”
Runtime errors in Solidity are like those plot twists in movies you never see coming but are crucial to the story. They occur while your smart contract is running on the Ethereum Virtual Machine (EVM) and can be a real headache if you don’t know what you’re looking for. Understanding these errors is key to avoiding them, debugging more efficiently, and ultimately, writing contracts that are as solid as the platform’s name suggests.
Learning about Solidity runtime errors is your ticket to smoother smart contract development. It’s like learning to dodge potholes on a road you travel every day. The journey becomes much smoother.
Types of Runtime Errors
Runtime errors in Solidity can crash your party in various ways. Let’s break them down:
Arithmetic Errors
- Integer Overflow/Underflow: Imagine counting avocados, but your counter only goes to 10. Try stuffing in an 11th one, and… BOOM! Overflow error. Same for negative numbers.
- Tip: Use uint256 for large numbers or consider libraries for safe math operations.
uint8 avocados = 10;
avocados++; // This works!
avocados++; // But this overflows, setting avocados to 0!
- Division by Zero: This one’s pretty self-explanatory, like trying to divide your guac recipe by the number of friends who actually showed up. Tip: Always check for zero before dividing!
uint256 pricePerAvocado = 10;
uint256 buyers = 0;
uint256 pricePerBuyer = pricePerAvocado / buyers; // Error: division by zero!
Type Errors
- Array Out-of-Bounds: Think of an array as a shelf with numbered slots. Grab something from slot 5… no problem. But reach for slot 11 on a 10-slot shelf? That’s an out-of-bounds error. Tip: Use array bounds checks or libraries for safer array access.
uint256[] avocadoStock = [10, 5, 2];
uint256 nonExistentStock = avocadoStock[11]; // Error: index out of bounds!
- Type Mismatch: Imagine trying to pay for avocados with, say, a signed message instead of Ether. The cashier (or in this case, the blockchain) won’t be happy.
- Tip: Double-check variable types and use clear variable names.
address payable seller = 0x...;
string payment = "I promise I'll pay you soon!"; // Error: wrong data type for payment!
seller.transfer(payment);
Logic Errors
- Infinite Loops: Imagine getting stuck in an endless loop at the avocado stand, forever asking “Do you have avocados?” This can happen in code too, draining gas and causing frustration. Tip: Use clear logic structures and avoid unbounded loops.
while (true) { // This loop never ends!
// Do something (but not something that ever makes the loop stop)
}
- Gas Limit Exceeded: Think of gas as the fuel for your smart contract transaction. Run out, and it sputters to a halt. Tip: Optimize your code, estimate gas costs, and set appropriate gas limits.
// Complex and computationally expensive calculation here
// Runs out of gas before finishing!
