TL;DR
- Understanding JavaScript syntax is important for writing clean and efficient code.
- This article covers all basic rules and syntax you need to follow while developing code in JavaScript.
- To write quality code, follow best practices like consistent indentation, meaningful comments, and keeping functions focused on a single task.
- By avoiding the common mistakes outlined in this article, you can quickly improve your skills and become an expert in JavaScript development.
JavaScript is one of the most widely used programming languages in the world, acting as the backbone of modern web functionality. For anyone starting their journey in web development, it is important to have a solid understanding of JavaScript and its foundational concepts.
In this article, we will explore the basic rules, statements, and best practices that define JavaScript. By the end, you should have a clearer understanding of how this works and how to write clean, efficient, and maintainable code.
Understanding JavaScript Syntax
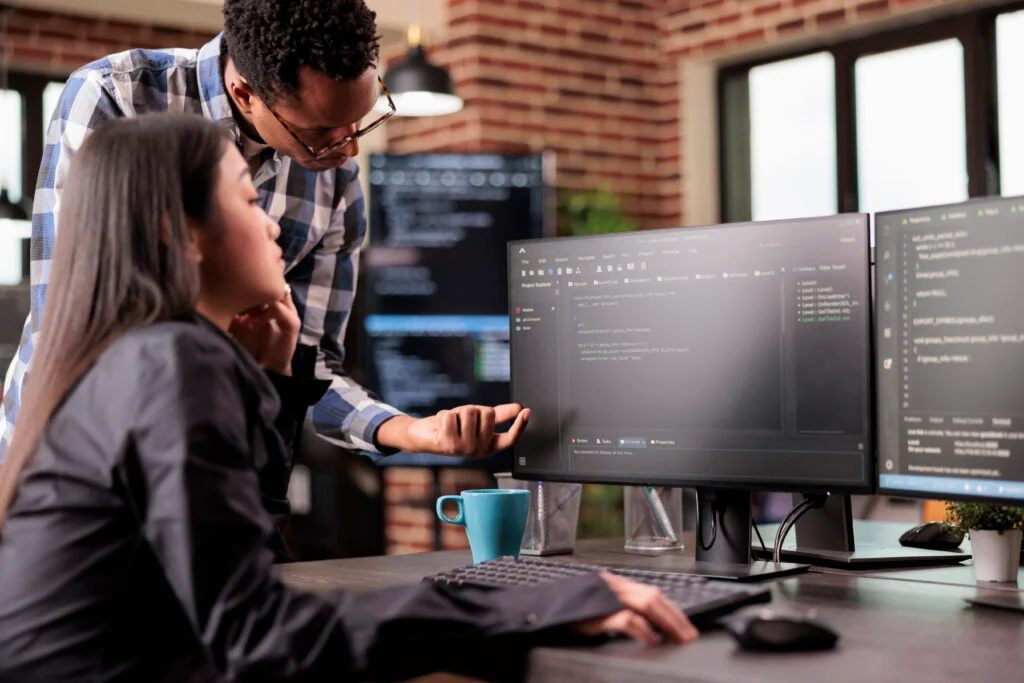
JavaScript is one of the most widely used programming languages for web development. It allows developers to create dynamic, interactive websites by controlling elements on a page. To write effective JavaScript code, it’s essential to understand its syntax rules and structure.
Before we dive into, some fundamental rules to keep in mind when working with JavaScript. let’s go over The term syntax refers to the set of rules that define how a programming language is written and interpreted. In JavaScript, syntax dictates how you structure statements, declarations, and expressions so that your code can be understood and executed properly by the JavaScript engine.
Variables and their Declarations
A variable is a named container that stores data. In JavaScript, you have three main ways to declare variables.
var
- Before ECMAScript 2015 (ES6),
var
was the only way to declare variables in JavaScript. Variables declared withvar
are function-scoped, meaning if you declare avar
inside a function, it cannot be accessed outside that function. However, if declared at the top level,var
becomes a property of the global object, which can lead to conflicts and unintended behavior.
- Before ECMAScript 2015 (ES6),
let
- Introduced in ES6,
let
is block-scoped, which means variables are only accessible within the nearest set of curly braces or within a function. Usinglet
is generally preferred overvar
for its predictable scoping and reduced risk of variable conflicts.
- Introduced in ES6,
const
- Similar to
let
,const
is also block-scoped, but its value cannot be reassigned once declared. This is particularly useful for values that should remain constant throughout the execution of your code, making your intent clearer to anyone reading your code. Note that while the reference can’t be reassigned, properties of a const-declared object can still be modified.
- Similar to
Following are some examples of how you can declare variables in JavaScript using var
, let
, and const
.
var name = "Alice";
name = "Bob";
console.log(name); // Output: Bob
let age = 25;
age = 30;
console.log(age); // Output: 30
const country = "USA";
// country = "Canada"; // Error: Cannot reassign
console.log(country); // USA
Data Types in JavaScript
JavaScript has several built-in data types that define how values can be used and manipulated. Understanding data types is important for learning the JavaScript syntax.
