Exception handling is a crucial aspect of any programming language, and Web3 development with Solidity is no exception. The try-catch
block offers a mechanism to gracefully handle errors and unexpected situations within your smart contracts. However, misuse of try-catch
can introduce security vulnerabilities and hinder the functionality of your code. Let’s dive into the potential pitfalls of Try-Catch Misuse in Web3 development.
The Misunderstood Safety Net
Imagine a smart contract function that interacts with an external oracle to retrieve data. It wraps the interaction within a try-catch
block, assuming any errors from the oracle will be caught and handled gracefully. But what if the catch
block is empty?
- Swept-Under-the-Rug Errors: Silent failures occur when the
catch
block doesn’t log the error or take corrective actions, leading to unnoticed errors. This can result in unexpected behavior downstream or mask critical issues within the contract. - Logic Shortcomings:
Try-catch
should not replace proper error handling logic. If the function relies on valid data from the oracle, a simplecatch
block without alternative actions might leave the contract in an inconsistent state.
Real-World Repercussions
Here’s how try-catch misuse can manifest in real-world scenarios:
- Incomplete Function Execution: A function wrapped in
try-catch
might encounter an error during an essential step (e.g., funds transfer). If thecatch
block doesn’t revert the transaction, the function might continue execution with partial completion, leading to inconsistencies in the overall state. - Denial-of-Service (DoS) Attacks: Attackers could exploit poorly designed
try-catch
blocks to trigger errors repeatedly. If thecatch
block doesn’t handle these errors efficiently, it can consume gas and potentially block legitimate transactions from being processed.
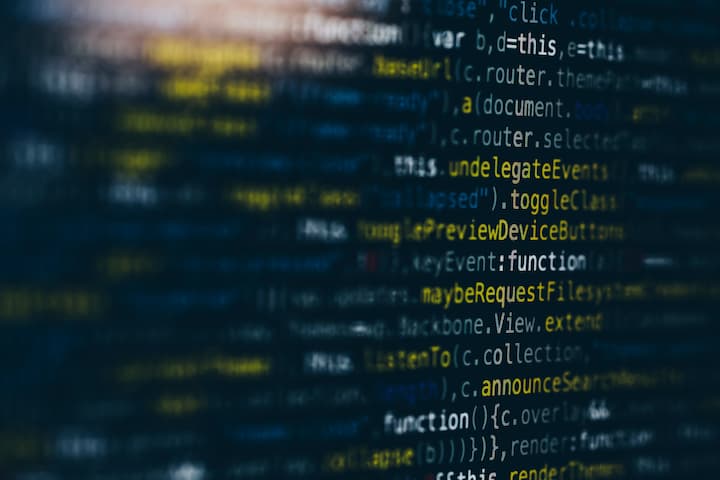
Example: Misuse of Try-Catch
Consider the following example where a smart contract interacts with an external oracle:
pragma solidity ^0.8.0;
contract OracleInteraction {
Oracle oracle;
constructor(address _oracleAddress) {
oracle = Oracle(_oracleAddress);
}
function getOracleData() public returns (bytes memory) {
try oracle.getData() returns (bytes memory data) {
return data;
} catch {
// Empty catch block
}
}
}
interface Oracle {
function getData() external returns (bytes memory);
}
In this example, if the oracle.getData()
call fails, the catch
block does nothing. This silent failure can lead to significant issues:
- Unnoticed Errors: The failure of
oracle.getData()
goes unnoticed, and the functiongetOracleData
returns nothing, which might lead to unexpected behavior in the calling function. - State Inconsistency: If the function depends on the data from the oracle to maintain state consistency, this misuse can result in an inconsistent state.
Wielding Try-Catch Effectively
Web3 developers can leverage try-catch
productively by following these guidelines:
Targeted Exception Handling
Use try-catch
for specific, expected errors. Don’t use it as a catch-all for any unforeseen issues. For instance, if you expect certain types of failures, handle them specifically:
pragma solidity ^0.8.0;
contract OracleInteraction {
Oracle oracle;
constructor(address _oracleAddress) {
oracle = Oracle(_oracleAddress);
}
function getOracleData() public returns (bytes memory) {
try oracle.getData() returns (bytes memory data) {
return data;
} catch Error(string memory reason) {
// Handle the error by reverting with the reason
revert(reason);
} catch (bytes memory lowLevelData) {
// Handle the low-level error
revert("Low-level error occurred");
}
}
}
interface Oracle {
function getData() external returns (bytes memory);
}
In this example, different types of errors are handled separately, providing more information about what went wrong.
