The Ethereum Virtual Machine (EVM) is a critical component of the Ethereum blockchain, powering smart contracts and decentralized applications (dApps). Understanding how to interact with the EVM can unlock immense potential for developers and blockchain enthusiasts alike. This guide delves into the intricacies of the EVM, offering a thorough explanation of its operations, the ways to interact with it, and how to leverage its features for building blockchain applications.
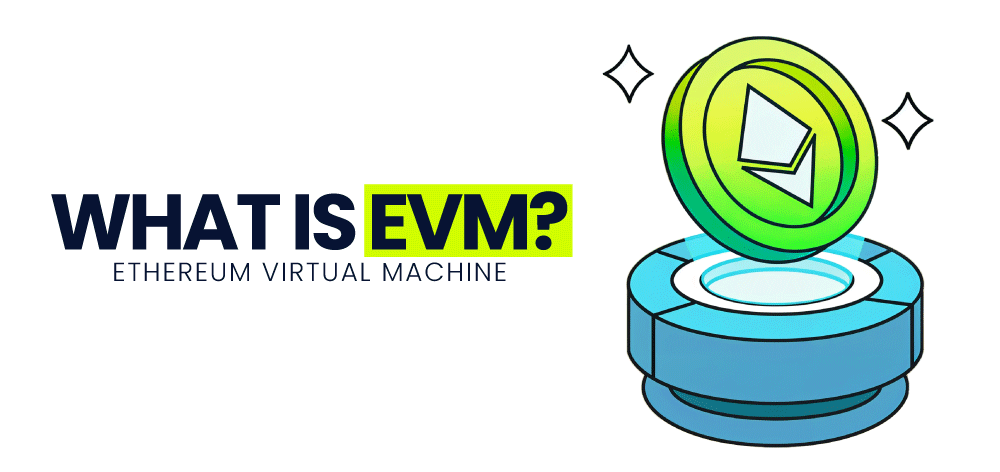
What is the Ethereum Virtual Machine (EVM)?
The Ethereum Virtual Machine (EVM) is a decentralized computing platform that enables the execution of smart contracts on the Ethereum network. It operates as a virtual environment, meaning it can run code exactly as intended, and the state of the blockchain is updated accordingly. The EVM ensures consistency and correctness across the network, meaning the same code will produce the same output on every Ethereum node, irrespective of where it is run.
The EVM is Turing complete, meaning it can perform any computation that a computer can, provided there is enough computational power and resources. It is this capability that has transformed Ethereum from a simple cryptocurrency network into a platform for decentralized applications and smart contracts.
Key Concepts of the Ethereum Virtual Machine
To effectively interact with the EVM, it is essential to understand some of its core components and how it functions. Here are key concepts:
Smart Contracts
- Smart contracts are self-executing contracts with the terms directly written into code. These are deployed and executed on the EVM, which ensures that once deployed, the contract operates as intended without external interference.
Bytecode
- Smart contracts written in high-level languages like Solidity are compiled into bytecode, which the EVM can interpret and execute. Bytecode is a low-level language understood by the EVM, consisting of opcodes that represent different computational tasks.
Gas
- Gas is the unit of measurement for computational work on the Ethereum network. Every action on the EVM, including executing a smart contract or sending Ether, requires a certain amount of gas. Users must pay gas fees to miners for validating and processing transactions.
Opcodes
- Opcodes (operation codes) are the EVM’s low-level instructions for performing specific tasks, like arithmetic operations, data storage, or branching logic. When interacting with the EVM, these opcodes are the building blocks for executing contracts.
State
- The EVM maintains the state of the Ethereum blockchain. Each node in the network holds a copy of the entire state, and every interaction with the EVM results in state changes, which are reflected on the blockchain.
How to Interact with the Ethereum Virtual Machine
Interacting with the EVM can be done in various ways depending on your goal—whether you want to deploy smart contracts, read from the blockchain, or send transactions. Here’s how you can effectively interact with the EVM:
Writing Smart Contracts
Smart contracts are the backbone of Ethereum’s decentralized ecosystem. The most common language for writing smart contracts is Solidity, though there are other languages like Vyper. Solidity is similar to JavaScript in syntax and is widely used due to its robust documentation and community support.
Once the smart contract is written, it is compiled into EVM bytecode, which can then be deployed on the Ethereum network.
Below is an example of a Simple Solidity Contract
pragma solidity ^0.8.0;
contract SimpleStorage {
uint storedData;
function set(uint x) public {
storedData = x;
}
function get() public view returns (uint) {
return storedData;
}
}
This contract stores a value and allows anyone to set or get that value.
Deploying Smart Contracts
Once you’ve written a smart contract, it needs to be deployed to the Ethereum network. Deployment involves broadcasting the compiled bytecode in a transaction. Popular tools like Remix, Hardhat, and Truffle allow developers to compile and deploy smart contracts seamlessly.
To deploy the contract, the developer must send a transaction containing the compiled contract bytecode to the Ethereum network. During the deployment, you will need to specify gas fees, and once the transaction is mined, the contract will have its own Ethereum address.
Interacting with Smart Contracts
After deployment, you can interact with the smart contract by calling its functions. There are two main types of function calls:
- Read-Only Calls (view/pure): These functions do not modify the blockchain state and do not require gas. For instance, retrieving the value stored in the contract’s variable.
