Web3 thrives on innovation, but unlike traditional software, upgrades to deployed smart contracts can be a tricky affair. The very nature of blockchain immutability presents a challenge: Upgrade Bugs. Let’s explore the potential pitfalls of upgrading smart contracts and how developers can navigate this complex landscape.
The Immutability Impasse
Imagine a decentralized application built on a smart contract with a critical bug. Fixing the bug necessitates an upgrade. However, once deployed on the blockchain, a smart contract cannot be directly altered. Here’s where the trouble begins:
Hard Forks
One approach involves a hard fork – a radical change to the blockchain protocol that creates a new chain with the upgraded code. This can be disruptive, requiring users and applications to adapt to the new chain. Hard forks are often seen as a last resort due to their potential to split the community and the ecosystem.
Proxy Contracts
A more common approach is to deploy a new, upgraded smart contract and then migrate user interactions to it. This introduces a new layer of complexity and potential vulnerabilities:
- Migration Issues: The migration process itself can be buggy, leading to data loss or inconsistencies between the old and new contracts.
- Centralization Risks: Proxy contracts might introduce a layer of centralization, where the upgrade process relies on a single entity controlling the migration logic.
Example: Simple Proxy Pattern
Here’s an example of how a proxy pattern can be implemented to facilitate upgrades:
// Proxy Contract
contract Proxy {
address public implementation;
constructor(address _implementation) {
implementation = _implementation;
}
function upgradeTo(address newImplementation) public {
implementation = newImplementation;
}
fallback() external payable {
address impl = implementation;
require(impl != address(0), "Implementation address not set");
assembly {
let ptr := mload(0x40)
calldatacopy(ptr, 0, calldatasize())
let result := delegatecall(gas(), impl, ptr, calldatasize(), 0, 0)
let size := returndatasize()
returndatacopy(ptr, 0, size)
switch result
case 0 { revert(ptr, size) }
default { return(ptr, size) }
}
}
}
// Initial Implementation
contract LogicV1 {
uint public value;
function setValue(uint _value) public {
value = _value;
}
}
// Upgraded Implementation
contract LogicV2 {
uint public value;
function setValue(uint _value) public {
value = _value * 2; // New logic for demonstration
}
}
In this example, the Proxy
contract delegates all calls to the current implementation
contract. The upgradeTo
function allows for the upgrade of the implementation contract, but care must be taken to ensure this process is secure and well-tested.
Real-World Roadblocks
Upgrade bugs have caused significant issues in Web3 projects, highlighting the need for robust upgrade strategies.
The DAO Split (2016)
A critical vulnerability in The DAO smart contract led to a hard fork, creating two versions of the Ethereum blockchain – Ethereum (ETH) and Ethereum Classic (ETC). This controversial decision highlighted the challenges and risks associated with upgrading smart contracts through hard forks.
The Parity Library Bug (23017)
A bug in a popular library used for creating upgradeable contracts caused several projects to lose access to their funds. The bug was due to an initialization issue in a multi-signature wallet library, showcasing the risks associated with complex migration mechanisms.
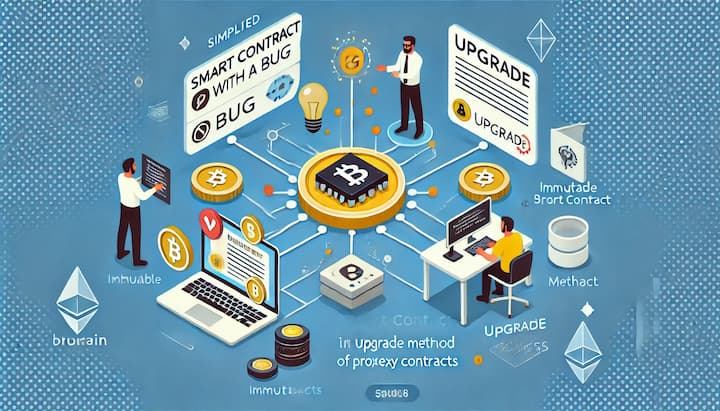
The Art of the Upgradable Contract
To mitigate upgrade bugs, developers can adopt several strategies:
Design for Upgradeability
From the outset, consider the upgrade path for your smart contract. This might involve using established upgrade patterns or frameworks that provide a safer approach to managing upgrades.
Example: OpenZeppelin’s Upgradeable Contracts
OpenZeppelin provides a library for creating upgradeable contracts. Here’s a basic example:
// Using OpenZeppelin's Upgradeable Contracts
// First, install the OpenZeppelin contracts:
// npm install @openzeppelin/contracts-upgradeable
import "@openzeppelin/contracts-upgradeable/proxy/utils/Initializable.sol";
import "@openzeppelin/contracts-upgradeable/access/OwnableUpgradeable.sol";
contract MyContract is Initializable, OwnableUpgradeable {
uint256 private value;
function initialize(uint256 _value) public initializer {
__Ownable_init();
value = _value;
}
function getValue() public view returns (uint256) {
return value;
}
function setValue(uint256 _value) public onlyOwner {
value = _value;
}
}
Using OpenZeppelin’s upgradeable contracts, the Initializable
and OwnableUpgradeable
contracts help manage initialization and ownership, ensuring that the contract can be securely upgraded.
