In the world of programming, understanding how a program flows is critical to writing efficient and functional code. Two common concepts that help control the execution flow of a program are control statements and control structures. These are often confused with each other, but they serve distinct purposes. In this article, we will explore the differences between control statements and control structures in programming, how they operate, and how they contribute to the overall functionality of a program.
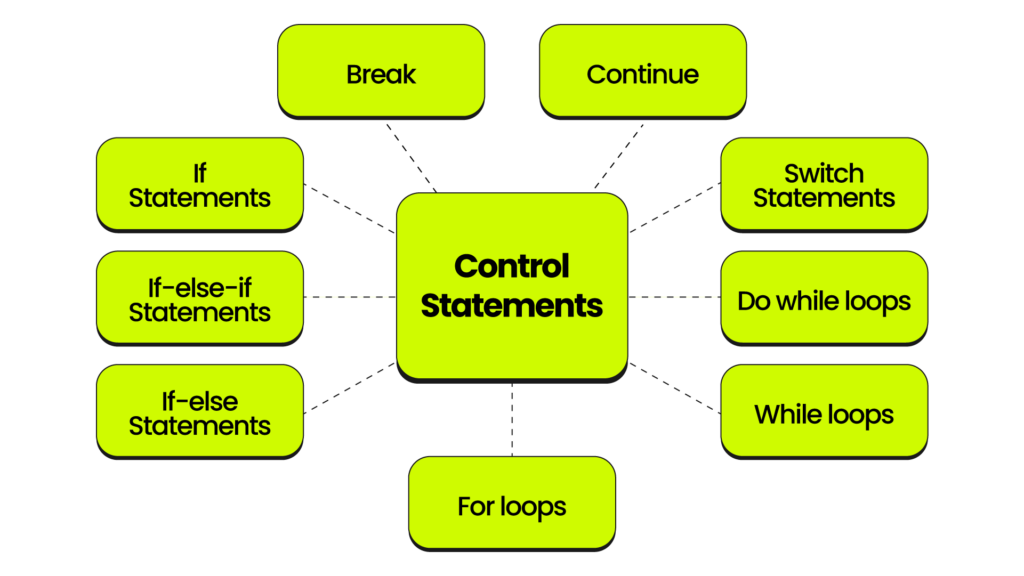
Understanding Control Statements
A control statement is a single command that directs the flow of execution within a program. These commands help the program make decisions, repeat actions, or jump to a different part of the program based on conditions. Control statements allow the program to make decisions during runtime, which makes them fundamental in controlling the logic of any application.
There are various types of control statements, each serving a specific purpose, such as conditional execution, looping, or jumping to a different part of the code. Some of the most commonly used control statements include:
- If-else statements: These control the program’s flow by allowing certain actions to occur only if a particular condition is met. For example, if a certain variable has a specific value, then the program performs a specific action; otherwise, it takes another path.
- Switch statements: Used to simplify multiple if-else conditions. Based on the value of an expression, the program selects one of many possible actions to execute.
- Loops (for, while, do-while): These statements allow the program to repeat a set of actions multiple times. They are typically used when dealing with arrays, lists, or repeated actions in games and applications.
- Break and continue: These are used to control the flow of loops. The break statement is used to exit a loop prematurely, while continue is used to skip the current iteration and move to the next one.
- Return: It is used to exit a function and return a value to the calling code.
The main purpose of a control statement is to direct the flow of control, making sure that the program executes different sets of actions based on specific conditions or loops through code efficiently.
Understanding Control Structures
A control structure is a block or collection of control statements grouped together in a specific sequence to manage the flow of a program. Control structures are a higher-level construct than control statements and can be seen as the logical organization of control statements within a program. They define how control statements are used to structure the program’s flow of execution.
Control structures dictate the overall organization and flow of control within a program. They can be divided into three broad categories:
- Sequential control structures: This is the simplest form of control, where instructions are executed one after another, in the order they appear in the program. There are no decisions or loops involved in this structure. It follows a straightforward top-to-bottom flow of execution.
- Selection control structures: These are used when a decision needs to be made based on a condition. Examples include if-else statements and switch cases. The program follows one path or another based on whether a condition is true or false.
- Repetition control structures: Also known as loops, these structures repeat a block of code multiple times based on a condition. Examples include for loops, while loops, and do-while loops. These control structures are useful for tasks that need to be repeated, such as iterating through a list or calculating a sum over a range of values.
Control structures are essential to create more complex and dynamic programs. They enable developers to design algorithms that can handle various inputs and scenarios efficiently.
Key Differences Between Control Statements and Control Structures
Although control statements and control structures might sound similar, they differ in their roles within a program’s execution flow.