Number | Represents both integers and floating-point values. |
String | A sequence of characters enclosed in single quotes, double quotes, or backticks |
Boolean | Represents either true or false |
Undefined | A variable that has been declared but not assigned a value. |
Null | Explicitly indicates an empty or non-existent value. |
Symbol | A unique and immutable data type introduced in ES6, often used as object keys. |
BigInt | An integer type that can represent arbitrarily large numbers |
Control Flow Statements
Now that we know how to declare variables and understand their data types, let’s explore how JavaScript executes code. Control flow statements determine the execution path of a program, allowing you to run specific blocks of code based on conditions, create loops, and handle errors. Let’s break down these control flow statements and examine them individually.
The if
, else if,
else
Statement
The if
statement executes a block of code if a specified condition evaluates to true
. If the condition is false
, JavaScript checks else if
conditions (if any) or defaults to the else
block.
For example let’s look at the code below
let score = 85;
if (score > 90) {
console.log("Grade: A");
} else if (score > 80) {
console.log("Grade: B");
} else {
console.log("Grade: C or below");
}
If score
is greater than 90, the output will be “Grade: A.” If it’s between 81 and 90, it will be “Grade: B.” Otherwise, “Grade: C or below” is displayed.
The switch
Statement
The switch
statement is useful when comparing a variable against multiple fixed values. It improves readability compared to multiple if
conditions.
For example let’s look at the code below
let color = "green";
switch (color) {
case "red":
console.log("Stop");
break;
case "yellow":
console.log("Caution");
break;
case "green":
console.log("Go");
break;
default:
console.log("Invalid color");
}
This program evaluates the color
variable and executes the matching case statement. If the value doesn’t match any case, it executes the default
statement.
The while
Loop
Executes a block of code as long as the condition remains true
.
For example let’s look at the code below
let count = 0;
while (count < 5) {
console.log(count);
count++;
}
The loop runs while count
is less than 5, increasing it in each iteration. When count reaches 5, the loop with be terminated.
The do...while
Loop
Executes the code at least once, even if the condition is false.
For example let’s look at the code below
let count = 5;
do {
console.log(count);
count++;
} while (count < 5);
Here, even though count
is already 5, the loop executes once before checking the condition.
The for
Loop
A for
loop is often used when you know in advance how many times you want the loop to run.
For example let’s look at the code below
for (let i = 0; i < 5; i++) {
console.log(i);
}
This loop runs 5 times, starting from i = 0
and stopping when i = 4
.
The for...of
Loop
This loop is useful for iterating over iterable objects like arrays or strings.
For example let’s look at the code below
const fruits = ["apple", "banana", "cherry"];
for (const fruit of fruits) {
console.log(fruit);
}
This loop outputs each element of the fruits
array.
The for...in
Loop
The for...in
loop iterates over the enumerable properties of an object.
For example let’s look at the code below
const user = { name: "Bob", age: 30 };
for (const key in user) {
console.log(key, user[key]);
}
This loop outputs each property and its value in the user
object.
Functions in JavaScript
Functions are fundamental building blocks in JavaScript, allowing you to write reusable and organized code. A function is a self-contained block of code designed to perform a specific task. Functions can accept inputs, process them, and return outputs.
JavaScript offers several ways to define functions, now we’ll explore each of them in detail.
Function Declarations
A function declaration defines a named function that can be called before it is defined in the code.
function add(a, b) {
return a + b;
}
let sum = add(2, 3); // sum = 5
Function Expressions
A function expression assigns a function to a variable. Unlike function declarations, function expressions are not hoisted, meaning they cannot be called before they are defined.
const subtract = function (a, b) {
return a - b;
};
let difference = subtract(5, 2); // difference = 3
Arrow Functions
Arrow functions provide a shorter and more concise syntax for writing functions. They also have lexical this
binding, which makes them useful in certain cases, such as event handlers and callbacks.
const multiply = (a, b) => a * b;
console.log(multiply(3, 4));
Objects and Arrays
In JavaScript, nearly everything that is not a primitive data type is an object. Objects are collections of key-value pairs, while arrays are ordered lists of values.
Objects
In JavaScript, objects are used to store key-value pairs, making it easy to organize and manage related data. Each property of an object can be accessed using either dot notation (object.property
) or bracket notation (object["property"]
). You can follow the syntax below to create an object.
const person = {
firstName: "John",
lastName: "Doe",
age: 25,
greet: function() {
console.log("Hello, I am " + this.firstName);
},
};
person.greet(); // "Hello, I am John"
console.log(person.age); // 25
Arrays
Arrays store values in an ordered list. Each value can be accessed by its index, starting at 0. You can follow the syntax below to create an array in JavaScript.
const colors = ["red", "green", "blue"];
console.log(colors[1]); // "green"
colors.push("yellow"); // Adds "yellow" to the end of the array
Best Practices for Clean JavaScript Code
Knowing JavaScript syntax is just the beginning. To write clean, readable, and maintainable code, it’s important to follow best practices and established conventions. Following are some key best practices to help you write cleaner, more efficient JavaScript code.
