Solidity is the most popular programming language for developing smart contracts on Ethereum. And just like in any programming language, Solidity variables play a crucial role in defining the state and behavior of these smart contracts. They enable developers to store and manipulate data, making it essential to have a comprehensive understanding of the various variable types and their uses in Solidity.
What are Solidity Variables?
In Solidity, a variable is a container for storing data. These variables can represent a wide range of data types, from simple numbers to complex structures. Solidity variables are crucial for defining the state of a contract and are used to store values that are manipulated during the contract’s execution.
Solidity supports both value types and reference types, each with its specific use cases and behavior. Let’s delve into these types and explore their uses.
What are the Data Types?
In the majority of programming languages, you’ll find to types of data, those that have a value, and those that hold a reference.
Value types are basic data types that store their data directly. They are copied when assigned to another variable or passed as function arguments. The primary value types in Solidity include:
- uint: Unsigned integer types. These types are used to represent a positive integer or natural number. The keyword uint is a shorthand for uint256 which is a number that can range between 0 and 2256-1 . Other uint types include uint8, uint16, uint40…
- int: Signed integer types. Similar to unsigned integers but these are normal integers that can be positive or negative.
- bool: Boolean values that are used to declare whether a variable is true or false.
- address: This is a type unique to Ethereum and Solidity. It’s used to store the addresses of users or other contracts.
- enum: User-defined types for creating a set of named constants.
- bytes and bytes32: Eventually all the other types are stored in the form of bytes. Bytes themselves are used to store data that is not represented by those other types.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.18;
contract Variables {
// value types
uint256 positiveNumber;
int256 positiveOrNegativeNumber;
bool trueOrFalse;
address userOrContractAddress;
bytes32 someData;
}
Reference types store a reference to the data, which means that they don’t hold a value themselves but rather hold a pointer to a certain location in memory or storage that can have many values. They are used because values such as text words and item lists don’t have a definite size and so cannot be accessed directly like numbers or Booleans. The primary reference types in Solidity include:
- strings: A collection of one or more characters. Any text from “Hello!” to “123” to “?” is considered a string.
- arrays: A collection of data of the same type, like uint[] or bool[];
- mappings: Key-value stores where data is organized in a mapping from keys to values.
- structs: User-defined data structures that can contain a combination of different data types.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.18;
contract Variables {
// value types
uint256 positiveNumber;
int256 positiveOrNegativeNumber;
bool trueOrFalse;
address userOrContractAddress;
bytes32 someData;
enum Colors {
Red,
Green,
Blue
}
// reference types
string text;
bytes data;
uint256[] positiveNumbersList;
address[] userOrContractAddressesList;
mapping(address => uint256) addressToPositiveNumber;
mapping(address => uint256[]) addressToPositiveNumbersList;
mapping(address => mapping(address => bool)) addressToAddressToTrueOrFalse;
struct User {
uint256 positiveNumber;
address userOrContractAddress;
}
}
Solidity Variables: Visibility
Variables can be declared with different visibility modifiers, such as public, private, internal, which determine who can access the variable. Let’s take a look at Solidity public variables, private variables and internal variables.
- Public variables, as their name suggests, are accessible by everyone including the contract itself.
- Private variables on the other hand, are ones that can only be read by the contract itself.
- Internal variables are a special case that can be used by contracts that inherit our contract. We will go in depth about contract inheritance in a separate article so stay tuned.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.18;
contract Variables {
uint256 public notSecretNumber;
uint256 private secretNumber;
uint256 internal internalNumber;
// when you don't specify a visibility, it defaults to public
uint256 number; // same as "public uint256 number";
}
contract VariablesClone is Variables {
// we can access notSecretNumber
// we can access internalNumber
// we can not access secretNumber
function accessNumbers() public {
notSecretNumber = 1;
internalNumber = 2;
// secretNumber = 3; // this will fail
}
}
It must be clarified that once a Solidity contract is live on the blockchain, anyone and everyone can have access to the data inside of it. Changing a variable’s visibility affects how other contracts can interact with that variable from within our contract, but it doesn’t mean that the variable cannot be publicly accessed. Please keep this in mind and refrain from storing any sensitive information in your smart contracts.