- Definition: Control statements are individual commands that control the flow of execution based on conditions or loops, while control structures are groups of control statements organized in a logical structure that governs the flow of the entire program.
- Complexity: Control statements are simpler and operate at the instruction level. They define what happens at each point in the program. Control structures, on the other hand, involve more complexity as they represent a framework or pattern that guides how the control statements interact with each other.
- Purpose: The main purpose of control statements is to make decisions, loop through code, or jump to specific points in the program. Control structures provide a larger framework that organizes these control statements to dictate the overall program flow.
- Examples: Examples of control statements include if-else, for, while, switch, break, and continue. Control structures include sequential execution, conditional branching (if-else), and loops (for, while, do-while).
- Usage: Control statements are typically used within the body of control structures. For example, an if-else control structure may contain control statements such as conditional checks and return statements. Control structures give context and meaning to the control statements by organizing them in a logical flow.
How Control Statements and Control Structures Work Together
In a well-designed program, control statements and control structures work in tandem to control the flow of execution. Consider the following example in a C-like pseudo code:
for (int i = 0; i < 10; i++) {
if (i % 2 == 0) {
print("Even number: ", i);
} else {
print("Odd number: ", i);
}
}
In this example:
- The for loop is a repetition control structure that repeats a block of code for each value of
i
from 0 to 9. - The if-else statement is a control statement that decides which block of code to execute based on whether
i
is even or odd.
The control structure (for loop) defines the overall structure of the execution, while the control statement (if-else) dictates the specific action based on conditions within that structure.
Importance of Control Statements and Control Structures in Programming
Both control statements and control structures are crucial to effective programming. They provide the flexibility needed to write dynamic and efficient programs that can handle various inputs and execute tasks based on specific conditions.
- Efficiency: Control structures help in reducing code redundancy by allowing loops and conditional execution. Control statements ensure that the program doesn’t waste time executing unnecessary code.
- Readability: Properly organized control structures make the program easier to read and understand. They create a clear logical flow for the code, making it easier for other developers to follow.
- Error Handling: Control statements can be used to manage errors or unexpected behavior during runtime. For example, a control structure can include try-catch blocks that handle exceptions gracefully, improving the robustness of the program.
- Automation: In many applications, automation is a key feature. Control structures like loops are crucial in automating repetitive tasks, such as processing a list of data or performing calculations over a large range of values.
Best Practices for Using Control Statements and Control Structures
Using control statements and control structures effectively is essential for writing efficient and maintainable code. Below are some best practices to keep in mind:
- Minimize nesting: Deeply nested control structures can make code difficult to read and understand. It’s better to break complex logic into functions or use simpler logic to avoid excessive nesting.
- Use meaningful conditions: Make sure the conditions used in control statements are clear and meaningful. Avoid writing overly complex conditions within if-else or loop statements, as this can make the code harder to debug.
- Optimize loops: When using loops, be mindful of their efficiency. Avoid unnecessary iterations by optimizing the loop condition. For example, instead of iterating through every item in a list, consider breaking out of the loop when a condition is met.
- Avoid code duplication: Control structures like loops and conditionals should be used to reduce code duplication. Instead of writing the same block of code multiple times, use loops or functions to handle repetitive tasks.
- Test thoroughly: Control statements, especially those that involve conditions or loops, should be tested thoroughly to ensure that they behave as expected under different conditions.
FAQs
What is the main difference between control statements and control structures?
- The main difference is that control statements are individual commands that control the execution flow based on conditions or loops. In contrast, control structures are patterns or frameworks that organize these control statements to guide the overall program flow.
Can control statements exist without control structures?
- Control statements typically exist within control structures. For example, an if-else control statement is often part of a selection control structure. However, in some cases, simple control statements like break or return may appear outside complex structures.
Why are control structures important in programming?
- Control structures are essential because they define the overall flow of a program. They organize control statements in a way that allows programs to handle various scenarios and make decisions dynamically, improving both efficiency and readability.
Which control structure is the most common?
- The sequential control structure is the most common, as it forms the foundation of most programs. However, conditional structures like if-else statements and loops are also prevalent, especially in more complex programs.
How do control structures improve code efficiency?
- Control structures improve code efficiency by enabling re-usability (e.g., through loops), reducing redundant code, and allowing the program to make decisions dynamically. This results in shorter, faster, and more efficient code.
What are the risks of poorly structured control statements and control structures?
- Poorly structured control statements and control structures can lead to errors, inefficient code, and a lack of clarity. Over-nesting, poor conditions, and un-optimized loops can make the code difficult to maintain and slow down execution.
What is an example of a control statement in programming?
- An example of a control statement is an if-else statement, where the program checks a condition and executes one block of code if the condition is true and another block if the condition is false.