A callback is a function passed as an argument to another function, allowing it to be executed later, often after the operation is complete. It is a key concept in JavaScript and are heavily used for handling asynchronous tasks, such as data fetching, event handling, and file I/O operations. They ensure that specific code runs only after a task has finished, enabling smoother and more efficient workflows in JavaScript programs.
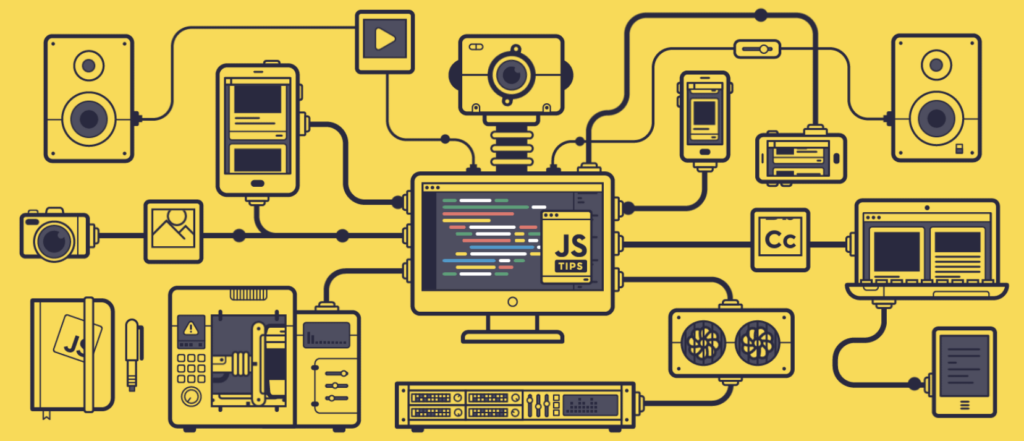
For example, the setTimeout
function in JavaScript executes a callback after a specified delay:
setTimeout(() => {
console.log('Executed after 1 second');
}, 1000); // 1 second = 1000 milliseconds
Understanding the Basics of Callbacks
They are simply functions that are executed after another function finishes its operation. Here is an example of how it can work:
function greet(name, callback) {
console.log(`Hello, ${name}`);
callback();
}
function sayGoodbye() {
console.log('Goodbye!');
}
greet('sam', sayGoodbye);
Output:
Hello, sam
Goodbye!
In this example, sayGoodbye
is passed as a callback to greet
. Once greet
completes, it calls the sayGoodbye
function.
Synchronous vs Asynchronous Callbacks
- Synchronous: Execute immediately after they are called in the function. They are typically used when tasks need to run in a specific order.
[1, 2, 3].forEach(num => console.log(num));
- Asynchronous: Execute later, often after completing a time-consuming task such as an API request or file reading.
setTimeout(() => console.log('Executed after 1 second'), 1000);
Key Differences:
- Synchronous block the execution of subsequent code.
- Asynchronous allow the program to perform other tasks while waiting for the operation to complete.
Real-World Examples:
setTimeout
:
setTimeout(() => {
console.log('Executed after 2 seconds');
}, 2000);
Event Handling:
const button = document.querySelector('button');
button.addEventListener('click', () => {
console.log('Button clicked!');
});
Promises and then
:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log('Data:', data));
How to Implement a Callback Function
To implement this function, you:
- Define a function that accepts another function as an argument.
- Execute the callback function within your main function.
Example:
function processInput(input, callback) {
const output = input.toUpperCase();
callback(output);
}
processInput('hello', console.log);
Output:
HELLO
Common Issues with Callbacks
- Callback Hell (Pyramid of Doom):
Occurs when multiple callbacks are nested within each other, leading to code that is difficult to read and maintain.
Example:
task1(() => {
task2(() => {
task3(() => {
// Further nested tasks
});
});
});
Solution:
Utilize Promises or async/await
to flatten the structure and enhance readability.
Example with Promises:
task1()
task1()
.then(() => task2())
.then(() => task3())
.catch(error => console.error(error));
Example with async/await
:
async function executeTasks() {
try {
await task1();
await task2();
await task3();
} catch (error) {
console.error(error);
}
}
executeTasks();
- Difficulty in Error Handling: errors can become challenging to manage, especially when dealing with multiple nested callbacks.
Solution: adopt the error-first callback pattern, where the first argument is an error object.
Example:
function performTask(callback) {
// Perform operations
if (errorOccurred) {
callback(new Error('An error occurred'));
} else {
callback(null, result);
}
}
performTask((err, result) => {
if (err) {
console.error(err);
return;
}
console.log(result);
});
Inversion of Control: passing a callback means relinquishing control over when and how the function is executed, which can lead to unexpected behaviors if it is invoked multiple times or not at all.
Solution: ensure that the callback is called appropriately within the function and document its expected behavior clearly.
Callback Hell: What It Is and How to Handle It
Callback hell occurs when callbacks are nested within each other, leading to unreadable and difficult-to-maintain code:
task1(() => {
task2(() => {
task3(() => {
console.log('Done!');
});
});
});
Solutions:
Use Promises:
task1()
.then(() => task2())
.then(() => task3())
.then(() => console.log('Done!'));
Use async/await
:
async function runTasks() {
await task1();
await task2();
await task3();
console.log('Done!');
}
Error-First Callbacks: A Standard Pattern
In Node.js, error-first callbacks are a common pattern. The first argument of the callback is reserved for error handling:
const fs = require('fs');
fs.readFile('file.txt', (err, data) => {
if (err) return console.error('Error reading file:', err);
console.log('File content:', data.toString());
});
Advantages and Disadvantages of Callbacks
Advantages:
- Asynchronous Processing: enable non-blocking operations, allowing other code to execute while waiting for long-running tasks to complete.
Example:
console.log('Start');
setTimeout(() => {
console.log('Executed after 2 seconds');
}, 2000);
console.log('End');
Output:
Start
End
Executed after 2 seconds
- Flexibility: allow functions to be designed for reusability by accepting different callback functions to handle various tasks.
Example:
function fetchData(url, callback) {
// Fetch data from the URL
callback(data);
}
fetchData('https://api.example.com/data', processData);
fetchData('https://api.example.com/data', displayData);
Disadvantages:
- Readability Issues: Extensive use of callbacks, especially when nested, can make code harder to read and understand.
Solution: Refactor code using Promises or async/await
to improve clarity.
- Complex Debugging and Maintenance: Tracing the flow of execution and identifying errors can be more challenging in callback-heavy code.
Solution: Implement proper error handling and consider using modern asynchronous patterns to simplify the codebase.
By understanding these common issues and the pros and cons, developers can write more efficient, readable, and maintainable JavaScript code.
FAQs
What is a callback in JavaScript?
- IT is a function passed as an argument to another function, which is then executed after the completion of the first function.
Why are callbacks important in JavaScript?
- Callbacks enable asynchronous programming, allowing tasks like API calls or timers to run without blocking the main execution thread.
How do you write a callback function?
- A callback is simply a function passed as an argument, e.g.,
function doSomething(callback) { callback(); }
.
What is the difference between synchronous and asynchronous callbacks?
- For synchronous, they are executed immediately, while asynchronous are triggered later, after an operation completes.
Are callbacks the same as promises or async/await?
- No, but they serve similar purposes. Promises and async/await provide cleaner, more readable alternatives to callbacks for handling asynchronous code.