- Use Strict Mode
- Enabling strict mode at the beginning of your script or function can help you avoid common pitfalls by throwing errors for unsafe actions. Strict mode also eliminates some silent JavaScript errors, making debugging easier.
- Prefer
const
andlet
overvar
- Using
const
for values that do not change andlet
for reassignable variables can help prevent variable re-declaration issues. This makes your code more predictable.
- Using
- Consistent Naming Conventions
- Use descriptive names for variables and functions, sticking to commonly accepted practices such as
camelCase
for variables and functions, andPascalCase
for constructor functions or classes in object-oriented JavaScript.
- Use descriptive names for variables and functions, sticking to commonly accepted practices such as
- Avoid Global Variables
- To prevent naming conflicts and make your code more modular, minimize the use of global variables. Whenever possible, encapsulate your code within functions, classes, or modules.
- Keep Functions Focused
- A function should ideally do one thing and do it well. If you find a function growing too large or handling multiple responsibilities, consider refactoring it into smaller functions.
- Meaningful Comments
- Write comments that add value for future readers. Avoid stating the obvious, but take the time to clarify complex logic or edge cases to make sure that the code is easily understandable.
- Avoid Deep Nesting
- Excessive nesting of loops or conditional statements can make your code difficult to read. Consider early returns or splitting logic into smaller functions instead of deeply nested structures.
Common Mistakes to Avoid
Even with a good understanding of JavaScript, it’s easy to run into common mistakes. Following are a few to watch out for.
- Implicit Type Coercion
- JavaScript can implicitly convert one data type to another during comparisons or arithmetic operations, which can lead to surprising results if you’re not careful. For example, using
==
may produce unexpected outcomes.
- JavaScript can implicitly convert one data type to another during comparisons or arithmetic operations, which can lead to surprising results if you’re not careful. For example, using
- Misuse of
this
- The value of
this
depends on the context in which a function is invoked, not where it is defined. Arrow functions do not have their ownthis
; instead, they capture thethis
from their enclosing scope. Understand these rules to avoid confusing behavior, especially in event handlers and callbacks.
- The value of
- Mutating Objects and Arrays Unintentionally
- If you declare a variable without
var
,let
, orconst
, it automatically becomes a global variable (in non-strict mode). This can cause naming collisions and hard-to-track bugs. In strict mode, this will throw an error, which is one reason to use strict mode.
- If you declare a variable without
- Forgetting the
var
,let
, orconst
Keyword- JavaScript objects and arrays are passed by reference. Changing an object or array inside a function changes it everywhere else in your code that references it. If you need to maintain an original copy, you might use immutable techniques or create a clone.
Conclusion
Learning the syntax is important to becoming a skilled developer, but the language continues to evolve. Staying updated with the latest ECMAScript features and refining your knowledge will help you write better code.
Coding isn’t just about getting things to work, it’s about writing clean, efficient, and maintainable code. By deeply understanding JavaScript and following best practices, you’ll be able to build scalable and long-lasting web applications.
FAQs
Why is JavaScript syntax important?
- Syntax is crucial because it is the language’s “grammar.” If you violate the syntax rules, your code will either fail to run or produce unexpected results. Proper syntax ensures clarity, maintainability, and compatibility across different environments.
Should I always use semicolons in JavaScript?
- While JavaScript does have Automatic Semicolon Insertion (ASI) that can add semicolons for you in many cases, it’s generally safer and more consistent to include them explicitly. This reduces ambiguity and prevents unexpected behavior in edge cases.
How can I improve the readability of my JavaScript code?
- To improve code readability, it’s important to follow certain best practices. Consistently using proper indentation and spacing helps make the code visually organized and easier to follow. Always include semicolons to avoid potential issues, and follow naming conventions, such as using camelCase for variables and functions, to maintain consistency across the codebase.
- Writing meaningful comments and avoiding deeply nested logic makes the code easier to understand for others and yourself in the future. Lastly, keeping functions focused on a single task enhances clarity and makes the code more maintainable.
What is the best way to handle data type comparisons in JavaScript?
- Use the strict equality operator (
===
) and strict inequality operator (!==
) whenever possible. Strict equality ensures that no type coercion occurs, which makes your comparisons more reliable and predictable.
Is JavaScript case-sensitive?
- Yes, JavaScript is case-sensitive. Variable names, function names, and object properties must be written using consistent casing, or the language will treat them as different identifiers.