Reentrancy Vulnerabilities
Ah, the villain of our story. Reentrancy is when an attacker repeatedly calls a function, draining funds. Remember the DAO hack? Exactly.
Example: Simplified, but imagine a function that sends Ether and doesn’t update its state until after the send.
Tip: Use the Checks-Effects-Interactions pattern to prevent reentrancy.
For each of these spooky errors, tools like Slither or Mythril can be your ghostbusters, helping detect them before they haunt your code.
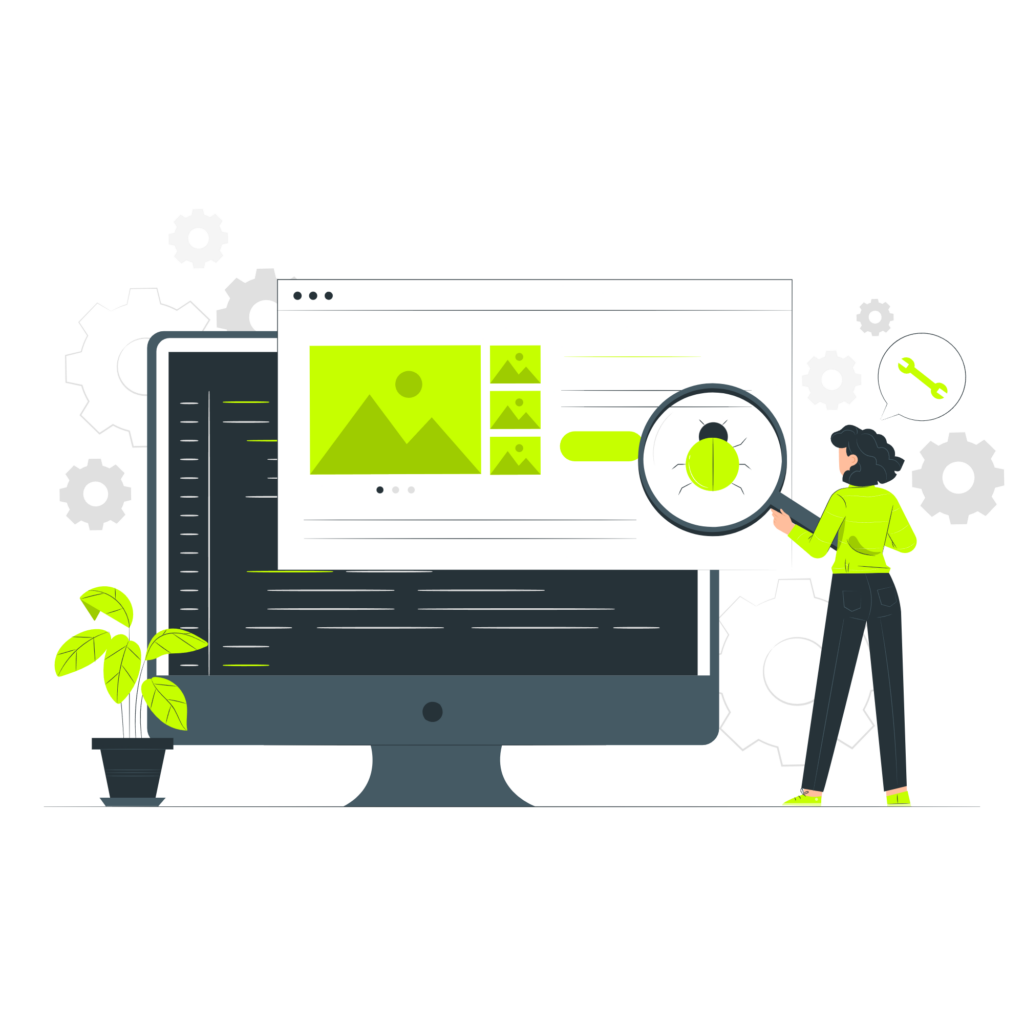
Debugging Solidity Runtime Errors
Okay, brave Solidity warriors, we’ve identified the runtime error culprits. Now, let’s arm ourselves with debugging techniques to vanquish them!
Debugging in a nutshell:
Imagine your contract as a mysterious black box. Errors are cryptic messages coming out. Debugging involves analyzing these messages, peeking inside the box (using tools), and understanding the logic flow to pinpoint the error’s origin.
Tips and Tricks for Debugging Prowess:
- Logging Like a Lumberjack: Add strategic
log
statements throughout your code, printing variable values and execution steps. These breadcrumbs will guide you to the error’s source.
uint256 balanceBefore = address(this).balance;
// Function logic here
uint256 balanceAfter = address(this).balance;
log("Balance difference:", balanceAfter - balanceBefore);
- Remix to the Rescue: This user-friendly IDE allows you to deploy, interact with, and debug your contracts in real-time. Step through code execution, inspect variables, and identify the error’s footprint.
- Gas Cost Awareness: Remember the gas limit exceeded error? Understanding gas costs and optimizing your code is crucial. Tools like Remix and online gas calculators can help you estimate and optimize gas usage.
Remember: Debugging is an iterative process. Don’t get discouraged by the initial confusion. Each error is a learning opportunity, honing your problem-solving skills and making you a more resilient developer.
Bonus Tip: Join online communities and forums. Share your debugging struggles and learn from others’ experiences. The blockchain community is full of helpful minds eager to assist!
So, go forth, intrepid developers! Embrace the challenge, wield your debugging tools, and conquer those runtime errors. Keep in mind, with each error vanquished, you become a stronger, wiser Solidity master!
Conclusion
Solidity runtime errors can be daunting, but they’re also a golden opportunity to learn and improve. By understanding the types of errors, how to avoid them, and how to debug when things go south, you’re on your way to becoming a Solidity samurai.
If you’ve battled with a runtime error that made you want to pull your hair out, I’d love to hear about it in the comments. Or, if you have questions, ask away! Sharing is caring in the developer world.