- State-Changing Transactions: These functions modify the blockchain state and require gas. An example is calling a function that updates a stored value in the contract.
To interact with the contract, you can use libraries such as Web3.js, Ethers.js, or a wallet like MetaMask. These tools facilitate communication with the Ethereum nodes (full or light nodes) to send transactions, query data, and handle contract calls.
Gas Management
As mentioned earlier, gas is crucial for executing any operation on the EVM. Every transaction or smart contract execution costs gas, and users must specify the gas limit and gas price. The gas price determines how much Ether (ETH) the user is willing to pay per unit of gas.
Gas management is critical for ensuring that your transaction is processed by miners. If you set a gas price too low, your transaction may be delayed or not processed at all.
Handling Transactions
Sending transactions is one of the most common ways to interact with the EVM. A transaction is a signed data package that stores a message to be sent from one account to another, along with an optional data payload (such as the invocation of a smart contract function).
To send a transaction, you need to:
- Specify the recipient address (or contract address in case of smart contract interaction).
- Provide the amount of Ether to send (if applicable).
- Attach the data payload (in the case of smart contract function calls).
- Specify the gas price and gas limit.
Popular tools like MetaMask, Web3.js, and Ethers.js allow users to create and sign transactions easily.
Tools for Interacting with the EVM
Interacting with the EVM requires specialized tools that simplify development, testing, and deployment processes. Here are some essential tools for working with the Ethereum Virtual Machine:
Remix IDE
A web-based integrated development environment (IDE) for writing, testing, and deploying smart contracts. It supports Solidity and provides a fast way to experiment with smart contracts without needing a local Ethereum node.
Truffle Suite
A popular development framework for Ethereum, Truffle provides tools for compiling, deploying, and testing smart contracts. It integrates with Ganache, a personal Ethereum blockchain for running tests, and is widely used by developers for professional dApp development.
Hardhat
Hardhat is an advanced Ethereum development environment. It allows you to deploy and test smart contracts locally, offers built-in support for debugging, and can integrate with popular Ethereum tools.
MetaMask
MetaMask is a browser extension wallet that enables users to interact with the Ethereum blockchain. Developers can use MetaMask to deploy contracts, send transactions, or connect with dApps.
Web3.js
A JavaScript library that allows you to interact with an Ethereum node using HTTP, WebSocket, or IPC. Web3.js is essential for querying the blockchain, sending transactions, or interacting with smart contracts via a web application.
Ethers.js
Similar to Web3.js, Ethers.js is a JavaScript library that provides a simpler and smaller alternative for interacting with the Ethereum blockchain. It is known for its lightweight design and developer-friendly API.
Security Best Practices for Interacting with the EVM
Security is paramount when interacting with the Ethereum network and EVM, as smart contracts, once deployed, cannot be modified or reversed. To ensure your interactions with the EVM are secure, follow these best practices:
- Audit Smart Contracts: Before deploying a contract to the Ethereum mainnet, it is essential to conduct thorough audits. This can help catch vulnerabilities or potential exploits.
- Use Reputable Libraries: When developing smart contracts, use well-established libraries like OpenZeppelin to ensure your code adheres to security best practices.
- Test Extensively: Testing your smart contracts locally or on test networks (like Ropsten or Goerli) ensures that any issues are addressed before deploying on the mainnet.
- Manage Gas Efficiently: Keep track of gas usage in your contracts to avoid unnecessary costs or transaction failures due to running out of gas.
Challenges of Interacting with the EVM
Interacting with the EVM presents several challenges, particularly for developers new to blockchain. Some of the common challenges include:
- Gas Optimization: Writing efficient smart contracts that minimize gas usage can be complex, especially when dealing with large transactions or intricate logic.
- Security Risks: Smart contracts are immutable once deployed, meaning any bugs or vulnerabilities cannot be fixed unless carefully accounted for during development.
- Learning Curve: Mastering Solidity, EVM opcodes, and Ethereum tooling can take time, as it requires understanding both blockchain principles and programming concepts.
FAQs
What is the Ethereum Virtual Machine (EVM)?
- The EVM is a decentralized, Turing-complete virtual machine that executes smart contracts on the Ethereum blockchain. It ensures consistency and correctness across the network by running the same code on every node.
How do I interact with the Ethereum Virtual Machine?
- You can interact with the EVM by writing smart contracts, deploying them to the Ethereum network, and executing or reading from those contracts using tools like Web3.js, Ethers.js, or MetaMask.
What are smart contracts?
- Smart contracts are self-executing contracts where the terms of the agreement are written in code. Once deployed, they run automatically on the EVM without the need for intermediaries.
What is gas in the Ethereum network?
- Gas is the unit of measurement for computational effort on the Ethereum network. Every operation, whether it’s a transaction or a smart contract execution, requires a certain amount of gas. Users pay gas fees to miners who validate and process the transaction.
Which tools are best for interacting with the EVM?
- Popular tools for interacting with the EVM include Remix IDE, Truffle, Hardhat, Web3.js, Ethers.js, and MetaMask. These tools simplify contract development, deployment, and transaction management.
Why is security important when interacting with the EVM?
- Once a smart contract is deployed, it cannot be modified or corrected. Therefore, ensuring that smart contracts are secure and free from vulnerabilities is critical, as any bugs or exploits could lead to loss of funds or contract failure.