Solidity is a popular, high-level programming language that is used to develop smart contracts on the ethereum blockchain. These self-executing contracts hold the potential to revolutionize industries by automating complex processes and minimizing the need for intermediaries. A blockchain continues to evolve, it is important for developers who are looking forward to dive into Web3 to understand the basics of Solidity.
This article provides an introduction to the fundamental concepts of Solidity, guiding you through solidity basic syntax and helping you start your journey in blockchain development. A solid understanding of these foundational principles is crucial for success in the evolving blockchain landscape.
Basic Solidity Syntax
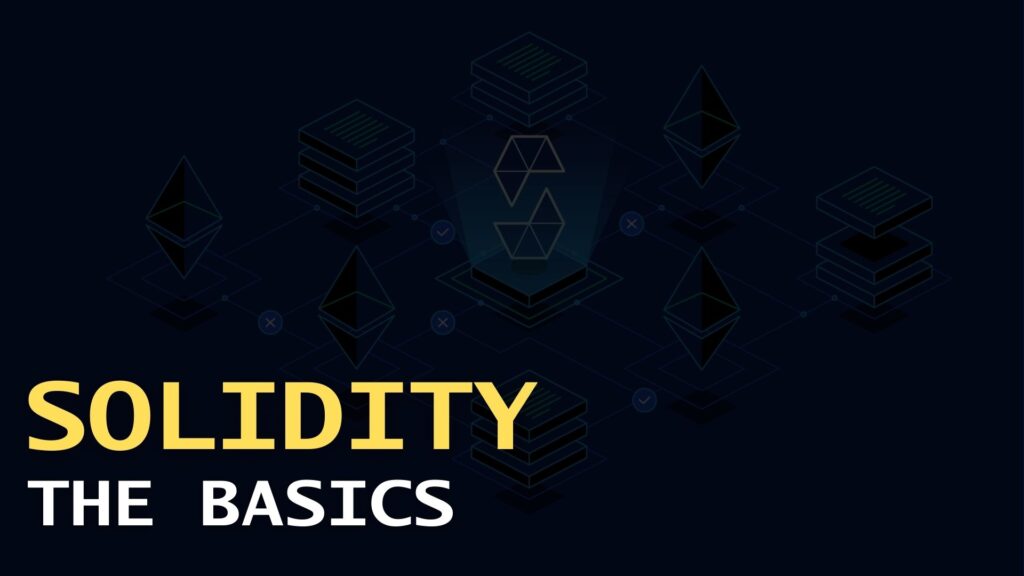
Solidity is a statically typed programming language, with a syntax influenced by JavaScript, designed to enable developers to create decentralized applications that function autonomously on the Ethereum network. For blockchain developers, mastering the fundamentals of Solidity is essential to building secure and efficient smart contracts that seamlessly operate within the blockchain environment.
1. Variables and Data Types
Similar to other programming languages, Solidity utilizes variables to store data. As a statically typed language, Solidity requires developers to specify a data type when declaring a variable, indicating the kind of value it holds. Below are some of the fundamental data types used in Solidity.
- uint: Unsigned integer – e.g.,
uint256
for 256-bit unsigned integer - int: Signed integer – e.g.,
int256
for 256-bit signed integer - address: Ethereum address – 160-bit hexadecimal value
- bool: Boolean – true or false
- string: String – e.g., “Hello world”
- bytes: Fixed-size byte array – e.g.,
bytes32
for 32 bytes - bytes32: 32-byte fixed-size byte array
You can declare a variable in Solidity using the following code.
// dataType variableName = value
uint balance = 100; // a variable with unit type
address owner = 0x1234567890abcdef; // a variable with address type
bool isPublic = true; // a variable with bool type
2. Pragma Directive in Solidity
The Pragma
directive is used to specify the version of the Solidity compiler to use for compiling the contract. This ensures compatibility with specific Solidity versions.
pragma solidity ^0.8.0;
The caret ( ^ )
allows for patch updates which allows the code to be compatible with versions 0.8.x
but not 0.9.0
or higher.