And remember, every error is just a step on the path to mastery. Happy coding. 🌟
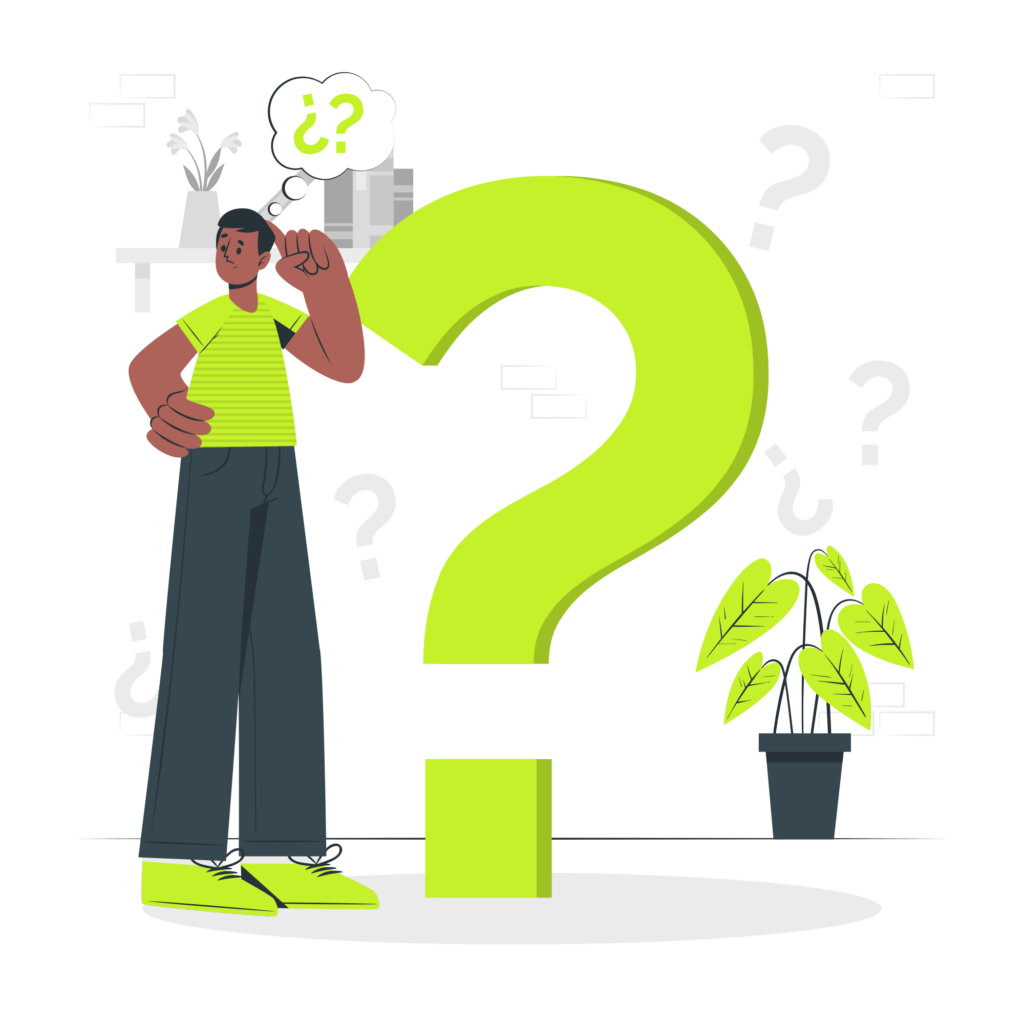
FAQs
What are Solidity runtime errors?
- Solidity runtime errors occur during the execution of smart contracts on the Ethereum blockchain, indicating issues like out-of-gas, division by zero, or invalid calls.
How can I debug Solidity runtime errors?
- Use tools like Remix, Truffle, or Hardhat for debugging. Adding
console.log
statements and analyzing transaction revert reasons can also help identify the issue.
What is a ‘revert’ error in Solidity, and how do I handle it?
- A ‘revert’ error undoes all changes made to the state during a transaction due to an exception. Handling it involves checking conditions and ensuring calls are valid before execution.
Can runtime errors affect deployed contracts on the Ethereum network?
- Yes, runtime errors in deployed contracts can lead to failed transactions or vulnerabilities. It’s crucial to thoroughly test contracts in test environments before deployment.
What are some common causes of out-of-gas errors in Solidity?
- Common causes include infinite loops, excessive computation, or large amounts of data storage. Optimizing code and setting appropriate gas limits can mitigate these issues.
What is gas optimization in Solidity, and why is it important?
- Gas optimization involves writing efficient code to reduce the gas cost of transactions, crucial for minimizing user fees and enhancing contract performance on the blockchain.
How can I ensure my Solidity smart contracts are secure?
- Conduct thorough testing, utilize static analysis tools, and consider audits by security professionals to identify and mitigate potential vulnerabilities.
What is the difference between compile-time and runtime errors in Solidity?
- Compile-time errors are detected during the compilation process, while runtime errors occur during contract execution on the blockchain.
Why is it important to handle errors properly in smart contracts?
- Proper error handling prevents contracts from executing unintended actions, enhances security, and ensures a better user experience by providing clear feedback.
What are best practices for testing Solidity contracts?
- Best practices include writing comprehensive unit and integration tests, leveraging test networks, and employing testing frameworks like Truffle or Hardhat to simulate contract interaction.