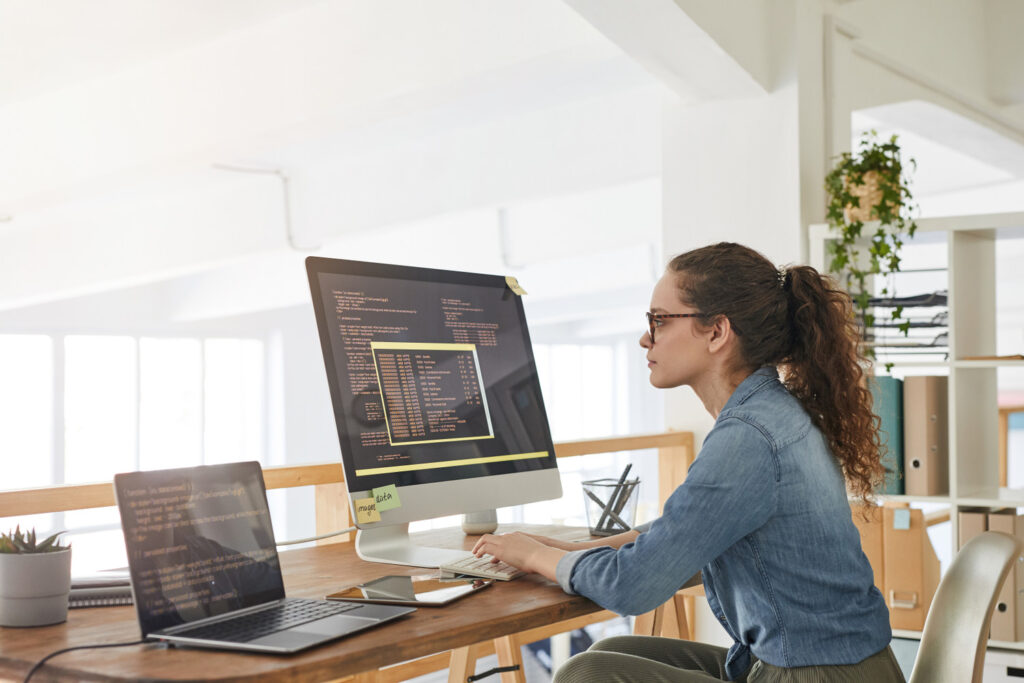
Constants
The last thing you can apply to storage variables is marking them as constant or immutable. Constant variables are not really variables because they never change, their value is declared directly and is encoded into the compiled code of the contract. Immutable values are similar, however they must be set when the contract is created using a special function called the “constructor.”
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.18;
contract Variables {
bool public constant THIS_IS_A_CONTRACT = true;
uint256 public immutable numberOfSnackEatenBeforeDeployment;
constructor(uint256 _numberOfSnackEatenBeforeDeployment) {
numberOfSnackEatenBeforeDeployment = _numberOfSnackEatenBeforeDeployment;
}
}
Solidity Global Variables
One more thing we didn’t talk about here is solidity global variables. Those variables are very special for solidity and the core functionality of the blockchain, so they deserve a spotlight of their own.
Using Your Variables
Variables don’t mean much if you can’t use them, so stay tuned for our next article were we will introduce functions and how to use your variables inside of them!
