Web3 applications represent the cutting edge of decentralization, but they do not operate in isolation. These applications require real-world data to function effectively, and this is where oracles come into play. Oracles act as bridges, fetching external data and feeding it securely onto the blockchain. However, this dependency introduces a critical vulnerability known as Oracle Dependence.
Why Oracles Matter ?
To understand the importance of oracles, consider a DeFi (Decentralized Finance) lending platform that needs to determine the current market price of an asset before processing a loan request. This platform cannot access this information directly from the blockchain. Instead, it relies on oracles to retrieve the price data, validate it, and deliver it to the smart contract that governs the lending process.
Here’s an example of how this might work in Solidity, the programming language used for writing Ethereum smart contracts:
pragma solidity ^0.8.0;
interface IPriceOracle {
function getLatestPrice() external view returns (int);
}
contract DeFiLending {
IPriceOracle public priceOracle;
constructor(address _oracleAddress) {
priceOracle = IPriceOracle(_oracleAddress);
}
function getAssetPrice() public view returns (int) {
return priceOracle.getLatestPrice();
}
function processLoan(uint256 amount) public {
int price = getAssetPrice();
// Logic to process loan based on the price
}
}
In this example, the DeFiLending
contract relies on an external PriceOracle
contract to fetch the latest price of an asset.
The Double-Edged Sword
While oracles are essential for connecting blockchain applications with the outside world, their dependence creates several vulnerabilities:
Single Point of Failure
If an oracle is compromised or malfunctions, it can deliver inaccurate data, leading to cascading errors within the smart contract. For instance, a price manipulation attack on an oracle could lead to unfair liquidations in a DeFi protocol.
Limited Scope
Oracles can only provide data they are programmed to fetch. If a critical piece of information is missing or incorrect, the smart contract might make faulty decisions. For instance, if an oracle fails to update prices during high volatility, the smart contract may operate on outdated information.
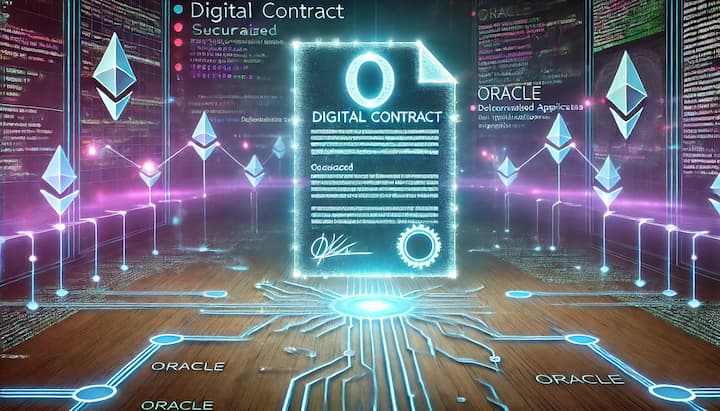
Real-World Wobbles
Oracle dependence has caused significant issues in the real world, highlighting the vulnerabilities:
The MakerDAO Black Thursday (2020)
During a flash crash, inaccurate price feeds from oracles led to a cascade of liquidations in the MakerDAO stablecoin system. The incident caused massive losses for users and highlighted the risks associated with relying on a single source of truth.
The Chainlink Flash Loan Attack (2021)
An exploit targeted a vulnerability in a specific oracle provider, briefly impacting some DeFi protocols that relied on its data. This incident underscored the need for robust and secure oracle systems to prevent similar exploits.
Building Bridges of Trust
To mitigate these risks, developers can take several measures to enhance the reliability and security of oracles:
- Decentralized Oracles Utilize decentralized oracle networks that aggregate data from multiple sources, reducing reliance on a single point of failure. For example, Chainlink is a popular decentralized oracle network that uses multiple nodes to fetch and verify data.
- Reputation Systems Integrate oracle reputation systems that assess the reliability of data providers. These systems can score oracles based on their performance and accuracy, helping smart contracts choose the most reliable sources.
- Multiple Oracle Feeds Consider incorporating data from multiple oracles to enhance overall accuracy and redundancy. By averaging data from several sources, the impact of any single faulty oracle can be minimized. Here’s an example of how to implement multiple oracle feeds in Solidity:
pragma solidity ^0.8.0;
interface IPriceOracle {
function getLatestPrice() external view returns (int);
}
contract MultiOracleLending {
IPriceOracle[] public priceOracles;
constructor(address[] memory _oracleAddresses) {
for (uint256 i = 0; i < _oracleAddresses.length; i++) {
priceOracles.push(IPriceOracle(_oracleAddresses[i]));
}
}
function getAveragePrice() public view returns (int) {
int sum = 0;
for (uint256 i = 0; i < priceOracles.length; i++) {
sum += priceOracles[i].getLatestPrice();
}
return sum / int(priceOracles.length);
}
function processLoan(uint256 amount) public {
int averagePrice = getAveragePrice();
// Logic to process loan based on the average price
}
}
In this example, the MultiOracleLending contract fetches prices from multiple oracles and calculates an average price to ensure more reliable data for loan processing.
Conclusion: Oracle Dependence
Oracle dependence is a critical aspect of Web3 applications that bridges the gap between blockchain and the real world. While oracles provide essential data, their reliance introduces vulnerabilities that developers must address. By using decentralized oracles, reputation systems, and multiple oracle feeds, developers can build more robust and secure smart contracts.
Understanding and mitigating oracle dependence is crucial for the continued growth and success of decentralized applications. As the Web3 ecosystem evolves, so too must the strategies for securing the oracles that underpin it.
FAQs:
What is an oracle in the context of smart contracts?
- An oracle is an external data source that provides smart contracts with real-world information needed to execute agreements.
Why are oracles important for smart contracts?
- They enable smart contracts to interact with real-world data, making them more versatile and applicable to a wider range of use cases.
How do oracles ensure data accuracy and reliability?
- They often use multiple data sources and verification methods to ensure the accuracy and reliability of the information provided.
What are the risks associated with oracle dependence in smart contracts?
- Oracle dependence can introduce vulnerabilities, such as data manipulation or single points of failure, potentially compromising the security of smart contracts.
How can oracle-related risks be mitigated in smart contracts?
- Implementing decentralized oracles and using multiple data sources can help mitigate risks associated with oracle dependence.
Can smart contracts function without oracles?
- Yes, but their functionality would be limited to blockchain-native data, reducing their potential use cases significantly.
What are decentralized oracles?
- These are oracles that aggregate data from multiple sources, reducing the risk of manipulation and single points of failure.
How do oracles impact the scalability of blockchain applications?
- They can affect scalability by introducing additional steps and verification processes, potentially slowing down transaction times.
What are some popular oracle providers in the blockchain space?
- Chainlink, Band Protocol, and API3 are some well-known providers that offer reliable data services for smart contracts.
How do oracles contribute to the evolution of decentralized finance (DeFi)?
- Provide essential real-time data for DeFi applications, enabling functionalities like price feeds, lending rates, and market predictions.