Informative Catch Blocks
Log errors within the catch
block to aid debugging and identify potential issues in the future. For example:
solidityCopy code
pragma solidity ^0.8.0;
contract OracleInteraction {
Oracle oracle;
event ErrorLog(string reason);
constructor(address _oracleAddress) {
oracle = Oracle(_oracleAddress);
}
function getOracleData() public returns (bytes memory) {
try oracle.getData() returns (bytes memory data) {
return data;
} catch Error(string memory reason) {
// Log the error
emit ErrorLog(reason);
revert(reason);
} catch (bytes memory lowLevelData) {
// Log the low-level error
emit ErrorLog("Low-level error occurred");
revert("Low-level error occurred");
}
}
}
interface Oracle {
function getData() external returns (bytes memory);
}
By emitting events with error information, you can track issues more effectively.
Revert Transactions on Critical Errors
For functions that modify contract state or involve financial transactions, consider reverting the entire transaction within the catch
block if a critical error occurs. This ensures the contract remains in a consistent state:
solidityCopy code
pragma solidity ^0.8.0;
contract FundsTransfer {
function transferFunds(address payable recipient, uint256 amount) public {
try recipient.send(amount) {
// Funds sent successfully
} catch {
// Revert the transaction on error
revert("Funds transfer failed");
}
}
}
In this example, if the send
function fails, the transaction is reverted, preventing an inconsistent state.
Alternative Actions
In some cases, the catch
block can provide alternative actions or fallback mechanisms if specific errors arise:
solidityCopy code
pragma solidity ^0.8.0;
contract FallbackMechanism {
Oracle oracle;
address fallbackOracle;
constructor(address _oracleAddress, address _fallbackOracleAddress) {
oracle = Oracle(_oracleAddress);
fallbackOracle = _fallbackOracleAddress;
}
function getOracleData() public returns (bytes memory) {
try oracle.getData() returns (bytes memory data) {
return data;
} catch {
// Fallback to another oracle on error
Oracle fallback = Oracle(fallbackOracle);
return fallback.getData();
}
}
}
interface Oracle {
function getData() external returns (bytes memory);
}
Here, if the primary oracle fails, the contract falls back to an alternative oracle to retrieve the data.
Conclusion
The try-catch
block is a powerful tool in Solidity for handling exceptions, but it must be used judiciously. Misuse can lead to silent failures, state inconsistencies, and vulnerabilities like DoS attacks. By following best practices such as targeted exception handling, logging errors, reverting transactions on critical errors, and implementing alternative actions, developers can ensure their smart contracts remain robust and secure.
FAQs
How does try-catch misuse affect Solidity smart contracts?
- Misusing try-catch can leave vulnerabilities in your Solidity smart contracts, potentially exposing them to attacks.
What are the best practices for securing Solidity smart contracts?
- Follow coding standards, conduct thorough testing, and use established security libraries to secure Solidity smart contracts.
Can try-catch statements prevent all errors in Solidity?
- No, try-catch statements can’t catch all types of errors, especially those related to logic flaws and unexpected behaviors.
Why is security important in Solidity smart contracts?
- Security is crucial to prevent loss of funds, unauthorized access, and ensure the reliability and trustworthiness of blockchain applications.
What tools can be used to analyze Solidity smart contract security?
- Tools like MythX, Slither, and Oyente can help analyze and improve the security of Solidity smart contracts.
What is Solidity?
- Solidity is a programming language used for developing smart contracts on the Ethereum blockchain.
How can I learn Solidity programming?
- You can learn Solidity through online bootcamps, courses, tutorials, and by studying documentation provided by the Ethereum community.
What are smart contracts?
- Smart contracts are self-executing contracts with the terms of the agreement directly written into code, running on blockchain networks.
How does blockchain enhance security?
- Blockchain enhances security through its decentralized, immutable, and transparent nature, making data tampering extremely difficult.
What is the role of auditing in smart contract security?
- Auditing involves reviewing code to identify and fix security vulnerabilities before deployment, ensuring the robustness of smart contracts.