3. Functions and Visibility Specifiers
Functions in Solidity are reusable code blocks that can be executed under specific conditions. You can define a function on Solidity using the following code.
function functionName(address to, unit amount) public {
// function body
}
In the code above, the function is declared using the function
keyword. The functionName
is a placeholder for the actual name of the function and can be customized accordingly. address to
and uint amount
are the function parameters that are passed whenever the function is invoked. The public
keyword specifies the visibility of the function. You can replace the // function body
with the appropriate implementation to achieve the desired functionality.
Following are some visibility specifiers available in Solidity
- public: Accessible from anywhere.
- private: Accessible only within the contract.
- internal: Accessible within the contract and its derived contracts.
- external: Accessible only from outside the contract.
4. Modifiers
Modifiers are reusable code blocks that can be applied to functions to add additional functionality. You can declare a modifier using the following code.
modifier onlyOwner() {
require(msg.sender == owner);
_;
}
//usage of a modifier in a function
function functionName(address to, unit amount) public onlyOwner {
// function body
}
In the above code, The onlyOwner
modifier checks if the caller of the function msg.sender
is the contract’s owner by using the require
statement. When this modifier is applied to a function, this restricts access to the function allowing it to be executed only when the condition is met.
5. Contract Declaration
In Solidity, we create smart contracts using the contract
keyword following by the contract name. A contract in Solidity can be thought of as a class in object-oriented programming. It can hold state variables, functions, modifiers, and events. You can use the following code to declare a contract on Solidity.
contract MyContract {
// contract body
}
6. Events
Events in Solidity allows smart contracts to communicate with external applications. They are used to emit notifications when specific actions occur within a smart contract. You can refer to the following code to create an event in Solidity.
event CountIncremented(uint256 newCount);
function increment() public {
count += 1;
emit CountIncremented(count);
}
7. Structs
Structs in Solidity are user-defined data types that allow developers to group related variables together. This concept is comparable to classes in Object-Oriented Programming (OOP), as it enables the creation of structured and organized data. You can refer to the following code to create a struct.
struct Person {
string name;
unit age;
}
// usage
Person public person = Person("Alice", 30);
Importance of Learning Solidity in Web3
Solidity serves as the backbone for developing decentralized applications and smart contracts on the blockchain. If you are a developer looking forward to dive into web3, having a deep understanding on Solidity is very important. Its syntax, inspired by JavaScript, makes it accessible to developers familiar with modern programming languages, however, it introduces concepts that are unique to blockchain, like gas optimization and security, which are essential for ensuring efficient and safe smart contracts.
Since smart contracts are immutable and cannot be changed after being deployed to the blockchain, it is important for a developer to have a deep knowledge on Solidity before getting started with developing smart contracts. Additionally, learning Solidity opens up opportunities for developers to not only build cutting-edge applications but also grasp the underlying mechanics of decentralized technologies.
This knowledge is valuable for anyone looking to participate in or contribute to the future of decentralized, trustless systems, making Solidity an indispensable skill for the Web3 era.
Conclusion
For developers already familiar with programming languages like JavaScript or Python, learning Solidity can be relatively straightforward. The syntax of Solidity is quite similar to these languages, which simplifies the learning process for experienced developers. However, for those new to coding, it is highly recommended to first learn basic programming principles before diving into Solidity.
On the other hand, getting started with Solidity is highly beneficial, even for developers in the early stages of their careers. It positions them well in the rapidly evolving blockchain field. Nevertheless, it’s crucial to understand that mastering the basic syntax of Solidity is just the first step toward writing smart contracts. As you delve deeper into Solidity, you’ll encounter more advanced topics that will become increasingly engaging and insightful as you progress in your learning journey.
FAQs:
What is Solidity?
- Solidity is a programming language used for writing smart contracts that run on Ethereum and other blockchain platforms.
How does Solidity syntax differ from other languages?
- Solidity has a syntax similar to JavaScript and C++, with specific structures for handling blockchain interactions and contract states.
What are the basic data types in Solidity?
- Solidity includes data types like integers, booleans, strings, addresses, arrays, and mappings, which are essential for smart contract development.
How do you define a function in Solidity?
- Functions in Solidity are defined using the
function
keyword, followed by the function name, parameters, and a code block enclosed in curly braces.
Is Solidity easy for beginners to learn?
- Solidity can be challenging, especially for beginners in programming, but with a solid grasp of its syntax, you can build smart contracts with practice and study.