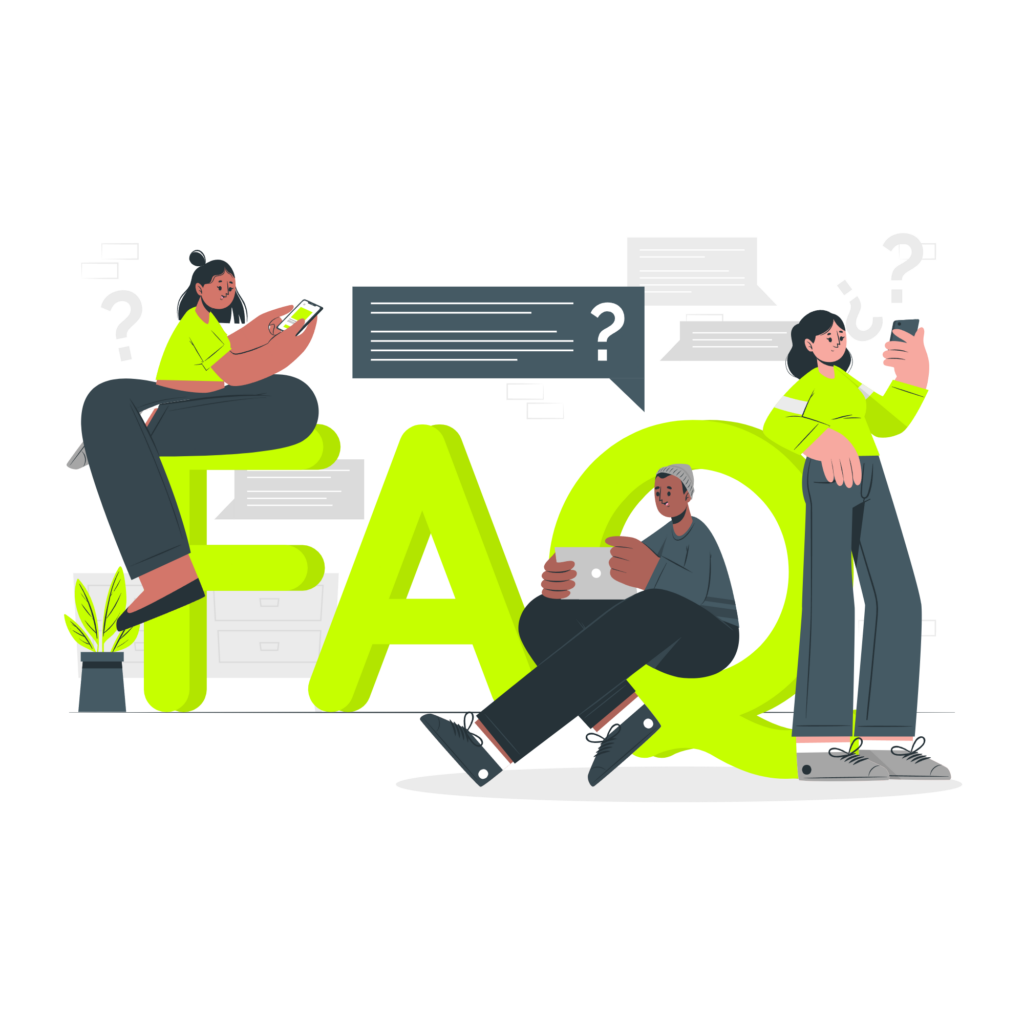
- What are variables in Solidity?
Variables in Solidity are used to store and manipulate data within smart contracts. They can hold different types of values, such as integers, strings, addresses, and more.
- What are the variables of Solidity language?
Solidity language supports various variable types, including uint, int, string, address, bool, and more. It also allows for the creation of custom data structures using structs and arrays.
- What are the types of state variables in Solidity?
In Solidity, state variables can be declared as storage variables, which reside permanently on the blockchain, or as memory variables, which are temporary and used for computations.
- How do you display variables in Solidity?
In Solidity, you can display variables using the emit statement inside events or by using the return statement inside functions to return variables as function outputs.
- How do you display a variable?
You can display a variable in Solidity by using it within a function or event and emitting or returning its value based on the desired use case.
- How do you display variable types?
Variable types in Solidity are determined during declaration by specifying the appropriate type keyword, such as uint, string, address, etc.
- How do you display a variable in a string?
To display a variable within a string in Solidity, you can use string concatenation with the toString() function or by using placeholder syntax, like string(abi.encodePacked(“Variable: “, variable)).
- How do you display two variable data?
To display or output two variable data in Solidity, you can concatenate them within a string using string concatenation or placeholder syntax.
- Which command displays a variable?
In Solidity, the emit command is commonly used within events to display or emit a variable’s value.
- What is a variable command?
There is no specific “variable command” in Solidity. Variables are declared using appropriate type keywords and can be accessed, modified, and displayed within functions and events based on the desired functionality.