Thorough Testing
Rigorously test the upgrade process, simulating potential migration scenarios and ensuring a smooth transition from the old to the new contract. This involves testing not only the new logic but also the migration steps themselves.
Example: Testing with Truffle
Here’s how you can set up a test for an upgradeable contract using Truffle:
// migrations/2_deploy_contracts.js
const MyContract = artifacts.require("MyContract");
module.exports = async function (deployer) {
await deployer.deploy(MyContract);
const myContractInstance = await MyContract.deployed();
await myContractInstance.initialize(42);
};
// test/MyContract.test.js
const MyContract = artifacts.require("MyContract");
contract("MyContract", (accounts) => {
it("should initialize with the correct value", async () => {
const instance = await MyContract.deployed();
const value = await instance.getValue();
assert.equal(value.toNumber(), 42, "The initial value is not correct");
});
it("should update the value", async () => {
const instance = await MyContract.deployed();
await instance.setValue(100, { from: accounts[0] });
const value = await instance.getValue();
assert.equal(value.toNumber(), 100, "The value was not updated correctly");
});
});
By testing the contract thoroughly, developers can catch potential issues early in the development process, reducing the risk of upgrade bugs in production.
Decentralized Governance
Explore mechanisms for decentralized governance where the community plays a role in approving and deploying upgrades, reducing reliance on a single entity. This can be achieved through on-chain voting mechanisms and multi-signature wallets.
Example: On-Chain Governance
Here’s a simplified example of how on-chain governance might be implemented:
// Governance Token
contract GovernanceToken is ERC20 {
constructor() ERC20("GovernanceToken", "GT") {
_mint(msg.sender, 1000 * 10 ** decimals());
}
}
// Governance Contract
contract Governance {
GovernanceToken public token;
address public proposal;
uint256 public votesFor;
uint256 public votesAgainst;
mapping(address => bool) public hasVoted;
constructor(address _token) {
token = GovernanceToken(_token);
}
function proposeUpgrade(address _proposal) public {
proposal = _proposal;
votesFor = 0;
votesAgainst = 0;
}
function vote(bool support) public {
require(!hasVoted[msg.sender], "Already voted");
uint256 weight = token.balanceOf(msg.sender);
if (support) {
votesFor += weight;
} else {
votesAgainst += weight;
}
hasVoted[msg.sender] = true;
}
function executeUpgrade() public {
require(votesFor > votesAgainst, "Upgrade not approved");
// Logic to upgrade the contract
}
}
In this example, token holders can propose and vote on upgrades, ensuring that the decision is made collectively, rather than by a single entity.
Conclusion: Deal with Upgrade Bugs
Upgrading smart contracts in Web3 is a complex but essential task. The immutable nature of the blockchain poses unique challenges, but with careful planning, thorough testing, and community involvement, developers can navigate these challenges and mitigate the risks of upgrade bugs. By adopting best practices and leveraging established frameworks and governance mechanisms, the Web3 ecosystem can continue to innovate and evolve while maintaining security and stability.
FAQs
What are smart contract upgrades?
- Smart contract upgrades involve modifying or replacing a smart contract to fix bugs or add new features without deploying a completely new contract.
Why are smart contract upgrades important?
- They address bugs, enhance functionality, and improve security, ensuring the contract remains effective and secure over time.
How do developers overcome immutability challenges in smart contracts?
- Developers use design patterns like proxy contracts or upgradeable contract frameworks to allow for modifications while preserving immutability.
What is a proxy contract in smart contract development?
- A proxy contract delegates calls to an implementation contract, enabling upgrades by changing the implementation without altering the proxy’s address.
Are there risks associated with smart contract upgrades?
- Yes, risks include introducing new vulnerabilities, losing contract state, and increasing complexity, which requires thorough testing and audits.
What is Solidity and how is it related to smart contract upgrades?
- Solidity is a programming language for writing smart contracts on Ethereum. It supports design patterns that facilitate contract upgrades.
How do decentralized applications (dApps) benefit from upgradeable smart contracts?
- Upgradeable contracts allow dApps to adapt to changing requirements, fix issues, and add new features without significant downtime or disruption.
What are the best practices for upgrading smart contracts?
- Best practices include using well-tested upgradeability patterns, thorough testing, regular audits, and maintaining clear documentation.
How does blockchain’s immutability impact smart contract upgrades?
- While immutability ensures data integrity and security, it poses challenges for updates, making upgradeable design patterns essential for flexibility.
What tools and frameworks assist in smart contract upgrades?
- Tools like OpenZeppelin’s upgradeable contracts library and frameworks like Truffle and Hardhat provide support for developing and managing upgradeable smart contracts.